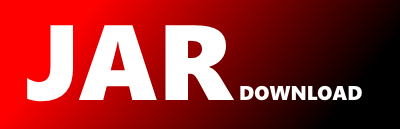
com.lob.model.Address Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.lob.model.CountryExtendedExpanded;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.jackson.nullable.JsonNullable;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* Address
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class Address {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
/**
* Unique identifier prefixed with `adr_`.
* @return id
**/
@javax.annotation.Nullable
public String getId() { return id; }
public void setId (String id) throws IllegalArgumentException {
if(!id.matches("^adr_[a-zA-Z0-9]+$")) {
throw new IllegalArgumentException("Invalid id provided");
}
this.id = id;
}
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
/**
* An internal description that identifies this resource. Must be no longer than 255 characters.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An internal description that identifies this resource. Must be no longer than 255 characters. ")
public String getDescription() {
return description;
}
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
/**
* name associated with address
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "name associated with address")
public String getName() {
return name;
}
public static final String SERIALIZED_NAME_COMPANY = "company";
@SerializedName(SERIALIZED_NAME_COMPANY)
private String company;
/**
* Either `name` or `company` is required, you may also add both.
* @return company
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Either `name` or `company` is required, you may also add both.")
public String getCompany() {
return company;
}
public static final String SERIALIZED_NAME_PHONE = "phone";
@SerializedName(SERIALIZED_NAME_PHONE)
private String phone;
/**
* Must be no longer than 40 characters.
* @return phone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Must be no longer than 40 characters.")
public String getPhone() {
return phone;
}
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
/**
* Must be no longer than 100 characters.
* @return email
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Must be no longer than 100 characters.")
public String getEmail() {
return email;
}
public static final String SERIALIZED_NAME_METADATA = "metadata";
@SerializedName(SERIALIZED_NAME_METADATA)
private Map metadata = null;
public Map getMetadata() {
if (this.metadata == null) {
this.metadata = new HashMap();
}
return this.metadata;
}
public static final String SERIALIZED_NAME_ADDRESS_LINE1 = "address_line1";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE1)
private String addressLine1;
/**
* Get addressLine1
* @return addressLine1
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAddressLine1() {
return addressLine1;
}
public static final String SERIALIZED_NAME_ADDRESS_LINE2 = "address_line2";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE2)
private String addressLine2;
/**
* Get addressLine2
* @return addressLine2
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAddressLine2() {
return addressLine2;
}
public static final String SERIALIZED_NAME_ADDRESS_CITY = "address_city";
@SerializedName(SERIALIZED_NAME_ADDRESS_CITY)
private String addressCity;
/**
* Get addressCity
* @return addressCity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAddressCity() {
return addressCity;
}
public static final String SERIALIZED_NAME_ADDRESS_STATE = "address_state";
@SerializedName(SERIALIZED_NAME_ADDRESS_STATE)
private String addressState;
/**
* 2 letter state short-name code
* @return addressState
**/
@javax.annotation.Nullable
public String getAddressState() { return addressState; }
public void setAddressState (String addressState) throws IllegalArgumentException {
if(!addressState.matches("^[a-zA-Z]{2}$")) {
throw new IllegalArgumentException("Invalid address_state provided");
}
this.addressState = addressState;
}
public static final String SERIALIZED_NAME_ADDRESS_ZIP = "address_zip";
@SerializedName(SERIALIZED_NAME_ADDRESS_ZIP)
private String addressZip;
/**
* Must follow the ZIP format of `12345` or ZIP+4 format of `12345-1234`.
