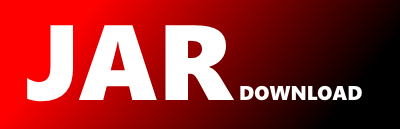
com.logicommerce.sdk.models.implementations.LocationImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
SDK for developing Logicommerce plugins.
package com.logicommerce.sdk.models.implementations;
import com.logicommerce.sdk.models.Location;
/**
* LocationImpl class.
*
* @author Logicommerce
* @since 1.0.16
*/
public class LocationImpl implements Location {
private String country;
private Integer locationId;
private Double latitude;
private Double longitude;
private String stateCode;
private String name;
/**
* Getter for the field country
.
*
* @return a {@link java.lang.String} object
*/
@Override
public String getCountry() {
return country;
}
/**
* Getter for the field locationId
.
*
* @return a {@link java.lang.Integer} object
*/
@Override
public Integer getLocationId() {
return locationId;
}
/**
* Getter for the field latitude
.
*
* @return a {@link java.lang.Double} object
*/
@Override
public Double getLatitude() {
return latitude;
}
/**
* Getter for the field longitude
.
*
* @return a {@link java.lang.Double} object
*/
@Override
public Double getLongitude() {
return longitude;
}
/**
* Getter for the field stateCode
.
*
* @return a {@link java.lang.String} object
*/
@Override
public String getStateCode() {
return stateCode;
}
/**
* Getter for the field country
.
*
* @param country a {@link java.lang.String} object
*/
public void setCountry(String country) {
this.country = country;
}
/**
* Setter for the field locationId
.
*
* @param locationId a {@link java.lang.Integer} object
*/
public void setLocationId(Integer locationId) {
this.locationId = locationId;
}
/**
* Setter for the field latitude
.
*
* @param latitude a {@link java.lang.Double} object
*/
public void setLatitude(Double latitude) {
this.latitude = latitude;
}
/**
* Setter for the field longitude
.
*
* @param longitude a {@link java.lang.Double} object
*/
public void setLongitude(Double longitude) {
this.longitude = longitude;
}
/**
* Setter for the field stateCode
.
*
* @param stateCode a {@link java.lang.String} object
*/
public void setStateCode(String stateCode) {
this.stateCode = stateCode;
}
/**
* Getter for the field name
.
*
* @return a {@link java.lang.String} object
*/
public String getName() {
return name;
}
/**
* Setter for the field name
.
*
* @param name a {@link java.lang.String} object
*/
public void setName(String name) {
this.name = name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy