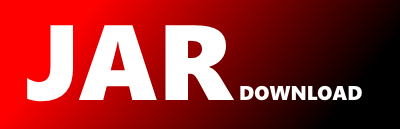
com.lob.model.QrCode Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* Customize and place a QR code on the creative at the required position.
*/
@ApiModel(description = "Customize and place a QR code on the creative at the required position.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class QrCode {
/**
* Sets how a QR code is being positioned in the document.
*/
@JsonAdapter(PositionEnum.Adapter.class)
public enum PositionEnum {
FIXED("fixed"),
RELATIVE("relative");
private String value;
PositionEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PositionEnum fromValue(String value) {
for (PositionEnum b : PositionEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PositionEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PositionEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PositionEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_POSITION = "position";
@SerializedName(SERIALIZED_NAME_POSITION)
private PositionEnum position;
/**
* Sets how a QR code is being positioned in the document.
* @return position
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Sets how a QR code is being positioned in the document.")
public PositionEnum getPosition() {
return position;
}
public static final String SERIALIZED_NAME_TOP = "top";
@SerializedName(SERIALIZED_NAME_TOP)
private String top;
/**
* Vertical distance(in inches) to place QR code from the top.
* @return top
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Vertical distance(in inches) to place QR code from the top.")
public String getTop() {
return top;
}
public static final String SERIALIZED_NAME_RIGHT = "right";
@SerializedName(SERIALIZED_NAME_RIGHT)
private String right;
/**
* Horizonal distance(in inches) to place QR code from the right.
* @return right
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Horizonal distance(in inches) to place QR code from the right.")
public String getRight() {
return right;
}
public static final String SERIALIZED_NAME_LEFT = "left";
@SerializedName(SERIALIZED_NAME_LEFT)
private String left;
/**
* Horizonal distance(in inches) to place QR code from the left.
* @return left
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Horizonal distance(in inches) to place QR code from the left.")
public String getLeft() {
return left;
}
public static final String SERIALIZED_NAME_BOTTOM = "bottom";
@SerializedName(SERIALIZED_NAME_BOTTOM)
private String bottom;
/**
* Vertical distance(in inches) to place QR code from the bottom.
* @return bottom
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Vertical distance(in inches) to place QR code from the bottom.")
public String getBottom() {
return bottom;
}
public static final String SERIALIZED_NAME_REDIRECT_URL = "redirect_url";
@SerializedName(SERIALIZED_NAME_REDIRECT_URL)
private String redirectUrl;
/**
* The url to redirect the user when a QR code is scanned. The url must start with `https://`
* @return redirectUrl
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The url to redirect the user when a QR code is scanned. The url must start with `https://`")
public String getRedirectUrl() {
return redirectUrl;
}
public static final String SERIALIZED_NAME_WIDTH = "width";
@SerializedName(SERIALIZED_NAME_WIDTH)
private String width;
/**
* The size(in inches) of the QR code. All QR codes are generated as a square.
* @return width
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The size(in inches) of the QR code. All QR codes are generated as a square.")
public String getWidth() {
return width;
}
/*
public QrCode position(PositionEnum position) {
this.position = position;
return this;
}
*/
public void setPosition(PositionEnum position) {
this.position = position;
}
/*
public QrCode top(String top) {
this.top = top;
return this;
}
*/
public void setTop(String top) {
this.top = top;
}
/*
public QrCode right(String right) {
this.right = right;
return this;
}
*/
public void setRight(String right) {
this.right = right;
}
/*
public QrCode left(String left) {
this.left = left;
return this;
}
*/
public void setLeft(String left) {
this.left = left;
}
/*
public QrCode bottom(String bottom) {
this.bottom = bottom;
return this;
}
*/
public void setBottom(String bottom) {
this.bottom = bottom;
}
/*
public QrCode redirectUrl(String redirectUrl) {
this.redirectUrl = redirectUrl;
return this;
}
*/
public void setRedirectUrl(String redirectUrl) {
this.redirectUrl = redirectUrl;
}
/*
public QrCode width(String width) {
this.width = width;
return this;
}
*/
public void setWidth(String width) {
this.width = width;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
QrCode qrCode = (QrCode) o;
return Objects.equals(this.position, qrCode.position) &&
Objects.equals(this.top, qrCode.top) &&
Objects.equals(this.right, qrCode.right) &&
Objects.equals(this.left, qrCode.left) &&
Objects.equals(this.bottom, qrCode.bottom) &&
Objects.equals(this.redirectUrl, qrCode.redirectUrl) &&
Objects.equals(this.width, qrCode.width);
}
@Override
public int hashCode() {
return Objects.hash(position, top, right, left, bottom, redirectUrl, width);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" position: ").append(toIndentedString(position)).append("\n");
sb.append(" top: ").append(toIndentedString(top)).append("\n");
sb.append(" right: ").append(toIndentedString(right)).append("\n");
sb.append(" left: ").append(toIndentedString(left)).append("\n");
sb.append(" bottom: ").append(toIndentedString(bottom)).append("\n");
sb.append(" redirectUrl: ").append(toIndentedString(redirectUrl)).append("\n");
sb.append(" width: ").append(toIndentedString(width)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("position", position);
localMap.put("top", top);
localMap.put("right", right);
localMap.put("left", left);
localMap.put("bottom", bottom);
localMap.put("redirect_url", redirectUrl);
localMap.put("width", width);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}