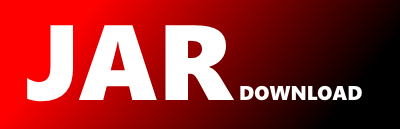
com.logicommerce.sdk.models.order.implementations.OrderTotalImpl Maven / Gradle / Ivy
package com.logicommerce.sdk.models.order.implementations;
import com.logicommerce.sdk.models.order.OrderTotal;
/**
* OrderTotalImpl class.
*
* @author Logicommerce
* @since 1.0.16
*/
public class OrderTotalImpl implements OrderTotal {
private double subtotal;
private double subtotalItems;
private double subtotalPaymentSystem;
private double subtotalShippings;
private double total;
private double totalDiscounts;
private double totalItems;
private double totalPaymentSystem;
private double totalShippings;
private double totalTaxes;
private double totalVouchers;
/**
* Getter for the field subtotal
.
*
* @return a double
*/
public double getSubtotal() {
return subtotal;
}
/**
* Getter for the field subtotalItems
.
*
* @return a double
*/
public double getSubtotalItems() {
return subtotalItems;
}
/**
* Getter for the field subtotalPaymentSystem
.
*
* @return a double
*/
public double getSubtotalPaymentSystem() {
return subtotalPaymentSystem;
}
/**
* Getter for the field subtotalShippings
.
*
* @return a double
*/
public double getSubtotalShippings() {
return subtotalShippings;
}
/**
* Getter for the field total
.
*
* @return a double
*/
public double getTotal() {
return total;
}
/**
* Getter for the field totalDiscounts
.
*
* @return a double
*/
public double getTotalDiscounts() {
return totalDiscounts;
}
/**
* Getter for the field totalItems
.
*
* @return a double
*/
public double getTotalItems() {
return totalItems;
}
/**
* Getter for the field totalPaymentSystem
.
*
* @return a double
*/
public double getTotalPaymentSystem() {
return totalPaymentSystem;
}
/**
* Getter for the field totalShippings
.
*
* @return a double
*/
public double getTotalShippings() {
return totalShippings;
}
/**
* Getter for the field totalTaxes
.
*
* @return a double
*/
public double getTotalTaxes() {
return totalTaxes;
}
/**
* Getter for the field totalVouchers
.
*
* @return a double
*/
public double getTotalVouchers() {
return totalVouchers;
}
/**
* Setter for the field subtotal
.
*
* @param subtotal a double
*/
public void setSubtotal(double subtotal) {
this.subtotal = subtotal;
}
/**
* Setter for the field subtotalItems
.
*
* @param subtotalItems a double
*/
public void setSubtotalItems(double subtotalItems) {
this.subtotalItems = subtotalItems;
}
/**
* Setter for the field subtotalPaymentSystem
.
*
* @param subtotalPaymentSystem a double
*/
public void setSubtotalPaymentSystem(double subtotalPaymentSystem) {
this.subtotalPaymentSystem = subtotalPaymentSystem;
}
/**
* Setter for the field subtotalShippings
.
*
* @param subtotalShippings a double
*/
public void setSubtotalShippings(double subtotalShippings) {
this.subtotalShippings = subtotalShippings;
}
/**
* Setter for the field total
.
*
* @param total a double
*/
public void setTotal(double total) {
this.total = total;
}
/**
* Setter for the field totalDiscounts
.
*
* @param totalDiscounts a double
*/
public void setTotalDiscounts(double totalDiscounts) {
this.totalDiscounts = totalDiscounts;
}
/**
* Setter for the field totalItems
.
*
* @param totalItems a double
*/
public void setTotalItems(double totalItems) {
this.totalItems = totalItems;
}
/**
* Setter for the field totalPaymentSystem
.
*
* @param totalPaymentSystem a double
*/
public void setTotalPaymentSystem(double totalPaymentSystem) {
this.totalPaymentSystem = totalPaymentSystem;
}
/**
* Setter for the field totalShippings
.
*
* @param totalShippings a double
*/
public void setTotalShippings(double totalShippings) {
this.totalShippings = totalShippings;
}
/**
* Setter for the field totalTaxes
.
*
* @param totalTaxes a double
*/
public void setTotalTaxes(double totalTaxes) {
this.totalTaxes = totalTaxes;
}
/**
* Setter for the field totalVouchers
.
*
* @param totalVouchers a double
*/
public void setTotalVouchers(double totalVouchers) {
this.totalVouchers = totalVouchers;
}
}