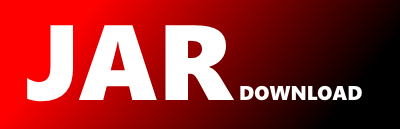
com.lob.model.RequiredAddressColumnMapping Maven / Gradle / Ivy
Show all versions of lob-java Show documentation
/*
* Lob
* The Lob API is organized around REST. Our API is designed to have predictable, resource-oriented URLs and uses HTTP response codes to indicate any API errors. Looking for our [previous documentation](https://lob.github.io/legacy-docs/)?
*
* The version of the OpenAPI document: 1.3.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.lob.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
/**
* The mapping of column headers in your file to Lob-required fields for the resource created. See our <a href=\"https://help.lob.com/print-and-mail/building-a-mail-strategy/campaign-or-triggered-sends/campaign-audience-guide#required-columns-2\" target=\"_blank\">Campaign Audience Guide</a> for additional details.
*/
@ApiModel(description = "The mapping of column headers in your file to Lob-required fields for the resource created. See our Campaign Audience Guide for additional details.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class RequiredAddressColumnMapping {
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name = "null";
/**
* The column header from the csv file that should be mapped to the required field `name`
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The column header from the csv file that should be mapped to the required field `name`")
public String getName() {
return name;
}
public static final String SERIALIZED_NAME_ADDRESS_LINE1 = "address_line1";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINE1)
private String addressLine1 = "null";
/**
* The column header from the csv file that should be mapped to the required field `address_line1`
* @return addressLine1
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The column header from the csv file that should be mapped to the required field `address_line1`")
public String getAddressLine1() {
return addressLine1;
}
public static final String SERIALIZED_NAME_ADDRESS_CITY = "address_city";
@SerializedName(SERIALIZED_NAME_ADDRESS_CITY)
private String addressCity = "null";
/**
* The column header from the csv file that should be mapped to the required field `address_city`
* @return addressCity
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The column header from the csv file that should be mapped to the required field `address_city`")
public String getAddressCity() {
return addressCity;
}
public static final String SERIALIZED_NAME_ADDRESS_STATE = "address_state";
@SerializedName(SERIALIZED_NAME_ADDRESS_STATE)
private String addressState = "null";
/**
* The column header from the csv file that should be mapped to the required field `address_state`
* @return addressState
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The column header from the csv file that should be mapped to the required field `address_state`")
public String getAddressState() {
return addressState;
}
public static final String SERIALIZED_NAME_ADDRESS_ZIP = "address_zip";
@SerializedName(SERIALIZED_NAME_ADDRESS_ZIP)
private String addressZip = "null";
/**
* The column header from the csv file that should be mapped to the required field `address_zip`
* @return addressZip
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The column header from the csv file that should be mapped to the required field `address_zip`")
public String getAddressZip() {
return addressZip;
}
/*
public RequiredAddressColumnMapping name(String name) {
this.name = name;
return this;
}
*/
public void setName(String name) {
this.name = name;
}
/*
public RequiredAddressColumnMapping addressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
return this;
}
*/
public void setAddressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
}
/*
public RequiredAddressColumnMapping addressCity(String addressCity) {
this.addressCity = addressCity;
return this;
}
*/
public void setAddressCity(String addressCity) {
this.addressCity = addressCity;
}
/*
public RequiredAddressColumnMapping addressState(String addressState) {
this.addressState = addressState;
return this;
}
*/
public void setAddressState(String addressState) {
this.addressState = addressState;
}
/*
public RequiredAddressColumnMapping addressZip(String addressZip) {
this.addressZip = addressZip;
return this;
}
*/
public void setAddressZip(String addressZip) {
this.addressZip = addressZip;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RequiredAddressColumnMapping requiredAddressColumnMapping = (RequiredAddressColumnMapping) o;
return Objects.equals(this.name, requiredAddressColumnMapping.name) &&
Objects.equals(this.addressLine1, requiredAddressColumnMapping.addressLine1) &&
Objects.equals(this.addressCity, requiredAddressColumnMapping.addressCity) &&
Objects.equals(this.addressState, requiredAddressColumnMapping.addressState) &&
Objects.equals(this.addressZip, requiredAddressColumnMapping.addressZip);
}
@Override
public int hashCode() {
return Objects.hash(name, addressLine1, addressCity, addressState, addressZip);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" addressLine1: ").append(toIndentedString(addressLine1)).append("\n");
sb.append(" addressCity: ").append(toIndentedString(addressCity)).append("\n");
sb.append(" addressState: ").append(toIndentedString(addressState)).append("\n");
sb.append(" addressZip: ").append(toIndentedString(addressZip)).append("\n");
sb.append("}");
return sb.toString();
}
public Map toMap() {
Map localMap = new HashMap();
localMap.put("name", name);
localMap.put("address_line1", addressLine1);
localMap.put("address_city", addressCity);
localMap.put("address_state", addressState);
localMap.put("address_zip", addressZip);
return localMap;
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}