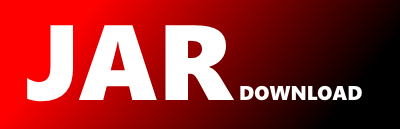
com.lob.model.DeliverabilityAnalysis Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.lob.model.DpvFootnote;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* A nested object containing a breakdown of the deliverability of an address.
*/
@ApiModel(description = "A nested object containing a breakdown of the deliverability of an address.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class DeliverabilityAnalysis {
/**
* Result of Delivery Point Validation (DPV), which determines whether or not the address is deliverable by the USPS. Possible values are: * `Y` –– The address is deliverable by the USPS. * `S` –– The address is deliverable by removing the provided secondary unit designator. This information may be incorrect or unnecessary. * `D` –– The address is deliverable to the building's default address but is missing a secondary unit designator and/or number. There is a chance the mail will not reach the intended recipient. * `N` –– The address is not deliverable according to the USPS, but parts of the address are valid (such as the street and ZIP code). * `''` –– This address is not deliverable. No matching street could be found within the city or ZIP code.
*/
@JsonAdapter(DpvConfirmationEnum.Adapter.class)
public enum DpvConfirmationEnum {
Y("Y"),
S("S"),
D("D"),
N("N"),
EMPTY("");
private String value;
DpvConfirmationEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvConfirmationEnum fromValue(String value) {
for (DpvConfirmationEnum b : DpvConfirmationEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvConfirmationEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvConfirmationEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvConfirmationEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_CONFIRMATION = "dpv_confirmation";
@SerializedName(SERIALIZED_NAME_DPV_CONFIRMATION)
private DpvConfirmationEnum dpvConfirmation;
/**
* Result of Delivery Point Validation (DPV), which determines whether or not the address is deliverable by the USPS. Possible values are: * `Y` –– The address is deliverable by the USPS. * `S` –– The address is deliverable by removing the provided secondary unit designator. This information may be incorrect or unnecessary. * `D` –– The address is deliverable to the building's default address but is missing a secondary unit designator and/or number. There is a chance the mail will not reach the intended recipient. * `N` –– The address is not deliverable according to the USPS, but parts of the address are valid (such as the street and ZIP code). * `''` –– This address is not deliverable. No matching street could be found within the city or ZIP code.
* @return dpvConfirmation
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Result of Delivery Point Validation (DPV), which determines whether or not the address is deliverable by the USPS. Possible values are: * `Y` –– The address is deliverable by the USPS. * `S` –– The address is deliverable by removing the provided secondary unit designator. This information may be incorrect or unnecessary. * `D` –– The address is deliverable to the building's default address but is missing a secondary unit designator and/or number. There is a chance the mail will not reach the intended recipient. * `N` –– The address is not deliverable according to the USPS, but parts of the address are valid (such as the street and ZIP code). * `''` –– This address is not deliverable. No matching street could be found within the city or ZIP code. ")
public DpvConfirmationEnum getDpvConfirmation() {
return dpvConfirmation;
}
/**
* indicates whether or not the address is [CMRA-authorized](https://en.wikipedia.org/wiki/Commercial_mail_receiving_agency). Possible values are: * `Y` –– Address is CMRA-authorized. * `N` –– Address is not CMRA-authorized. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
*/
@JsonAdapter(DpvCmraEnum.Adapter.class)
public enum DpvCmraEnum {
Y("Y"),
N("N"),
EMPTY("");
private String value;
DpvCmraEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvCmraEnum fromValue(String value) {
for (DpvCmraEnum b : DpvCmraEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvCmraEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvCmraEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvCmraEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_CMRA = "dpv_cmra";
@SerializedName(SERIALIZED_NAME_DPV_CMRA)
private DpvCmraEnum dpvCmra;
/**
* indicates whether or not the address is [CMRA-authorized](https://en.wikipedia.org/wiki/Commercial_mail_receiving_agency). Possible values are: * `Y` –– Address is CMRA-authorized. * `N` –– Address is not CMRA-authorized. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
* @return dpvCmra
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "indicates whether or not the address is [CMRA-authorized](https://en.wikipedia.org/wiki/Commercial_mail_receiving_agency). Possible values are: * `Y` –– Address is CMRA-authorized. * `N` –– Address is not CMRA-authorized. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). ")
public DpvCmraEnum getDpvCmra() {
return dpvCmra;
}
/**
* indicates that an address was once deliverable, but has become vacant and is no longer receiving deliveries. Possible values are: * `Y` –– Address is vacant. * `N` –– Address is not vacant. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
*/
@JsonAdapter(DpvVacantEnum.Adapter.class)
public enum DpvVacantEnum {
Y("Y"),
N("N"),
EMPTY("");
private String value;
DpvVacantEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvVacantEnum fromValue(String value) {
for (DpvVacantEnum b : DpvVacantEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvVacantEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvVacantEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvVacantEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_VACANT = "dpv_vacant";
@SerializedName(SERIALIZED_NAME_DPV_VACANT)
private DpvVacantEnum dpvVacant;
/**
* indicates that an address was once deliverable, but has become vacant and is no longer receiving deliveries. Possible values are: * `Y` –– Address is vacant. * `N` –– Address is not vacant. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
* @return dpvVacant
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "indicates that an address was once deliverable, but has become vacant and is no longer receiving deliveries. Possible values are: * `Y` –– Address is vacant. * `N` –– Address is not vacant. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). ")
public DpvVacantEnum getDpvVacant() {
return dpvVacant;
}
/**
* Corresponds to the USPS field `dpv_no_stat`. Indicates that an address has been vacated in the recent past, and is no longer receiving deliveries. If it's been unoccupied for 90+ days, or temporarily vacant, this will be flagged. Possible values are: * `Y` –– Address is active. * `N` –– Address is not active. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
*/
@JsonAdapter(DpvActiveEnum.Adapter.class)
public enum DpvActiveEnum {
Y("Y"),
N("N"),
EMPTY("");
private String value;
DpvActiveEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvActiveEnum fromValue(String value) {
for (DpvActiveEnum b : DpvActiveEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvActiveEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvActiveEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvActiveEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_ACTIVE = "dpv_active";
@SerializedName(SERIALIZED_NAME_DPV_ACTIVE)
private DpvActiveEnum dpvActive;
/**
* Corresponds to the USPS field `dpv_no_stat`. Indicates that an address has been vacated in the recent past, and is no longer receiving deliveries. If it's been unoccupied for 90+ days, or temporarily vacant, this will be flagged. Possible values are: * `Y` –– Address is active. * `N` –– Address is not active. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
* @return dpvActive
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Corresponds to the USPS field `dpv_no_stat`. Indicates that an address has been vacated in the recent past, and is no longer receiving deliveries. If it's been unoccupied for 90+ days, or temporarily vacant, this will be flagged. Possible values are: * `Y` –– Address is active. * `N` –– Address is not active. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). ")
public DpvActiveEnum getDpvActive() {
return dpvActive;
}
/**
* Indicates the reason why an address is vacant or no longer receiving deliveries. Possible values are: * `01` –– Address does not receive mail from the USPS directly, but is serviced by a drop address. * `02` –– Address not yet deliverable. * `03` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). * `04` –– Address is a College, Military Zone, or other type. * `05` –– Address no longer receives deliveries. * `06` –– Address is missing required secondary information. * `''` –– A DPV match is not made or the address is active.
*/
@JsonAdapter(DpvInactiveReasonEnum.Adapter.class)
public enum DpvInactiveReasonEnum {
_01("01"),
_02("02"),
_03("03"),
_04("04"),
_05("05"),
_06("06"),
EMPTY("");
private String value;
DpvInactiveReasonEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvInactiveReasonEnum fromValue(String value) {
for (DpvInactiveReasonEnum b : DpvInactiveReasonEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvInactiveReasonEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvInactiveReasonEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvInactiveReasonEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_INACTIVE_REASON = "dpv_inactive_reason";
@SerializedName(SERIALIZED_NAME_DPV_INACTIVE_REASON)
private DpvInactiveReasonEnum dpvInactiveReason;
/**
* Indicates the reason why an address is vacant or no longer receiving deliveries. Possible values are: * `01` –– Address does not receive mail from the USPS directly, but is serviced by a drop address. * `02` –– Address not yet deliverable. * `03` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). * `04` –– Address is a College, Military Zone, or other type. * `05` –– Address no longer receives deliveries. * `06` –– Address is missing required secondary information. * `''` –– A DPV match is not made or the address is active.
* @return dpvInactiveReason
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Indicates the reason why an address is vacant or no longer receiving deliveries. Possible values are: * `01` –– Address does not receive mail from the USPS directly, but is serviced by a drop address. * `02` –– Address not yet deliverable. * `03` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). * `04` –– Address is a College, Military Zone, or other type. * `05` –– Address no longer receives deliveries. * `06` –– Address is missing required secondary information. * `''` –– A DPV match is not made or the address is active. ")
public DpvInactiveReasonEnum getDpvInactiveReason() {
return dpvInactiveReason;
}
/**
* Indicates a street address for which mail is delivered to a PO Box. Possible values are: * `Y` –– Address is a PO Box throwback delivery point. * `N` –– Address is not a PO Box throwback delivery point. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
*/
@JsonAdapter(DpvThrowbackEnum.Adapter.class)
public enum DpvThrowbackEnum {
Y("Y"),
N("N"),
EMPTY("");
private String value;
DpvThrowbackEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvThrowbackEnum fromValue(String value) {
for (DpvThrowbackEnum b : DpvThrowbackEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvThrowbackEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvThrowbackEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvThrowbackEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_THROWBACK = "dpv_throwback";
@SerializedName(SERIALIZED_NAME_DPV_THROWBACK)
private DpvThrowbackEnum dpvThrowback;
/**
* Indicates a street address for which mail is delivered to a PO Box. Possible values are: * `Y` –– Address is a PO Box throwback delivery point. * `N` –– Address is not a PO Box throwback delivery point. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
* @return dpvThrowback
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Indicates a street address for which mail is delivered to a PO Box. Possible values are: * `Y` –– Address is a PO Box throwback delivery point. * `N` –– Address is not a PO Box throwback delivery point. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). ")
public DpvThrowbackEnum getDpvThrowback() {
return dpvThrowback;
}
/**
* Indicates whether deliveries are not performed on one or more days of the week at an address. Possible values are: * `Y` –– Mail delivery does not occur on some days of the week. * `N` –– Mail delivery occurs every day of the week. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
*/
@JsonAdapter(DpvNonDeliveryDayFlagEnum.Adapter.class)
public enum DpvNonDeliveryDayFlagEnum {
Y("Y"),
N("N"),
EMPTY("");
private String value;
DpvNonDeliveryDayFlagEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvNonDeliveryDayFlagEnum fromValue(String value) {
for (DpvNonDeliveryDayFlagEnum b : DpvNonDeliveryDayFlagEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvNonDeliveryDayFlagEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvNonDeliveryDayFlagEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvNonDeliveryDayFlagEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_NON_DELIVERY_DAY_FLAG = "dpv_non_delivery_day_flag";
@SerializedName(SERIALIZED_NAME_DPV_NON_DELIVERY_DAY_FLAG)
private DpvNonDeliveryDayFlagEnum dpvNonDeliveryDayFlag;
/**
* Indicates whether deliveries are not performed on one or more days of the week at an address. Possible values are: * `Y` –– Mail delivery does not occur on some days of the week. * `N` –– Mail delivery occurs every day of the week. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
* @return dpvNonDeliveryDayFlag
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Indicates whether deliveries are not performed on one or more days of the week at an address. Possible values are: * `Y` –– Mail delivery does not occur on some days of the week. * `N` –– Mail delivery occurs every day of the week. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). ")
public DpvNonDeliveryDayFlagEnum getDpvNonDeliveryDayFlag() {
return dpvNonDeliveryDayFlag;
}
public static final String SERIALIZED_NAME_DPV_NON_DELIVERY_DAY_VALUES = "dpv_non_delivery_day_values";
@SerializedName(SERIALIZED_NAME_DPV_NON_DELIVERY_DAY_VALUES)
private String dpvNonDeliveryDayValues;
/**
* Indicates days of the week (starting on Sunday) deliveries are not performed at an address. For example: * `YNNNNNN` –– Mail delivery does not occur on Sunday's. * `NYNNNYN` –– Mail delivery does not occur on Monday's or Friday's. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string) or address receives mail every day of the week (`deliverability_analysis[dpv_non_delivery_day_flag]` is `N` or an empty string).
* @return dpvNonDeliveryDayValues
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Indicates days of the week (starting on Sunday) deliveries are not performed at an address. For example: * `YNNNNNN` –– Mail delivery does not occur on Sunday's. * `NYNNNYN` –– Mail delivery does not occur on Monday's or Friday's. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string) or address receives mail every day of the week (`deliverability_analysis[dpv_non_delivery_day_flag]` is `N` or an empty string). ")
public String getDpvNonDeliveryDayValues() {
return dpvNonDeliveryDayValues;
}
/**
* Indicates packages to this address will not be left due to security concerns. Possible values are: * `Y` –– Address does not have a secure mailbox. * `N` –– Address has a secure mailbox. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
*/
@JsonAdapter(DpvNoSecureLocationEnum.Adapter.class)
public enum DpvNoSecureLocationEnum {
Y("Y"),
N("N"),
EMPTY("");
private String value;
DpvNoSecureLocationEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvNoSecureLocationEnum fromValue(String value) {
for (DpvNoSecureLocationEnum b : DpvNoSecureLocationEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvNoSecureLocationEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvNoSecureLocationEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvNoSecureLocationEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_NO_SECURE_LOCATION = "dpv_no_secure_location";
@SerializedName(SERIALIZED_NAME_DPV_NO_SECURE_LOCATION)
private DpvNoSecureLocationEnum dpvNoSecureLocation;
/**
* Indicates packages to this address will not be left due to security concerns. Possible values are: * `Y` –– Address does not have a secure mailbox. * `N` –– Address has a secure mailbox. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
* @return dpvNoSecureLocation
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Indicates packages to this address will not be left due to security concerns. Possible values are: * `Y` –– Address does not have a secure mailbox. * `N` –– Address has a secure mailbox. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). ")
public DpvNoSecureLocationEnum getDpvNoSecureLocation() {
return dpvNoSecureLocation;
}
/**
* Indicates the door of the address is not accessible for mail delivery. Possible values are: * `Y` –– Door is not accessible. * `N` –– Door is accessible. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
*/
@JsonAdapter(DpvDoorNotAccessibleEnum.Adapter.class)
public enum DpvDoorNotAccessibleEnum {
Y("Y"),
N("N"),
EMPTY("");
private String value;
DpvDoorNotAccessibleEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static DpvDoorNotAccessibleEnum fromValue(String value) {
for (DpvDoorNotAccessibleEnum b : DpvDoorNotAccessibleEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final DpvDoorNotAccessibleEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public DpvDoorNotAccessibleEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return DpvDoorNotAccessibleEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_DPV_DOOR_NOT_ACCESSIBLE = "dpv_door_not_accessible";
@SerializedName(SERIALIZED_NAME_DPV_DOOR_NOT_ACCESSIBLE)
private DpvDoorNotAccessibleEnum dpvDoorNotAccessible;
/**
* Indicates the door of the address is not accessible for mail delivery. Possible values are: * `Y` –– Door is not accessible. * `N` –– Door is accessible. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
* @return dpvDoorNotAccessible
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Indicates the door of the address is not accessible for mail delivery. Possible values are: * `Y` –– Door is not accessible. * `N` –– Door is accessible. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). ")
public DpvDoorNotAccessibleEnum getDpvDoorNotAccessible() {
return dpvDoorNotAccessible;
}
public static final String SERIALIZED_NAME_DPV_FOOTNOTES = "dpv_footnotes";
@SerializedName(SERIALIZED_NAME_DPV_FOOTNOTES)
private List dpvFootnotes = new ArrayList<>();
public List getDpvFootnotes() {
if (this.dpvFootnotes == null) {
this.dpvFootnotes = new ArrayList();
}
return this.dpvFootnotes;
}
public static final String SERIALIZED_NAME_EWS_MATCH = "ews_match";
@SerializedName(SERIALIZED_NAME_EWS_MATCH)
private Boolean ewsMatch;
/**
* indicates whether or not an address has been flagged in the [Early Warning System](https://docs.informatica.com/data-engineering/data-engineering-quality/10-4-0/address-validator-port-reference/postal-carrier-certification-data-ports/early-warning-system-return-code.html), meaning the address is under development and not yet ready to receive mail. However, it should become available in a few months.
* @return ewsMatch
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "indicates whether or not an address has been flagged in the [Early Warning System](https://docs.informatica.com/data-engineering/data-engineering-quality/10-4-0/address-validator-port-reference/postal-carrier-certification-data-ports/early-warning-system-return-code.html), meaning the address is under development and not yet ready to receive mail. However, it should become available in a few months. ")
public Boolean getEwsMatch() {
return ewsMatch;
}
/**
* indicates whether this address has been converted by [LACS<sup>Link</sup>](https://postalpro.usps.com/address-quality/lacslink). LACS<sup>Link</sup> corrects outdated addresses into their modern counterparts. Possible values are: * `Y` –– New address produced with a matching record in LACS<sup>Link</sup>. * `N` –– New address could not be produced with a matching record in LACS<sup>Link</sup>. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
*/
@JsonAdapter(LacsIndicatorEnum.Adapter.class)
public enum LacsIndicatorEnum {
Y("Y"),
N("N"),
EMPTY("");
private String value;
LacsIndicatorEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static LacsIndicatorEnum fromValue(String value) {
for (LacsIndicatorEnum b : LacsIndicatorEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final LacsIndicatorEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public LacsIndicatorEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return LacsIndicatorEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_LACS_INDICATOR = "lacs_indicator";
@SerializedName(SERIALIZED_NAME_LACS_INDICATOR)
private LacsIndicatorEnum lacsIndicator;
/**
* indicates whether this address has been converted by [LACSLink](https://postalpro.usps.com/address-quality/lacslink). LACSLink corrects outdated addresses into their modern counterparts. Possible values are: * `Y` –– New address produced with a matching record in LACSLink. * `N` –– New address could not be produced with a matching record in LACSLink. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string).
* @return lacsIndicator
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "indicates whether this address has been converted by [LACSLink](https://postalpro.usps.com/address-quality/lacslink). LACSLink corrects outdated addresses into their modern counterparts. Possible values are: * `Y` –– New address produced with a matching record in LACSLink. * `N` –– New address could not be produced with a matching record in LACSLink. * `''` –– A DPV match is not made (`deliverability_analysis[dpv_confirmation]` is `N` or an empty string). ")
public LacsIndicatorEnum getLacsIndicator() {
return lacsIndicator;
}
public static final String SERIALIZED_NAME_LACS_RETURN_CODE = "lacs_return_code";
@SerializedName(SERIALIZED_NAME_LACS_RETURN_CODE)
private String lacsReturnCode;
/**
* A code indicating how `deliverability_analysis[lacs_indicator]` was determined. Possible values are: * `A` — A new address was produced because a match was found in LACSLink. * `92` — A LACSLink record was matched after dropping secondary information. * `14` — A match was found in LACSLink, but could not be converted to a deliverable address. * `00` — A match was not found in LACSLink, and no new address was produced. * `''` — LACSLink was not attempted.
* @return lacsReturnCode
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "A code indicating how `deliverability_analysis[lacs_indicator]` was determined. Possible values are: * `A` — A new address was produced because a match was found in LACSLink. * `92` — A LACSLink record was matched after dropping secondary information. * `14` — A match was found in LACSLink, but could not be converted to a deliverable address. * `00` — A match was not found in LACSLink, and no new address was produced. * `''` — LACSLink was not attempted. ")
public String getLacsReturnCode() {
return lacsReturnCode;
}
/**
* A return code that indicates whether the address was matched and corrected by [Suite<sup>Link</sup>](https://postalpro.usps.com/address-quality-solutions/suitelink). Suite<sup>Link</sup> attempts to provide secondary information to business addresses. Possible values are: * `A` –– A Suite<sup>Link</sup> match was found and secondary information was added. * `00` –– A Suite<sup>Link</sup> match could not be found and no secondary information was added. * `''` –– Suite<sup>Link</sup> lookup was not attempted.
*/
@JsonAdapter(SuiteReturnCodeEnum.Adapter.class)
public enum SuiteReturnCodeEnum {
A("A"),
_00("00"),
EMPTY("");
private String value;
SuiteReturnCodeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static SuiteReturnCodeEnum fromValue(String value) {
for (SuiteReturnCodeEnum b : SuiteReturnCodeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final SuiteReturnCodeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public SuiteReturnCodeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return SuiteReturnCodeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_SUITE_RETURN_CODE = "suite_return_code";
@SerializedName(SERIALIZED_NAME_SUITE_RETURN_CODE)
private SuiteReturnCodeEnum suiteReturnCode;
/**
* A return code that indicates whether the address was matched and corrected by [SuiteLink](https://postalpro.usps.com/address-quality-solutions/suitelink). SuiteLink attempts to provide secondary information to business addresses. Possible values are: * `A` –– A SuiteLink match was found and secondary information was added. * `00` –– A SuiteLink match could not be found and no secondary information was added. * `''` –– SuiteLink lookup was not attempted.
* @return suiteReturnCode
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "A return code that indicates whether the address was matched and corrected by [SuiteLink](https://postalpro.usps.com/address-quality-solutions/suitelink). SuiteLink attempts to provide secondary information to business addresses. Possible values are: * `A` –– A SuiteLink match was found and secondary information was added. * `00` –– A SuiteLink match could not be found and no secondary information was added. * `''` –– SuiteLink lookup was not attempted. ")
public SuiteReturnCodeEnum getSuiteReturnCode() {
return suiteReturnCode;
}
/*
public DeliverabilityAnalysis dpvConfirmation(DpvConfirmationEnum dpvConfirmation) {
this.dpvConfirmation = dpvConfirmation;
return this;
}
*/
public void setDpvConfirmation(DpvConfirmationEnum dpvConfirmation) {
this.dpvConfirmation = dpvConfirmation;
}
/*
public DeliverabilityAnalysis dpvCmra(DpvCmraEnum dpvCmra) {
this.dpvCmra = dpvCmra;
return this;
}
*/
public void setDpvCmra(DpvCmraEnum dpvCmra) {
this.dpvCmra = dpvCmra;
}
/*
public DeliverabilityAnalysis dpvVacant(DpvVacantEnum dpvVacant) {
this.dpvVacant = dpvVacant;
return this;
}
*/
public void setDpvVacant(DpvVacantEnum dpvVacant) {
this.dpvVacant = dpvVacant;
}
/*
public DeliverabilityAnalysis dpvActive(DpvActiveEnum dpvActive) {
this.dpvActive = dpvActive;
return this;
}
*/
public void setDpvActive(DpvActiveEnum dpvActive) {
this.dpvActive = dpvActive;
}
/*
public DeliverabilityAnalysis dpvInactiveReason(DpvInactiveReasonEnum dpvInactiveReason) {
this.dpvInactiveReason = dpvInactiveReason;
return this;
}
*/
public void setDpvInactiveReason(DpvInactiveReasonEnum dpvInactiveReason) {
this.dpvInactiveReason = dpvInactiveReason;
}
/*
public DeliverabilityAnalysis dpvThrowback(DpvThrowbackEnum dpvThrowback) {
this.dpvThrowback = dpvThrowback;
return this;
}
*/
public void setDpvThrowback(DpvThrowbackEnum dpvThrowback) {
this.dpvThrowback = dpvThrowback;
}
/*
public DeliverabilityAnalysis dpvNonDeliveryDayFlag(DpvNonDeliveryDayFlagEnum dpvNonDeliveryDayFlag) {
this.dpvNonDeliveryDayFlag = dpvNonDeliveryDayFlag;
return this;
}
*/
public void setDpvNonDeliveryDayFlag(DpvNonDeliveryDayFlagEnum dpvNonDeliveryDayFlag) {
this.dpvNonDeliveryDayFlag = dpvNonDeliveryDayFlag;
}
/*
public DeliverabilityAnalysis dpvNonDeliveryDayValues(String dpvNonDeliveryDayValues) {
this.dpvNonDeliveryDayValues = dpvNonDeliveryDayValues;
return this;
}
*/
public void setDpvNonDeliveryDayValues(String dpvNonDeliveryDayValues) {
this.dpvNonDeliveryDayValues = dpvNonDeliveryDayValues;
}
/*
public DeliverabilityAnalysis dpvNoSecureLocation(DpvNoSecureLocationEnum dpvNoSecureLocation) {
this.dpvNoSecureLocation = dpvNoSecureLocation;
return this;
}
*/
public void setDpvNoSecureLocation(DpvNoSecureLocationEnum dpvNoSecureLocation) {
this.dpvNoSecureLocation = dpvNoSecureLocation;
}
/*
public DeliverabilityAnalysis dpvDoorNotAccessible(DpvDoorNotAccessibleEnum dpvDoorNotAccessible) {
this.dpvDoorNotAccessible = dpvDoorNotAccessible;
return this;
}
*/
public void setDpvDoorNotAccessible(DpvDoorNotAccessibleEnum dpvDoorNotAccessible) {
this.dpvDoorNotAccessible = dpvDoorNotAccessible;
}
/*
public DeliverabilityAnalysis dpvFootnotes(List dpvFootnotes) {
this.dpvFootnotes = dpvFootnotes;
return this;
}
*/
public DeliverabilityAnalysis addDpvFootnotesItem(DpvFootnote dpvFootnotesItem) {
this.dpvFootnotes.add(dpvFootnotesItem);
return this;
}
public void setDpvFootnotes(List dpvFootnotes) {
this.dpvFootnotes = dpvFootnotes;
}
/*
public DeliverabilityAnalysis ewsMatch(Boolean ewsMatch) {
this.ewsMatch = ewsMatch;
return this;
}
*/
public void setEwsMatch(Boolean ewsMatch) {
this.ewsMatch = ewsMatch;
}
/*
public DeliverabilityAnalysis lacsIndicator(LacsIndicatorEnum lacsIndicator) {
this.lacsIndicator = lacsIndicator;
return this;
}
*/
public void setLacsIndicator(LacsIndicatorEnum lacsIndicator) {
this.lacsIndicator = lacsIndicator;
}
/*
public DeliverabilityAnalysis lacsReturnCode(String lacsReturnCode) {
this.lacsReturnCode = lacsReturnCode;
return this;
}
*/
public void setLacsReturnCode(String lacsReturnCode) {
this.lacsReturnCode = lacsReturnCode;
}
/*
public DeliverabilityAnalysis suiteReturnCode(SuiteReturnCodeEnum suiteReturnCode) {
this.suiteReturnCode = suiteReturnCode;
return this;
}
*/
public void setSuiteReturnCode(SuiteReturnCodeEnum suiteReturnCode) {
this.suiteReturnCode = suiteReturnCode;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DeliverabilityAnalysis deliverabilityAnalysis = (DeliverabilityAnalysis) o;
return Objects.equals(this.dpvConfirmation, deliverabilityAnalysis.dpvConfirmation) &&
Objects.equals(this.dpvCmra, deliverabilityAnalysis.dpvCmra) &&
