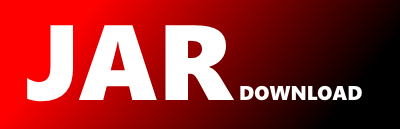
com.logicommerce.sdk.models.order.implementations.OrderDeliveryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
SDK for developing Logicommerce plugins.
package com.logicommerce.sdk.models.order.implementations;
import java.util.List;
import com.logicommerce.sdk.enums.DeliveryType;
import com.logicommerce.sdk.enums.DocumentPickingDeliveryMode;
import com.logicommerce.sdk.models.order.GeographicalZone;
import com.logicommerce.sdk.models.order.OrderDelivery;
import com.logicommerce.sdk.models.order.OrderDeliveryPhysicalLocation;
import com.logicommerce.sdk.models.order.OrderShipment;
import com.logicommerce.sdk.models.order.ProviderPickupPointPickingDocumentDelivery;
import com.logicommerce.utilities.annotations.Uses;
/**
* OrderDeliveryImpl class.
*
* @author Logicommerce
* @since 1.0.16
*/
public class OrderDeliveryImpl implements OrderDelivery {
private DeliveryType type;
@Uses(value = OrderDeliveryPhysicalLocationImpl.class)
private OrderDeliveryPhysicalLocation physicalLocation;
@Uses(value = OrderShipmentImpl.class)
private List shipments;
@Uses(value = GeographicalZoneImpl.class)
private GeographicalZone geographicalZone;
private DocumentPickingDeliveryMode mode;
@Uses(value = ProviderPickupPointPickingDocumentDeliveryImpl.class)
private ProviderPickupPointPickingDocumentDelivery providerPickupPointPickingDocumentDelivery;
/**
* Getter for the field type
.
*
* @return a {@link com.logicommerce.sdk.enums.DeliveryType} object
*/
@Override
public DeliveryType getType() {
return type;
}
/**
* Getter for the field physicalLocation
.
*
* @return a {@link com.logicommerce.sdk.models.order.OrderDeliveryPhysicalLocation} object
*/
@Override
public OrderDeliveryPhysicalLocation getPhysicalLocation() {
return physicalLocation;
}
/**
* Getter for the field shipments
.
*
* @return a {@link java.util.List} object
*/
@Override
public List getShipments() {
return shipments;
}
/**
* Getter for the field geographicalZone
.
*
* @return a {@link com.logicommerce.sdk.models.order.GeographicalZone} object
*/
@Override
public GeographicalZone getGeographicalZone() {
return geographicalZone;
}
/**
* Getter for the field mode
.
* @since 1.3.8
* @return a {@link com.logicommerce.sdk.enums.DocumentPickingDeliveryMode} object
*/
@Override
public DocumentPickingDeliveryMode getMode() {
return mode;
}
@Override
public ProviderPickupPointPickingDocumentDelivery getProviderPickupPointPickingDocumentDelivery() {
return providerPickupPointPickingDocumentDelivery;
}
/**
* Setter for the field type
.
*
* @param type a {@link com.logicommerce.sdk.enums.DeliveryType} object
*/
public void setType(DeliveryType type) {
this.type = type;
}
/**
* Setter for the field physicalLocation
.
*
* @param physicalLocation a {@link com.logicommerce.sdk.models.order.OrderDeliveryPhysicalLocation} object
*/
public void setPhysicalLocation(OrderDeliveryPhysicalLocation physicalLocation) {
this.physicalLocation = physicalLocation;
}
/**
* Setter for the field shipments
.
*
* @param shipments a {@link java.util.List} object
*/
public void setShipments(List shipments) {
this.shipments = shipments;
}
/**
* Setter for the field geographicalZone
.
*
* @param geographicalZone a {@link com.logicommerce.sdk.models.order.GeographicalZone} object
*/
public void setGeographicalZone(GeographicalZone geographicalZone) {
this.geographicalZone = geographicalZone;
}
/**
* Setter for the field mode
.
* @since 1.3.8
* @param mode a {@link com.logicommerce.sdk.enums.DocumentPickingDeliveryMode} object
*/
public void setMode(DocumentPickingDeliveryMode mode) {
this.mode = mode;
}
/**
* Setter for the field providerPickupPointPickingDocumentDelivery
.
*
* @param providerPickupPointPickingDocumentDelivery a
* {@link com.logicommerce.sdk.models.order.ProviderPickupPointPickingDocumentDelivery} object
*/
public void setProviderPickupPointPickingDocumentDelivery(ProviderPickupPointPickingDocumentDelivery
providerPickupPointPickingDocumentDelivery) {
this.providerPickupPointPickingDocumentDelivery = providerPickupPointPickingDocumentDelivery;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy