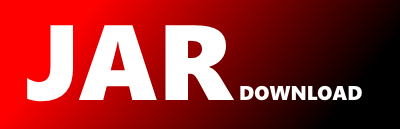
com.lob.api.client.IdentityValidationApi Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.api.client;
import com.lob.api.ApiCallback;
import com.lob.api.ApiClient;
import com.lob.api.ApiException;
import com.lob.api.ApiResponse;
import com.lob.api.Configuration;
import com.lob.api.Pair;
import com.lob.api.ProgressRequestBody;
import com.lob.api.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import com.lob.model.IdentityValidation;
import com.lob.model.LobError;
import com.lob.model.MultiLineAddress;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class IdentityValidationApi {
private ApiClient localVarApiClient;
public IdentityValidationApi() {
this(Configuration.getDefaultApiClient());
}
public IdentityValidationApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
/**
* Build call for validate
* @param multiLineAddress (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @http.response.details
Status Code Description Response Headers
200 Returns the likelihood a given name is associated with an address. * ratelimit-limit -
* ratelimit-remaining -
* ratelimit-reset -
0 Lob uses RESTful HTTP response codes to indicate success or failure of an API request. -
*/
public okhttp3.Call validateCall(MultiLineAddress multiLineAddress, final ApiCallback _callback) throws ApiException {
Object localVarPostBody = multiLineAddress;
// create path and map variables
String localVarPath = "/identity_validation";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json", "application/x-www-form-urlencoded", "multipart/form-data"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
String[] localVarAuthNames = new String[] { "basicAuth" };
return localVarApiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call validateValidateBeforeCall(MultiLineAddress multiLineAddress, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'multiLineAddress' is set
if (multiLineAddress == null) {
throw new ApiException("Missing the required parameter 'multiLineAddress' when calling validate(Async)");
}
okhttp3.Call localVarCall = validateCall(multiLineAddress, _callback);
return localVarCall;
}
/**
* validate
* Validates whether a given name is associated with an address.
* @param multiLineAddress (required)
* @return IdentityValidation
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
* @http.response.details
Status Code Description Response Headers
200 Returns the likelihood a given name is associated with an address. * ratelimit-limit -
* ratelimit-remaining -
* ratelimit-reset -
0 Lob uses RESTful HTTP response codes to indicate success or failure of an API request. -
*/
public IdentityValidation validate(MultiLineAddress multiLineAddress) throws ApiException {
try {
ApiResponse localVarResp = validateWithHttpInfo(multiLineAddress);
return localVarResp.getData();
} catch (ApiException e) {
throw e;
}
}
/**
* validate
* Validates whether a given name is associated with an address.
* @param multiLineAddress (required)
* @return ApiResponse<IdentityValidation>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
* @http.response.details
Status Code Description Response Headers
200 Returns the likelihood a given name is associated with an address. * ratelimit-limit -
* ratelimit-remaining -
* ratelimit-reset -
0 Lob uses RESTful HTTP response codes to indicate success or failure of an API request. -
*/
public ApiResponse validateWithHttpInfo(MultiLineAddress multiLineAddress) throws ApiException {
try {
okhttp3.Call localVarCall = validateValidateBeforeCall(multiLineAddress, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
throw e;
}
}
/**
* validate (asynchronously)
* Validates whether a given name is associated with an address.
* @param multiLineAddress (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @http.response.details
Status Code Description Response Headers
200 Returns the likelihood a given name is associated with an address. * ratelimit-limit -
* ratelimit-remaining -
* ratelimit-reset -
0 Lob uses RESTful HTTP response codes to indicate success or failure of an API request. -
*/
public okhttp3.Call validateAsync(MultiLineAddress multiLineAddress, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = validateValidateBeforeCall(multiLineAddress, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
}