Objects.equals(this.dpvVacant, deliverabilityAnalysis.dpvVacant) &&
Objects.equals(this.dpvActive, deliverabilityAnalysis.dpvActive) &&
Objects.equals(this.dpvInactiveReason, deliverabilityAnalysis.dpvInactiveReason) &&
Objects.equals(this.dpvThrowback, deliverabilityAnalysis.dpvThrowback) &&
Objects.equals(this.dpvNonDeliveryDayFlag, deliverabilityAnalysis.dpvNonDeliveryDayFlag) &&
Objects.equals(this.dpvNonDeliveryDayValues, deliverabilityAnalysis.dpvNonDeliveryDayValues) &&
Objects.equals(this.dpvNoSecureLocation, deliverabilityAnalysis.dpvNoSecureLocation) &&
Objects.equals(this.dpvDoorNotAccessible, deliverabilityAnalysis.dpvDoorNotAccessible) &&
Objects.equals(this.dpvFootnotes, deliverabilityAnalysis.dpvFootnotes) &&
Objects.equals(this.ewsMatch, deliverabilityAnalysis.ewsMatch) &&
Objects.equals(this.lacsIndicator, deliverabilityAnalysis.lacsIndicator) &&
Objects.equals(this.lacsReturnCode, deliverabilityAnalysis.lacsReturnCode) &&
Objects.equals(this.suiteReturnCode, deliverabilityAnalysis.suiteReturnCode);
}
@Override
public int hashCode() {
return Objects.hash(dpvConfirmation, dpvCmra, dpvVacant, dpvActive, dpvInactiveReason, dpvThrowback, dpvNonDeliveryDayFlag, dpvNonDeliveryDayValues, dpvNoSecureLocation, dpvDoorNotAccessible, dpvFootnotes, ewsMatch, lacsIndicator, lacsReturnCode, suiteReturnCode);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" dpvConfirmation: ").append(toIndentedString(dpvConfirmation)).append("\n");
sb.append(" dpvCmra: ").append(toIndentedString(dpvCmra)).append("\n");
sb.append(" dpvVacant: ").append(toIndentedString(dpvVacant)).append("\n");
sb.append(" dpvActive: ").append(toIndentedString(dpvActive)).append("\n");
sb.append(" dpvInactiveReason: ").append(toIndentedString(dpvInactiveReason)).append("\n");
sb.append(" dpvThrowback: ").append(toIndentedString(dpvThrowback)).append("\n");
sb.append(" dpvNonDeliveryDayFlag: ").append(toIndentedString(dpvNonDeliveryDayFlag)).append("\n");
sb.append(" dpvNonDeliveryDayValues: ").append(toIndentedString(dpvNonDeliveryDayValues)).append("\n");
sb.append(" dpvNoSecureLocation: ").append(toIndentedString(dpvNoSecureLocation)).append("\n");
sb.append(" dpvDoorNotAccessible: ").append(toIndentedString(dpvDoorNotAccessible)).append("\n");
sb.append(" dpvFootnotes: ").append(toIndentedString(dpvFootnotes)).append("\n");
sb.append(" ewsMatch: ").append(toIndentedString(ewsMatch)).append("\n");
sb.append(" lacsIndicator: ").append(toIndentedString(lacsIndicator)).append("\n");
sb.append(" lacsReturnCode: ").append(toIndentedString(lacsReturnCode)).append("\n");
sb.append(" suiteReturnCode: ").append(toIndentedString(suiteReturnCode)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("dpv_confirmation", dpvConfirmation);
localMap.put("dpv_cmra", dpvCmra);
localMap.put("dpv_vacant", dpvVacant);
localMap.put("dpv_active", dpvActive);
localMap.put("dpv_inactive_reason", dpvInactiveReason);
localMap.put("dpv_throwback", dpvThrowback);
localMap.put("dpv_non_delivery_day_flag", dpvNonDeliveryDayFlag);
localMap.put("dpv_non_delivery_day_values", dpvNonDeliveryDayValues);
localMap.put("dpv_no_secure_location", dpvNoSecureLocation);
localMap.put("dpv_door_not_accessible", dpvDoorNotAccessible);
localMap.put("dpv_footnotes", dpvFootnotes);
localMap.put("ews_match", ewsMatch);
localMap.put("lacs_indicator", lacsIndicator);
localMap.put("lacs_return_code", lacsReturnCode);
localMap.put("suite_return_code", suiteReturnCode);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}