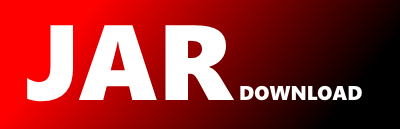
com.lob.model.BankAccountDeletion Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* Lob uses RESTful HTTP response codes to indicate success or failure of an API request. In general, 2xx indicates success, 4xx indicate an input error, and 5xx indicates an error on Lob's end.
*/
@ApiModel(description = "Lob uses RESTful HTTP response codes to indicate success or failure of an API request. In general, 2xx indicates success, 4xx indicate an input error, and 5xx indicates an error on Lob's end.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class BankAccountDeletion {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
/**
* Unique identifier prefixed with `bank_`.
* @return id
**/
@javax.annotation.Nullable
public String getId() { return id; }
public void setId (String id) throws IllegalArgumentException {
if(!id.matches("^bank_[a-zA-Z0-9]+$")) {
throw new IllegalArgumentException("Invalid id provided");
}
this.id = id;
}
public static final String SERIALIZED_NAME_DELETED = "deleted";
@SerializedName(SERIALIZED_NAME_DELETED)
private Boolean deleted;
/**
* Only returned if the resource has been successfully deleted.
* @return deleted
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Only returned if the resource has been successfully deleted.")
public Boolean getDeleted() {
return deleted;
}
/**
* Value is type of resource.
*/
@JsonAdapter(ObjectEnum.Adapter.class)
public enum ObjectEnum {
BANK_ACCOUNT_DELETED("bank_account_deleted");
private String value;
ObjectEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ObjectEnum fromValue(String value) {
for (ObjectEnum b : ObjectEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ObjectEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ObjectEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ObjectEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_OBJECT = "object";
@SerializedName(SERIALIZED_NAME_OBJECT)
private ObjectEnum _object = ObjectEnum.BANK_ACCOUNT_DELETED;
/**
* Value is type of resource.
* @return _object
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Value is type of resource.")
public ObjectEnum getObject() {
return _object;
}
/*
public BankAccountDeletion id(String id) {
this.id = id;
return this;
}
*/
/*
public BankAccountDeletion deleted(Boolean deleted) {
this.deleted = deleted;
return this;
}
*/
public void setDeleted(Boolean deleted) {
this.deleted = deleted;
}
/*
public BankAccountDeletion _object(ObjectEnum _object) {
this._object = _object;
return this;
}
*/
public void setObject(ObjectEnum _object) {
this._object = _object;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BankAccountDeletion bankAccountDeletion = (BankAccountDeletion) o;
return Objects.equals(this.id, bankAccountDeletion.id) &&
Objects.equals(this.deleted, bankAccountDeletion.deleted) &&
Objects.equals(this._object, bankAccountDeletion._object);
}
@Override
public int hashCode() {
return Objects.hash(id, deleted, _object);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" deleted: ").append(toIndentedString(deleted)).append("\n");
sb.append(" _object: ").append(toIndentedString(_object)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("id", id);
localMap.put("deleted", deleted);
localMap.put("object", _object);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}