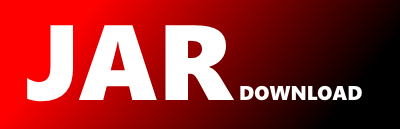
com.logicommerce.sdk.models.order.implementations.DocumentImpl Maven / Gradle / Ivy
Show all versions of sdk Show documentation
package com.logicommerce.sdk.models.order.implementations;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import com.logicommerce.sdk.enums.ExportStatusType;
import com.logicommerce.sdk.models.CustomTag;
import com.logicommerce.sdk.models.ElementProperty;
import com.logicommerce.sdk.models.ElementProperyImpl;
import com.logicommerce.sdk.models.implementations.CustomTagImpl;
import com.logicommerce.sdk.models.order.Document;
import com.logicommerce.sdk.models.order.OrderAdditionalInformation;
import com.logicommerce.sdk.models.order.OrderCurrency;
import com.logicommerce.sdk.models.order.OrderDelivery;
import com.logicommerce.sdk.models.order.OrderDiscount;
import com.logicommerce.sdk.models.order.OrderInformation;
import com.logicommerce.sdk.models.order.OrderItem;
import com.logicommerce.sdk.models.order.OrderPaymentSystem;
import com.logicommerce.sdk.models.order.OrderStatus;
import com.logicommerce.sdk.models.order.OrderTax;
import com.logicommerce.sdk.models.order.OrderTotal;
import com.logicommerce.sdk.models.order.OrderUser;
import com.logicommerce.sdk.models.order.OrderVoucher;
import com.logicommerce.utilities.annotations.NoMappable;
import com.logicommerce.utilities.annotations.Uses;
public abstract class DocumentImpl implements Document {
private String comment;
@Uses(value = CustomTagImpl.class)
private List customTags;
private Integer languageId;
@Uses(value = OrderUserImpl.class)
private OrderUser user;
@Uses(value = OrderTotalImpl.class)
private OrderTotal totals;
@Uses(value = OrderVoucherImpl.class)
private List vouchers;
@Uses(value = OrderItemImpl.class)
private List items;
@Uses(value = OrderPaymentSystemImpl.class)
private OrderPaymentSystem paymentSystem;
@Uses(value = OrderAdditionalInformationImpl.class)
private List additionalInformation;
@NoMappable
private List properties;
@Uses(value = OrderCurrencyImpl.class)
private List currencies;
private LocalDateTime date;
private LocalDateTime deliveryDate;
@Uses(value = OrderDiscountImpl.class)
private List discounts;
private String documentNumber;
private ExportStatusType exportStatus;
private Integer id;
private String pId;
private Integer documentId;
private LocalDateTime paymentDate;
@Uses(value = OrderDeliveryImpl.class)
private OrderDelivery delivery;
@Uses(value = OrderStatusImpl.class)
private List statuses;
private boolean paid;
private boolean reverseChargeVat;
@Uses(value = OrderInformationImpl.class)
private OrderInformation information;
@Uses(value = OrderTaxImpl.class)
private List taxes;
/**
* Getter for the field additionalInformation
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getAdditionalInformation() {
return additionalInformation;
}
/**
* Getter for the field properties
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getProperties() {
return properties;
}
/** {@inheritDoc} */
@Override
public void addProperty(ElementProperty property) {
if (properties == null) {
properties = new ArrayList<>();
}
properties.add(property);
}
/** {@inheritDoc} */
@Override
public void addProperty(String name, String value) {
if (name == null || value == null) {
return;
}
addProperty(new ElementProperyImpl(name, value));
}
/**
* Getter for the field comment
.
*
* @return a {@link java.lang.String} object
*/
@Override
public String getComment() {
return comment;
}
/**
* Getter for the field currencies
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getCurrencies() {
return currencies;
}
/**
* Getter for the field customTags
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getCustomTags() {
return customTags;
}
/**
* Getter for the field date
.