* @return addressZip
**/
@javax.annotation.Nullable
public String getAddressZip() { return addressZip; }
public void setAddressZip (String addressZip) throws IllegalArgumentException {
if(!addressZip.matches("^\\d{5}(-\\d{4})?$")) {
throw new IllegalArgumentException("Invalid address_zip provided");
}
this.addressZip = addressZip;
}
public static final String SERIALIZED_NAME_ADDRESS_COUNTRY = "address_country";
@SerializedName(SERIALIZED_NAME_ADDRESS_COUNTRY)
private CountryExtendedExpanded addressCountry;
/**
* Get addressCountry
* @return addressCountry
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CountryExtendedExpanded getAddressCountry() {
return addressCountry;
}
/**
* Gets or Sets _object
*/
@JsonAdapter(ObjectEnum.Adapter.class)
public enum ObjectEnum {
ADDRESS("address");
private String value;
ObjectEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ObjectEnum fromValue(String value) {
for (ObjectEnum b : ObjectEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ObjectEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ObjectEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ObjectEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_OBJECT = "object";
@SerializedName(SERIALIZED_NAME_OBJECT)
private ObjectEnum _object = ObjectEnum.ADDRESS;
/**
* Get _object
* @return _object
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ObjectEnum getObject() {
return _object;
}
public static final String SERIALIZED_NAME_DATE_CREATED = "date_created";
@SerializedName(SERIALIZED_NAME_DATE_CREATED)
private OffsetDateTime dateCreated;
/**
* A timestamp in ISO 8601 format of the date the resource was created.
* @return dateCreated
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A timestamp in ISO 8601 format of the date the resource was created.")
public OffsetDateTime getDateCreated() {
return dateCreated;
}
public static final String SERIALIZED_NAME_DATE_MODIFIED = "date_modified";
@SerializedName(SERIALIZED_NAME_DATE_MODIFIED)
private OffsetDateTime dateModified;
/**
* A timestamp in ISO 8601 format of the date the resource was last modified.
* @return dateModified
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A timestamp in ISO 8601 format of the date the resource was last modified.")
public OffsetDateTime getDateModified() {
return dateModified;
}
public static final String SERIALIZED_NAME_DELETED = "deleted";
@SerializedName(SERIALIZED_NAME_DELETED)
private Boolean deleted;
/**
* Only returned if the resource has been successfully deleted.
* @return deleted
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Only returned if the resource has been successfully deleted.")
public Boolean getDeleted() {
return deleted;
}
public static final String SERIALIZED_NAME_RECIPIENT_MOVED = "recipient_moved";
@SerializedName(SERIALIZED_NAME_RECIPIENT_MOVED)
private Boolean recipientMoved;
/**
* Only returned for accounts on certain Print & Mail Editions. Value is `true` if the address was altered because the recipient filed for a National Change of Address (NCOA), `false` if the NCOA check was run but no altered address was found, and `null` if the NCOA check was not run. The NCOA check does not happen for non-US addresses, for non-deliverable US addresses, or for addresses created before the NCOA feature was added to your account.
* @return recipientMoved
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Only returned for accounts on certain Print & Mail Editions. Value is `true` if the address was altered because the recipient filed for a National Change of Address (NCOA), `false` if the NCOA check was run but no altered address was found, and `null` if the NCOA check was not run. The NCOA check does not happen for non-US addresses, for non-deliverable US addresses, or for addresses created before the NCOA feature was added to your account. ")
public Boolean getRecipientMoved() {
return recipientMoved;
}
/*
public Address id(String id) {
this.id = id;
return this;
}
*/
/*
public Address description(String description) {
this.description = description;
return this;
}
*/
public void setDescription(String description) {
this.description = description;
}
/*
public Address name(String name) {
this.name = name;
return this;
}
*/
public void setName(String name) {
this.name = name;
}
/*
public Address company(String company) {
this.company = company;
return this;
}
*/
public void setCompany(String company) {
this.company = company;
}
/*
public Address phone(String phone) {
this.phone = phone;
return this;
}
*/
public void setPhone(String phone) {
this.phone = phone;
}
/*
public Address email(String email) {
this.email = email;
return this;
}
*/
public void setEmail(String email) {
this.email = email;
}
/*
public Address metadata(Map metadata) {
this.metadata = metadata;
return this;
}
*/
public Address putMetadataItem(String key, String metadataItem) {
if (this.metadata == null) {
this.metadata = new HashMap();
}
this.metadata.put(key, metadataItem);
return this;
}
public void setMetadata(Map metadata) {
