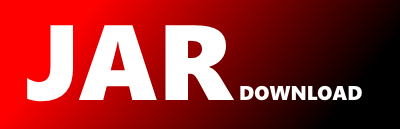
com.lob.model.AddressEditable Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.lob.model.CountryExtended;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.jackson.nullable.JsonNullable;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* AddressEditable
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class AddressEditable {
public static final String SERIALIZED_NAME_ADDRESS_LINE1 = "address_line1";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE1)
private String addressLine1;
/**
* The building number, street name, street suffix, and any street directionals. For US addresses, the max length is 64 characters.
* @return addressLine1
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The building number, street name, street suffix, and any street directionals. For US addresses, the max length is 64 characters.")
public String getAddressLine1() {
return addressLine1;
}
public static final String SERIALIZED_NAME_ADDRESS_LINE2 = "address_line2";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE2)
private String addressLine2;
/**
* The suite or apartment number of the recipient address, if applicable. For US addresses, the max length is 64 characters.
* @return addressLine2
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The suite or apartment number of the recipient address, if applicable. For US addresses, the max length is 64 characters.")
public String getAddressLine2() {
return addressLine2;
}
public static final String SERIALIZED_NAME_ADDRESS_CITY = "address_city";
@SerializedName(SERIALIZED_NAME_ADDRESS_CITY)
private String addressCity;
/**
* Get addressCity
* @return addressCity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAddressCity() {
return addressCity;
}
public static final String SERIALIZED_NAME_ADDRESS_STATE = "address_state";
@SerializedName(SERIALIZED_NAME_ADDRESS_STATE)
private String addressState;
/**
* Get addressState
* @return addressState
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAddressState() {
return addressState;
}
public static final String SERIALIZED_NAME_ADDRESS_ZIP = "address_zip";
@SerializedName(SERIALIZED_NAME_ADDRESS_ZIP)
private String addressZip;
/**
* Optional postal code. For US addresses, must be either 5 or 9 digits.
* @return addressZip
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Optional postal code. For US addresses, must be either 5 or 9 digits.")
public String getAddressZip() {
return addressZip;
}
public static final String SERIALIZED_NAME_ADDRESS_COUNTRY = "address_country";
@SerializedName(SERIALIZED_NAME_ADDRESS_COUNTRY)
private CountryExtended addressCountry;
/**
* Get addressCountry
* @return addressCountry
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CountryExtended getAddressCountry() {
return addressCountry;
}
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
/**
* An internal description that identifies this resource. Must be no longer than 255 characters.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An internal description that identifies this resource. Must be no longer than 255 characters. ")
public String getDescription() {
return description;
}
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
/**
* name associated with address.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "name associated with address.")
public String getName() {
return name;
}
public static final String SERIALIZED_NAME_COMPANY = "company";
@SerializedName(SERIALIZED_NAME_COMPANY)
private String company;
/**
* Either `name` or `company` is required, you may also add both.
* @return company
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Either `name` or `company` is required, you may also add both.")
public String getCompany() {
return company;
}
public static final String SERIALIZED_NAME_PHONE = "phone";
@SerializedName(SERIALIZED_NAME_PHONE)
private String phone;
/**
* Must be no longer than 40 characters.
* @return phone
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Must be no longer than 40 characters.")
public String getPhone() {
return phone;
}
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
/**
* Must be no longer than 100 characters.
* @return email
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Must be no longer than 100 characters.")
public String getEmail() {
return email;
}
public static final String SERIALIZED_NAME_METADATA = "metadata";
@SerializedName(SERIALIZED_NAME_METADATA)
private Map metadata = null;
public Map getMetadata() {
if (this.metadata == null) {
this.metadata = new HashMap();
}
return this.metadata;
}
/*
public AddressEditable addressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
return this;
}
*/
public void setAddressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
}
/*
public AddressEditable addressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
return this;
}
*/
public void setAddressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
}
/*
public AddressEditable addressCity(String addressCity) {
this.addressCity = addressCity;
return this;
}
*/
public void setAddressCity(String addressCity) {
this.addressCity = addressCity;
}
/*
public AddressEditable addressState(String addressState) {
this.addressState = addressState;
return this;
}
*/
public void setAddressState(String addressState) {
this.addressState = addressState;
}
/*
public AddressEditable addressZip(String addressZip) {
this.addressZip = addressZip;
return this;
}
*/
public void setAddressZip(String addressZip) {
this.addressZip = addressZip;
}
/*
public AddressEditable addressCountry(CountryExtended addressCountry) {
this.addressCountry = addressCountry;
return this;
}
*/
public void setAddressCountry(CountryExtended addressCountry) {
this.addressCountry = addressCountry;
}
/*
public AddressEditable description(String description) {
this.description = description;
return this;
}
*/
public void setDescription(String description) {
this.description = description;
}
/*
public AddressEditable name(String name) {
this.name = name;
return this;
}
*/
public void setName(String name) {
this.name = name;
}
/*
public AddressEditable company(String company) {
this.company = company;
return this;
}
*/
public void setCompany(String company) {
this.company = company;
}
/*
public AddressEditable phone(String phone) {
this.phone = phone;
return this;
}
*/
public void setPhone(String phone) {
this.phone = phone;
}
/*
public AddressEditable email(String email) {
this.email = email;
return this;
}
*/
public void setEmail(String email) {
this.email = email;
}
/*
public AddressEditable metadata(Map metadata) {
this.metadata = metadata;
return this;
}
*/
public AddressEditable putMetadataItem(String key, String metadataItem) {
if (this.metadata == null) {
this.metadata = new HashMap();
}
this.metadata.put(key, metadataItem);
return this;
}
public void setMetadata(Map metadata) {
this.metadata = metadata;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AddressEditable addressEditable = (AddressEditable) o;
return Objects.equals(this.addressLine1, addressEditable.addressLine1) &&
Objects.equals(this.addressLine2, addressEditable.addressLine2) &&
Objects.equals(this.addressCity, addressEditable.addressCity) &&
Objects.equals(this.addressState, addressEditable.addressState) &&
Objects.equals(this.addressZip, addressEditable.addressZip) &&
Objects.equals(this.addressCountry, addressEditable.addressCountry) &&
Objects.equals(this.description, addressEditable.description) &&
Objects.equals(this.name, addressEditable.name) &&
Objects.equals(this.company, addressEditable.company) &&
Objects.equals(this.phone, addressEditable.phone) &&
Objects.equals(this.email, addressEditable.email) &&
Objects.equals(this.metadata, addressEditable.metadata);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(addressLine1, addressLine2, addressCity, addressState, addressZip, addressCountry, description, name, company, phone, email, metadata);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" addressLine1: ").append(toIndentedString(addressLine1)).append("\n");
sb.append(" addressLine2: ").append(toIndentedString(addressLine2)).append("\n");
sb.append(" addressCity: ").append(toIndentedString(addressCity)).append("\n");
sb.append(" addressState: ").append(toIndentedString(addressState)).append("\n");
sb.append(" addressZip: ").append(toIndentedString(addressZip)).append("\n");
sb.append(" addressCountry: ").append(toIndentedString(addressCountry)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" company: ").append(toIndentedString(company)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("address_line1", addressLine1);
localMap.put("address_line2", addressLine2);
localMap.put("address_city", addressCity);
localMap.put("address_state", addressState);
localMap.put("address_zip", addressZip);
localMap.put("address_country", addressCountry);
localMap.put("description", description);
localMap.put("name", name);
localMap.put("company", company);
localMap.put("phone", phone);
localMap.put("email", email);
localMap.put("metadata", metadata);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}