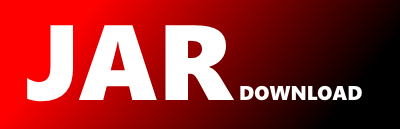
com.lob.model.IdentityValidation Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.openapitools.jackson.nullable.JsonNullable;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* IdentityValidation
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class IdentityValidation {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
/**
* Unique identifier prefixed with `id_validation_`.
* @return id
**/
@javax.annotation.Nullable
public String getId() { return id; }
public void setId (String id) throws IllegalArgumentException {
if(!id.matches("^id_validation_[a-zA-Z0-9_]+$")) {
throw new IllegalArgumentException("Invalid id provided");
}
this.id = id;
}
public static final String SERIALIZED_NAME_RECIPIENT = "recipient";
@SerializedName(SERIALIZED_NAME_RECIPIENT)
private String recipient;
/**
* The intended recipient, typically a person's or firm's name.
* @return recipient
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The intended recipient, typically a person's or firm's name.")
public String getRecipient() {
return recipient;
}
public static final String SERIALIZED_NAME_PRIMARY_LINE = "primary_line";
@SerializedName(SERIALIZED_NAME_PRIMARY_LINE)
private String primaryLine;
/**
* The primary delivery line (usually the street address) of the address. Combination of the following applicable `components`: * `primary_number` * `street_predirection` * `street_name` * `street_suffix` * `street_postdirection` * `secondary_designator` * `secondary_number` * `pmb_designator` * `pmb_number`
* @return primaryLine
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The primary delivery line (usually the street address) of the address. Combination of the following applicable `components`: * `primary_number` * `street_predirection` * `street_name` * `street_suffix` * `street_postdirection` * `secondary_designator` * `secondary_number` * `pmb_designator` * `pmb_number` ")
public String getPrimaryLine() {
return primaryLine;
}
public static final String SERIALIZED_NAME_SECONDARY_LINE = "secondary_line";
@SerializedName(SERIALIZED_NAME_SECONDARY_LINE)
private String secondaryLine;
/**
* The secondary delivery line of the address. This field is typically empty but may contain information if `primary_line` is too long.
* @return secondaryLine
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The secondary delivery line of the address. This field is typically empty but may contain information if `primary_line` is too long. ")
public String getSecondaryLine() {
return secondaryLine;
}
public static final String SERIALIZED_NAME_URBANIZATION = "urbanization";
@SerializedName(SERIALIZED_NAME_URBANIZATION)
private String urbanization;
/**
* Only present for addresses in Puerto Rico. An urbanization refers to an area, sector, or development within a city. See [USPS documentation](https://pe.usps.com/text/pub28/28api_008.htm#:~:text=I51.,-4%20Urbanizations&text=In%20Puerto%20Rico%2C%20identical%20street,placed%20before%20the%20urbanization%20name.) for clarification.
* @return urbanization
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Only present for addresses in Puerto Rico. An urbanization refers to an area, sector, or development within a city. See [USPS documentation](https://pe.usps.com/text/pub28/28api_008.htm#:~:text=I51.,-4%20Urbanizations&text=In%20Puerto%20Rico%2C%20identical%20street,placed%20before%20the%20urbanization%20name.) for clarification. ")
public String getUrbanization() {
return urbanization;
}
public static final String SERIALIZED_NAME_LAST_LINE = "last_line";
@SerializedName(SERIALIZED_NAME_LAST_LINE)
private String lastLine;
/**
* Combination of the following applicable `components`: * City (`city`) * State (`state`) * ZIP code (`zip_code`) * ZIP+4 (`zip_code_plus_4`)
* @return lastLine
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Combination of the following applicable `components`: * City (`city`) * State (`state`) * ZIP code (`zip_code`) * ZIP+4 (`zip_code_plus_4`) ")
public String getLastLine() {
return lastLine;
}
public static final String SERIALIZED_NAME_SCORE = "score";
@SerializedName(SERIALIZED_NAME_SCORE)
private Float score;
/**
* A numerical score between 0 and 100 that represents the likelihood the provided name is associated with a physical address.
* minimum: 0
* maximum: 100
* @return score
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A numerical score between 0 and 100 that represents the likelihood the provided name is associated with a physical address. ")
public Float getScore() {
return score;
}
/**
* Indicates the likelihood the recipient name and address match based on our custom internal calculation. Possible values are: - `high` — Has a Lob confidence score greater than 70. - `medium` — Has a Lob confidence score between 40 and 70. - `low` — Has a Lob confidence score less than 40. - `\"\"` — No tracking data exists for this address.
*/
@JsonAdapter(ConfidenceEnum.Adapter.class)
public enum ConfidenceEnum {
HIGH("high"),
MEDIUM("medium"),
LOW("low"),
EMPTY("");
private String value;
ConfidenceEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ConfidenceEnum fromValue(String value) {
for (ConfidenceEnum b : ConfidenceEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ConfidenceEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ConfidenceEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ConfidenceEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_CONFIDENCE = "confidence";
@SerializedName(SERIALIZED_NAME_CONFIDENCE)
private ConfidenceEnum confidence;
/**
* Indicates the likelihood the recipient name and address match based on our custom internal calculation. Possible values are: - `high` — Has a Lob confidence score greater than 70. - `medium` — Has a Lob confidence score between 40 and 70. - `low` — Has a Lob confidence score less than 40. - `\"\"` — No tracking data exists for this address.
* @return confidence
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates the likelihood the recipient name and address match based on our custom internal calculation. Possible values are: - `high` — Has a Lob confidence score greater than 70. - `medium` — Has a Lob confidence score between 40 and 70. - `low` — Has a Lob confidence score less than 40. - `\"\"` — No tracking data exists for this address. ")
public ConfidenceEnum getConfidence() {
return confidence;
}
/**
* Value is resource type.
*/
@JsonAdapter(ObjectEnum.Adapter.class)
public enum ObjectEnum {
ID_VALIDATION("id_validation");
private String value;
ObjectEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ObjectEnum fromValue(String value) {
for (ObjectEnum b : ObjectEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ObjectEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ObjectEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ObjectEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_OBJECT = "object";
@SerializedName(SERIALIZED_NAME_OBJECT)
private ObjectEnum _object = ObjectEnum.ID_VALIDATION;
/**
* Value is resource type.
* @return _object
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Value is resource type.")
public ObjectEnum getObject() {
return _object;
}
/*
public IdentityValidation id(String id) {
this.id = id;
return this;
}
*/
/*
public IdentityValidation recipient(String recipient) {
this.recipient = recipient;
return this;
}
*/
public void setRecipient(String recipient) {
this.recipient = recipient;
}
/*
public IdentityValidation primaryLine(String primaryLine) {
this.primaryLine = primaryLine;
return this;
}
*/
public void setPrimaryLine(String primaryLine) {
this.primaryLine = primaryLine;
}
/*
public IdentityValidation secondaryLine(String secondaryLine) {
this.secondaryLine = secondaryLine;
return this;
}
*/
public void setSecondaryLine(String secondaryLine) {
this.secondaryLine = secondaryLine;
}
/*
public IdentityValidation urbanization(String urbanization) {
this.urbanization = urbanization;
return this;
}
*/
public void setUrbanization(String urbanization) {
this.urbanization = urbanization;
}
/*
public IdentityValidation lastLine(String lastLine) {
this.lastLine = lastLine;
return this;
}
*/
public void setLastLine(String lastLine) {
this.lastLine = lastLine;
}
/*
public IdentityValidation score(Float score) {
this.score = score;
return this;
}
*/
public void setScore(Float score) {
this.score = score;
}
/*
public IdentityValidation confidence(ConfidenceEnum confidence) {
this.confidence = confidence;
return this;
}
*/
public void setConfidence(ConfidenceEnum confidence) {
this.confidence = confidence;
}
/*
public IdentityValidation _object(ObjectEnum _object) {
this._object = _object;
return this;
}
*/
public void setObject(ObjectEnum _object) {
this._object = _object;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
IdentityValidation identityValidation = (IdentityValidation) o;
return Objects.equals(this.id, identityValidation.id) &&
Objects.equals(this.recipient, identityValidation.recipient) &&
Objects.equals(this.primaryLine, identityValidation.primaryLine) &&
Objects.equals(this.secondaryLine, identityValidation.secondaryLine) &&
Objects.equals(this.urbanization, identityValidation.urbanization) &&
Objects.equals(this.lastLine, identityValidation.lastLine) &&
Objects.equals(this.score, identityValidation.score) &&
Objects.equals(this.confidence, identityValidation.confidence) &&
Objects.equals(this._object, identityValidation._object);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(id, recipient, primaryLine, secondaryLine, urbanization, lastLine, score, confidence, _object);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" recipient: ").append(toIndentedString(recipient)).append("\n");
sb.append(" primaryLine: ").append(toIndentedString(primaryLine)).append("\n");
sb.append(" secondaryLine: ").append(toIndentedString(secondaryLine)).append("\n");
sb.append(" urbanization: ").append(toIndentedString(urbanization)).append("\n");
sb.append(" lastLine: ").append(toIndentedString(lastLine)).append("\n");
sb.append(" score: ").append(toIndentedString(score)).append("\n");
sb.append(" confidence: ").append(toIndentedString(confidence)).append("\n");
sb.append(" _object: ").append(toIndentedString(_object)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("id", id);
localMap.put("recipient", recipient);
localMap.put("primary_line", primaryLine);
localMap.put("secondary_line", secondaryLine);
localMap.put("urbanization", urbanization);
localMap.put("last_line", lastLine);
localMap.put("score", score);
localMap.put("confidence", confidence);
localMap.put("object", _object);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}