this.metadata = metadata;
}
/*
public Address addressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
return this;
}
*/
public void setAddressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
}
/*
public Address addressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
return this;
}
*/
public void setAddressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
}
/*
public Address addressCity(String addressCity) {
this.addressCity = addressCity;
return this;
}
*/
public void setAddressCity(String addressCity) {
this.addressCity = addressCity;
}
/*
public Address addressState(String addressState) {
this.addressState = addressState;
return this;
}
*/
/*
public Address addressZip(String addressZip) {
this.addressZip = addressZip;
return this;
}
*/
/*
public Address addressCountry(CountryExtendedExpanded addressCountry) {
this.addressCountry = addressCountry;
return this;
}
*/
public void setAddressCountry(CountryExtendedExpanded addressCountry) {
this.addressCountry = addressCountry;
}
/*
public Address _object(ObjectEnum _object) {
this._object = _object;
return this;
}
*/
public void setObject(ObjectEnum _object) {
this._object = _object;
}
/*
public Address dateCreated(OffsetDateTime dateCreated) {
this.dateCreated = dateCreated;
return this;
}
*/
public void setDateCreated(OffsetDateTime dateCreated) {
this.dateCreated = dateCreated;
}
/*
public Address dateModified(OffsetDateTime dateModified) {
this.dateModified = dateModified;
return this;
}
*/
public void setDateModified(OffsetDateTime dateModified) {
this.dateModified = dateModified;
}
/*
public Address deleted(Boolean deleted) {
this.deleted = deleted;
return this;
}
*/
public void setDeleted(Boolean deleted) {
this.deleted = deleted;
}
/*
public Address recipientMoved(Boolean recipientMoved) {
this.recipientMoved = recipientMoved;
return this;
}
*/
public void setRecipientMoved(Boolean recipientMoved) {
this.recipientMoved = recipientMoved;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Address address = (Address) o;
return Objects.equals(this.id, address.id) &&
Objects.equals(this.description, address.description) &&
Objects.equals(this.name, address.name) &&
Objects.equals(this.company, address.company) &&
Objects.equals(this.phone, address.phone) &&
Objects.equals(this.email, address.email) &&
Objects.equals(this.metadata, address.metadata) &&
Objects.equals(this.addressLine1, address.addressLine1) &&
Objects.equals(this.addressLine2, address.addressLine2) &&
Objects.equals(this.addressCity, address.addressCity) &&
Objects.equals(this.addressState, address.addressState) &&
Objects.equals(this.addressZip, address.addressZip) &&
Objects.equals(this.addressCountry, address.addressCountry) &&
Objects.equals(this._object, address._object) &&
Objects.equals(this.dateCreated, address.dateCreated) &&
Objects.equals(this.dateModified, address.dateModified) &&
Objects.equals(this.deleted, address.deleted) &&
Objects.equals(this.recipientMoved, address.recipientMoved);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, description, name, company, phone, email, metadata, addressLine1, addressLine2, addressCity, addressState, addressZip, addressCountry, _object, dateCreated, dateModified, deleted, recipientMoved);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" company: ").append(toIndentedString(company)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" addressLine1: ").append(toIndentedString(addressLine1)).append("\n");
sb.append(" addressLine2: ").append(toIndentedString(addressLine2)).append("\n");
sb.append(" addressCity: ").append(toIndentedString(addressCity)).append("\n");
sb.append(" addressState: ").append(toIndentedString(addressState)).append("\n");
sb.append(" addressZip: ").append(toIndentedString(addressZip)).append("\n");
sb.append(" addressCountry: ").append(toIndentedString(addressCountry)).append("\n");
sb.append(" _object: ").append(toIndentedString(_object)).append("\n");
sb.append(" dateCreated: ").append(toIndentedString(dateCreated)).append("\n");
sb.append(" dateModified: ").append(toIndentedString(dateModified)).append("\n");
sb.append(" deleted: ").append(toIndentedString(deleted)).append("\n");
sb.append(" recipientMoved: ").append(toIndentedString(recipientMoved)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("id", id);
localMap.put("description", description);
localMap.put("name", name);
localMap.put("company", company);
localMap.put("phone", phone);
localMap.put("email", email);
localMap.put("metadata", metadata);
localMap.put("address_line1", addressLine1);
localMap.put("address_line2", addressLine2);
localMap.put("address_city", addressCity);
localMap.put("address_state", addressState);
localMap.put("address_zip", addressZip);
localMap.put("address_country", addressCountry);
localMap.put("object", _object);
localMap.put("date_created", dateCreated);
localMap.put("date_modified", dateModified);
localMap.put("deleted", deleted);
localMap.put("recipient_moved", recipientMoved);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}