*
* @return a {@link java.time.LocalDateTime} object
*/
@Override
public LocalDateTime getDate() {
return date;
}
/**
* Getter for the field deliveryDate
.
*
* @return a {@link java.time.LocalDateTime} object
*/
@Override
public LocalDateTime getDeliveryDate() {
return deliveryDate;
}
/**
* Getter for the field discounts
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getDiscounts() {
return discounts;
}
/**
* Getter for the field documentNumber
.
*
* @return a {@link java.lang.String} object
*/
@Override
public String getDocumentNumber() {
return documentNumber;
}
/**
* Getter for the field exportStatus
.
*
* @return a {@link com.logicommerce.sdk.enums.ExportStatusType} object
*/
@Override
public ExportStatusType getExportStatus() {
return exportStatus;
}
/**
* Getter for the field id
.
*
* @return a {@link java.lang.Integer} object
*/
@Override
public Integer getId() {
return id;
}
/**
* Getter for the field items
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getItems() {
return items;
}
/**
* Getter for the field languageId
.
*
* @return a {@link java.lang.Integer} object
*/
@Override
public Integer getLanguageId() {
return languageId;
}
/**
* Getter for the field pId
.
*
* @return a {@link java.lang.String} object
*/
@Override
public String getPId() {
return pId;
}
/**
* Getter for the field documentId
.
*
* @return a {@link java.lang.String} object
*/
@Override
public Integer getDocumentId() {
return documentId;
}
/**
* Getter for the field paymentDate
.
*
* @return a {@link java.time.LocalDateTime} object
*/
@Override
public LocalDateTime getPaymentDate() {
return paymentDate;
}
/**
* Getter for the field information
.
*
* @return a {@link com.logicommerce.sdk.models.order.OrderInformation} object
*/
@Override
public OrderInformation getInformation() {
return information;
}
/**
* Getter for the field paymentSystem
.
*
* @return a {@link com.logicommerce.sdk.models.order.OrderPaymentSystem} object
*/
@Override
public OrderPaymentSystem getPaymentSystem() {
return paymentSystem;
}
/**
* Getter for the field statuses
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getStatuses() {
return statuses;
}
/**
* Getter for the field taxes
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getTaxes() {
return taxes;
}
/**
* Getter for the field totals
.
*
* @return a {@link com.logicommerce.sdk.models.order.OrderTotal} object
*/
@Override
public OrderTotal getTotals() {
return totals;
}
/**
* Getter for the field user
.
*
* @return a {@link com.logicommerce.sdk.models.order.OrderUser} object
*/
@Override
public OrderUser getUser() {
return user;
}
/**
* Getter for the field delivery
.
*
* @return a {@link com.logicommerce.sdk.models.order.OrderDelivery} object
*/
@Override
public OrderDelivery getDelivery() {
return delivery;
}
/**
* Getter for the field vouchers
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getVouchers() {
return vouchers;
}
/**
* isPaid.
*
* @return a boolean
*/
@Override
public boolean isPaid() {
return paid;
}
/**
* isReverseChargeVat.
*
* @return a boolean
*/
@Override
public boolean isReverseChargeVat() {
return reverseChargeVat;
}
/**
* Setter for the field comment
.
*
* @param comment a {@link java.lang.String} object
*/
public void setComment(String comment) {
this.comment = comment;
}
/**
* Setter for the field customTags
.
*
* @param customTags a {@link java.util.List} object
*/
public void setCustomTags(List customTags) {
this.customTags = customTags;
}
/**
* Setter for the field languageId
.
*
* @param languageId a {@link java.lang.Integer} object
*/
public void setLanguageId(Integer languageId) {
this.languageId = languageId;
}
/**
* Setter for the field user
.
*
* @param user a {@link com.logicommerce.sdk.models.order.OrderUser} object
*/
public void setUser(OrderUser user) {
this.user = user;
}
/**
* Setter for the field totals
.
*
* @param totals a {@link com.logicommerce.sdk.models.order.OrderTotal} object
*/
public void setTotals(OrderTotal totals) {
this.totals = totals;
}
/**
* Setter for the field vouchers
.
*
* @param vouchers a {@link java.util.List} object
*/
public void setVouchers(List vouchers) {
this.vouchers = vouchers;
}
/**
* Setter for the field items
.
*
* @param items a {@link java.util.List} object
*/
public void setItems(List items) {
this.items = items;
}
/**
* Setter for the field paymentSystem
.
*
* @param paymentSystem a {@link com.logicommerce.sdk.models.order.OrderPaymentSystem} object
*/
public void setPaymentSystem(OrderPaymentSystem paymentSystem) {
this.paymentSystem = paymentSystem;
}
/**
* Setter for the field additionalInformation
.
*
* @param additionalInformation a {@link java.util.List} object
*/
public void setAdditionalInformation(List additionalInformation) {
this.additionalInformation = additionalInformation;
}
/**
* Setter for the field currencies
.
*
* @param currencies a {@link java.util.List} object
*/
public void setCurrencies(List currencies) {
this.currencies = currencies;
}
/**
* Setter for the field date
.
*
* @param date a {@link java.time.LocalDateTime} object
*/
public void setDate(LocalDateTime date) {
this.date = date;
}
/**
* Setter for the field deliveryDate
.
*
* @param deliveryDate a {@link java.time.LocalDateTime} object
*/
public void setDeliveryDate(LocalDateTime deliveryDate) {
this.deliveryDate = deliveryDate;
}
/**
* Setter for the field discounts
.
*
* @param discounts a {@link java.util.List} object
*/
public void setDiscounts(List discounts) {
this.discounts = discounts;
}
/**
* Setter for the field documentNumber
.
*
* @param documentNumber a {@link java.lang.String} object
*/
public void setDocumentNumber(String documentNumber) {
this.documentNumber = documentNumber;
}
/**
* Setter for the field exportStatus
.
*
* @param exportStatus a {@link com.logicommerce.sdk.enums.ExportStatusType} object
*/
public void setExportStatus(ExportStatusType exportStatus) {
this.exportStatus = exportStatus;
}
/**
* Setter for the field id
.
*
* @param id a {@link java.lang.Integer} object
*/
public void setId(Integer id) {
this.id = id;
}
/**
* Setter for the field pId
.
*
* @param pId a {@link java.lang.String} object
*/
public void setPId(String pId) {
this.pId = pId;
}
/**
* Setter for the field paymentDate
.
*
* @param paymentDate a {@link java.time.LocalDateTime} object
*/
public void setPaymentDate(LocalDateTime paymentDate) {
this.paymentDate = paymentDate;
}
/**
* Setter for the field delivery
.
*
* @param delivery a {@link com.logicommerce.sdk.models.order.OrderDelivery} object
*/
public void setDelivery(OrderDelivery delivery) {
this.delivery = delivery;
}
/**
* Setter for the field statuses
.
*
* @param statuses a {@link java.util.List} object
*/
public void setStatuses(List statuses) {
this.statuses = statuses;
}
/**
* Setter for the field paid
.
*
* @param paid a boolean
*/
public void setPaid(boolean paid) {
this.paid = paid;
}
/**
* Setter for the field reverseChargeVat
.
*
* @param reverseChargeVat a boolean
*/
public void setReverseChargeVat(boolean reverseChargeVat) {
this.reverseChargeVat = reverseChargeVat;
}
/**
* Setter for the field information
.
*
* @param information a {@link com.logicommerce.sdk.models.order.OrderInformation} object
*/
public void setInformation(OrderInformation information) {
this.information = information;
}
/**
* cleanProperties.
*/
public void cleanProperties() {
this.properties = null;
}
/**
* Setter for the field taxes
.
*
* @param taxes a {@link java.util.List} object
*/
public void setTaxes(List taxes) {
this.taxes = taxes;
}
}