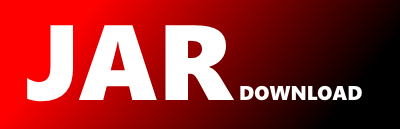
com.lob.model.LobConfidenceScore Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.openapitools.jackson.nullable.JsonNullable;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* Lob Confidence Score is a nested object that provides a numerical value between 0-100 of the likelihood that an address is deliverable based on Lob’s mail delivery data to over half of US households.
*/
@ApiModel(description = "Lob Confidence Score is a nested object that provides a numerical value between 0-100 of the likelihood that an address is deliverable based on Lob’s mail delivery data to over half of US households.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class LobConfidenceScore {
public static final String SERIALIZED_NAME_SCORE = "score";
@SerializedName(SERIALIZED_NAME_SCORE)
private Float score;
/**
* A numerical score between 0 and 100 that represents the percentage of mailpieces Lob has sent to this addresses that have been delivered successfully over the past 2 years. Will be `null` if no tracking data exists for this address.
* minimum: 0
* maximum: 100
* @return score
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A numerical score between 0 and 100 that represents the percentage of mailpieces Lob has sent to this addresses that have been delivered successfully over the past 2 years. Will be `null` if no tracking data exists for this address. ")
public Float getScore() {
return score;
}
/**
* indicates the likelihood that the address is a valid, mail-receiving address. Possible values are: - `high` — Over 70% of mailpieces Lob has sent to this address were delivered successfully and recent mailings were also successful. - `medium` — Between 40% and 70% of mailpieces Lob has sent to this address were delivered successfully. - `low` — Less than 40% of mailpieces Lob has sent to this address were delivered successfully and recent mailings weren't successful. - `\"\"` — No tracking data exists for this address or lob deliverability was unable to find a corresponding level of mail success.
*/
@JsonAdapter(LevelEnum.Adapter.class)
public enum LevelEnum {
HIGH("high"),
MEDIUM("medium"),
LOW("low"),
EMPTY("");
private String value;
LevelEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static LevelEnum fromValue(String value) {
for (LevelEnum b : LevelEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final LevelEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public LevelEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return LevelEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_LEVEL = "level";
@SerializedName(SERIALIZED_NAME_LEVEL)
private LevelEnum level;
/**
* indicates the likelihood that the address is a valid, mail-receiving address. Possible values are: - `high` — Over 70% of mailpieces Lob has sent to this address were delivered successfully and recent mailings were also successful. - `medium` — Between 40% and 70% of mailpieces Lob has sent to this address were delivered successfully. - `low` — Less than 40% of mailpieces Lob has sent to this address were delivered successfully and recent mailings weren't successful. - `\"\"` — No tracking data exists for this address or lob deliverability was unable to find a corresponding level of mail success.
* @return level
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "indicates the likelihood that the address is a valid, mail-receiving address. Possible values are: - `high` — Over 70% of mailpieces Lob has sent to this address were delivered successfully and recent mailings were also successful. - `medium` — Between 40% and 70% of mailpieces Lob has sent to this address were delivered successfully. - `low` — Less than 40% of mailpieces Lob has sent to this address were delivered successfully and recent mailings weren't successful. - `\"\"` — No tracking data exists for this address or lob deliverability was unable to find a corresponding level of mail success. ")
public LevelEnum getLevel() {
return level;
}
/*
public LobConfidenceScore score(Float score) {
this.score = score;
return this;
}
*/
public void setScore(Float score) {
this.score = score;
}
/*
public LobConfidenceScore level(LevelEnum level) {
this.level = level;
return this;
}
*/
public void setLevel(LevelEnum level) {
this.level = level;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LobConfidenceScore lobConfidenceScore = (LobConfidenceScore) o;
return Objects.equals(this.score, lobConfidenceScore.score) &&
Objects.equals(this.level, lobConfidenceScore.level);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(score, level);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" score: ").append(toIndentedString(score)).append("\n");
sb.append(" level: ").append(toIndentedString(level)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("score", score);
localMap.put("level", level);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}