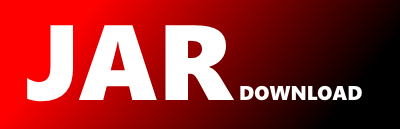
com.lob.model.LetterDetailsWritable Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.lob.model.MailType;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.openapitools.jackson.nullable.JsonNullable;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* Properties that the letters in your Creative should have.
*/
@ApiModel(description = "Properties that the letters in your Creative should have.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class LetterDetailsWritable {
/**
* Specifies the location of the address information that will show through the double-window envelope.
*/
@JsonAdapter(AddressPlacementEnum.Adapter.class)
public enum AddressPlacementEnum {
TOP_FIRST_PAGE("top_first_page"),
INSERT_BLANK_PAGE("insert_blank_page"),
BOTTOM_FIRST_PAGE_CENTER("bottom_first_page_center"),
BOTTOM_FIRST_PAGE("bottom_first_page");
private String value;
AddressPlacementEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static AddressPlacementEnum fromValue(String value) {
for (AddressPlacementEnum b : AddressPlacementEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final AddressPlacementEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public AddressPlacementEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return AddressPlacementEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_ADDRESS_PLACEMENT = "address_placement";
@SerializedName(SERIALIZED_NAME_ADDRESS_PLACEMENT)
private AddressPlacementEnum addressPlacement = AddressPlacementEnum.TOP_FIRST_PAGE;
/**
* Specifies the location of the address information that will show through the double-window envelope.
* @return addressPlacement
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the location of the address information that will show through the double-window envelope. ")
public AddressPlacementEnum getAddressPlacement() {
return addressPlacement;
}
public static final String SERIALIZED_NAME_CARDS = "cards";
@SerializedName(SERIALIZED_NAME_CARDS)
private List cards = new ArrayList<>();
public static final String SERIALIZED_NAME_COLOR = "color";
@SerializedName(SERIALIZED_NAME_COLOR)
private Boolean color;
/**
* Set this key to `true` if you would like to print in color. Set to `false` if you would like to print in black and white.
* @return color
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Set this key to `true` if you would like to print in color. Set to `false` if you would like to print in black and white.")
public Boolean getColor() {
return color;
}
public static final String SERIALIZED_NAME_DOUBLE_SIDED = "double_sided";
@SerializedName(SERIALIZED_NAME_DOUBLE_SIDED)
private Boolean doubleSided = true;
/**
* Set this attribute to `true` for double sided printing, or `false` for for single sided printing. Defaults to `true`.
* @return doubleSided
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set this attribute to `true` for double sided printing, or `false` for for single sided printing. Defaults to `true`.")
public Boolean getDoubleSided() {
return doubleSided;
}
public static final String SERIALIZED_NAME_EXTRA_SERVICE = "extra_service";
@SerializedName(SERIALIZED_NAME_EXTRA_SERVICE)
private String extraService;
/**
* Add an extra service to your letter.
* @return extraService
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Add an extra service to your letter.")
public String getExtraService() {
return extraService;
}
public static final String SERIALIZED_NAME_MAIL_TYPE = "mail_type";
@SerializedName(SERIALIZED_NAME_MAIL_TYPE)
private MailType mailType = MailType.FIRST_CLASS;
/**
* Get mailType
* @return mailType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public MailType getMailType() {
return mailType;
}
public static final String SERIALIZED_NAME_RETURN_ENVELOPE = "return_envelope";
@SerializedName(SERIALIZED_NAME_RETURN_ENVELOPE)
private Boolean returnEnvelope = false;
/**
* Get returnEnvelope
* @return returnEnvelope
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getReturnEnvelope() {
return returnEnvelope;
}
public static final String SERIALIZED_NAME_CUSTOM_ENVELOPE = "custom_envelope";
@SerializedName(SERIALIZED_NAME_CUSTOM_ENVELOPE)
private String customEnvelope;
/**
* Accepts an envelope ID for any customized envelope with available inventory.
* @return customEnvelope
**/
@javax.annotation.Nullable
public String getCustomEnvelope() { return customEnvelope; }
public void setCustomEnvelope (String customEnvelope) throws IllegalArgumentException {
if(!customEnvelope.matches("^env_[a-zA-Z0-9]+$")) {
throw new IllegalArgumentException("Invalid custom_envelope provided");
}
this.customEnvelope = customEnvelope;
}
/*
public LetterDetailsWritable addressPlacement(AddressPlacementEnum addressPlacement) {
this.addressPlacement = addressPlacement;
return this;
}
*/
public void setAddressPlacement(AddressPlacementEnum addressPlacement) {
this.addressPlacement = addressPlacement;
}
/*
public LetterDetailsWritable cards(List cards) {
this.cards = cards;
return this;
}
*/
public LetterDetailsWritable addCardsItem(String cardsItem) {
this.cards.add(cardsItem);
return this;
}
public void setCards(List cards) {
this.cards = cards;
}
/*
public LetterDetailsWritable color(Boolean color) {
this.color = color;
return this;
}
*/
public void setColor(Boolean color) {
this.color = color;
}
/*
public LetterDetailsWritable doubleSided(Boolean doubleSided) {
this.doubleSided = doubleSided;
return this;
}
*/
public void setDoubleSided(Boolean doubleSided) {
this.doubleSided = doubleSided;
}
/*
public LetterDetailsWritable extraService(String extraService) {
this.extraService = extraService;
return this;
}
*/
public void setExtraService(String extraService) {
this.extraService = extraService;
}
/*
public LetterDetailsWritable mailType(MailType mailType) {
this.mailType = mailType;
return this;
}
*/
public void setMailType(MailType mailType) {
this.mailType = mailType;
}
/*
public LetterDetailsWritable returnEnvelope(Boolean returnEnvelope) {
this.returnEnvelope = returnEnvelope;
return this;
}
*/
public void setReturnEnvelope(Boolean returnEnvelope) {
this.returnEnvelope = returnEnvelope;
}
/*
public LetterDetailsWritable customEnvelope(String customEnvelope) {
this.customEnvelope = customEnvelope;
return this;
}
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LetterDetailsWritable letterDetailsWritable = (LetterDetailsWritable) o;
return Objects.equals(this.addressPlacement, letterDetailsWritable.addressPlacement) &&
Objects.equals(this.cards, letterDetailsWritable.cards) &&
Objects.equals(this.color, letterDetailsWritable.color) &&
Objects.equals(this.doubleSided, letterDetailsWritable.doubleSided) &&
Objects.equals(this.extraService, letterDetailsWritable.extraService) &&
Objects.equals(this.mailType, letterDetailsWritable.mailType) &&
Objects.equals(this.returnEnvelope, letterDetailsWritable.returnEnvelope) &&
Objects.equals(this.customEnvelope, letterDetailsWritable.customEnvelope);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(addressPlacement, cards, color, doubleSided, extraService, mailType, returnEnvelope, customEnvelope);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" addressPlacement: ").append(toIndentedString(addressPlacement)).append("\n");
sb.append(" cards: ").append(toIndentedString(cards)).append("\n");
sb.append(" color: ").append(toIndentedString(color)).append("\n");
sb.append(" doubleSided: ").append(toIndentedString(doubleSided)).append("\n");
sb.append(" extraService: ").append(toIndentedString(extraService)).append("\n");
sb.append(" mailType: ").append(toIndentedString(mailType)).append("\n");
sb.append(" returnEnvelope: ").append(toIndentedString(returnEnvelope)).append("\n");
sb.append(" customEnvelope: ").append(toIndentedString(customEnvelope)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("address_placement", addressPlacement);
localMap.put("cards", cards);
localMap.put("color", color);
localMap.put("double_sided", doubleSided);
localMap.put("extra_service", extraService);
localMap.put("mail_type", mailType);
localMap.put("return_envelope", returnEnvelope);
localMap.put("custom_envelope", customEnvelope);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}