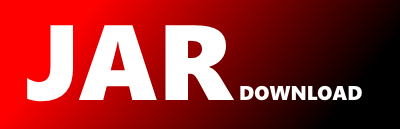
com.logmein.gotocorelib.api.AuthenticationApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of goto-core-lib Show documentation
Show all versions of goto-core-lib Show documentation
Core Library for GoTo Product Java SDKs
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotocorelib.api;
import com.logmein.gotocorelib.api.common.ApiException;
import com.logmein.gotocorelib.api.common.ApiInvoker;
import com.logmein.gotocorelib.api.common.JsonUtil;
import com.logmein.gotocorelib.api.model.LoginResponse;
import com.sun.jersey.multipart.FormDataMultiPart;
import javax.ws.rs.core.MediaType;
import java.util.*;
public class AuthenticationApi {
private String basePath = "https://api.getgo.com/oauth";
private ApiInvoker apiInvoker = ApiInvoker.getInstance();
/**
* Initializes a new instance of the AuthenticationApi class using the
* default endpoint base url for the services being accessed.
*/
public AuthenticationApi() {
}
/**
* Initializes a new instance of the AuthenticationApi class using an endpoint base
* url other than the default. Use this constructor only if you should need
* to override the default endpoint base url.
* @param basePath The endpoint base url for the services being accessed.
*/
public AuthenticationApi(String basePath) {
this.basePath = basePath;
}
public ApiInvoker getInvoker() {
return apiInvoker;
}
public String getBasePath() {
return basePath;
}
/**
* Direct Login
* The standard OAuth flow for authentication is designed to be browser-based, and requires the GoTo product user to login with their email and password, and then approve the access. The Direct Login method can be used as an alternative for cases where the application is non-browser-based or unable to send the GoTo product user through such a flow.
* @param userId The GoTo product user email
* @param password The GoTo product user password
* @param clientId The app's consumer (API) key
* @param grantType This should always be set to 'password'
* @return LoginResponse
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public LoginResponse directLogin(String userId, String password, String clientId, String grantType) throws ApiException {
Object postBody = null;
if(userId == null) {
throw new ApiException("Required parameter userId is null.");
}
if(password == null) {
throw new ApiException("Required parameter password is null.");
}
if(clientId == null) {
throw new ApiException("Required parameter clientId is null.");
}
if(grantType == null) {
throw new ApiException("Required parameter grantType is null.");
}
// create path and map variables
String path = "/access_token"
.replaceAll("\\{format\\}", "json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String[] contentTypes = {
"application/x-www-form-urlencoded"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
hasFields = true;
mp.field("user_id", userId, MediaType.MULTIPART_FORM_DATA_TYPE);
hasFields = true;
mp.field("password", password, MediaType.MULTIPART_FORM_DATA_TYPE);
hasFields = true;
mp.field("client_id", clientId, MediaType.MULTIPART_FORM_DATA_TYPE);
hasFields = true;
mp.field("grant_type", grantType, MediaType.MULTIPART_FORM_DATA_TYPE);
if(hasFields)
postBody = mp;
}
else {
formParams.put("user_id", String.valueOf(userId));
formParams.put("password", String.valueOf(password));
formParams.put("client_id", String.valueOf(clientId));
formParams.put("grant_type", String.valueOf(grantType));
}
String response = apiInvoker.invokeAPI(basePath, path, "POST", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (LoginResponse) ApiInvoker.deserialize(response, "", LoginResponse.class);
}
return null;
}
/**
* Refresh Token
* Generates a new access token without the need for the user to provide credentials again. If the old token has not expired yet, it is revoked. This call can be used for tokens generated after July 1, 2015 (version 2 and above).
* @param refreshToken The refresh token corresponding to the access token we want to refresh
* @param clientId The app's consumer (API) key
* @param grantType This should always be set to 'refresh_token'
* @return LoginResponse
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public LoginResponse refreshToken(String refreshToken, String clientId, String grantType) throws ApiException {
Object postBody = null;
if(refreshToken == null) {
throw new ApiException("Required parameter refreshToken is null.");
}
if(clientId == null) {
throw new ApiException("Required parameter clientId is null.");
}
if(grantType == null) {
throw new ApiException("Required parameter grantType is null.");
}
// create path and map variables
String path = "/access_token"
.replaceAll("\\{format\\}", "json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String refreshTokenString = JsonUtil.Stringify(refreshToken);
if(!"null".equals(refreshTokenString)){
queryParams.put("refresh_token", refreshTokenString);
}
String clientIdString = JsonUtil.Stringify(clientId);
if(!"null".equals(clientIdString)){
queryParams.put("client_id", clientIdString);
}
String grantTypeString = JsonUtil.Stringify(grantType);
if(!"null".equals(grantTypeString)){
queryParams.put("grant_type", grantTypeString);
}
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (LoginResponse) ApiInvoker.deserialize(response, "", LoginResponse.class);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy