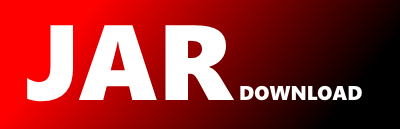
com.logmein.gotocorelib.api.OAuth2Api Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of goto-core-lib Show documentation
Show all versions of goto-core-lib Show documentation
Core Library for GoTo Product Java SDKs
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotocorelib.api;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import org.apache.commons.lang3.StringUtils;
import com.logmein.gotocorelib.api.common.ApiException;
import com.logmein.gotocorelib.api.common.ApiInvoker;
import com.logmein.gotocorelib.api.model.LoginResponse;
public class OAuth2Api {
private String basePath = "https://api.getgo.com/oauth";
private ApiInvoker apiInvoker = ApiInvoker.getInstance();
/**
* Initializes a new instance of the OAuth2Api class using the
* default endpoint base url for the services being accessed.
*/
public OAuth2Api() {
}
/**
* Initializes a new instance of the OAuth2Api class using an endpoint base
* url other than the default. Use this constructor only if you should need
* to override the default endpoint base url.
* @param basePath The endpoint base url for the services being accessed.
*/
public OAuth2Api(String basePath) {
this.basePath = basePath;
}
public ApiInvoker getInvoker() {
return apiInvoker;
}
public String getBasePath() {
return basePath;
}
/**
* Returns the authorization URL where the browser will be directed given the consumer key
* of the app and a redirect Url.
*
* @param clientId The consumer (API) key of the application.
* @param redirectUrl The redirect URL. The application URL associated with the API key
* and the redirect URL domain specified must match. If they do not match the user will be redirected
* to the application URL.
* @return The authorization URL where the browser will be directed.
*/
public String getOAuth2AuthorisationUrl(String clientId, String redirectUrl) {
String authUrl = String.format("/authorize?client_id=%s", clientId);
String redirectPath = String.format("&redirect_uri=%s", redirectUrl);
return String.format("%s%s%s", basePath, authUrl, redirectPath);
}
/**
* Gets the Response Key from the OAuth flow contained in the user redirection back to the application.
* This must be exchanged for an Access Token.
*
* @param navigatedUrl The user redirection.
* @return The response key.
* @throws ApiException If an error occurred while obtaining a responce key.
*/
public String getResponseKey(URL navigatedUrl) throws ApiException {
String query = navigatedUrl.getQuery();
if (StringUtils.isBlank(query))
return null;
String[] pairs = query.split("[ .&,?,/]+");
HashMap parameters = new HashMap();
for (String pair : pairs) {
String[] nameValue = pair.split("=", 2);
parameters.put(nameValue[0], nameValue[1]);
}
if (parameters.containsKey("code"))
return parameters.get("code");
if (parameters.containsKey("error_description"))
throw new ApiException(parameters.get("error_description"));
return null;
}
/**
* Exchanges the response key for an access token.
*
* @param clientId The consumer (API) key of the application.
* @param responseKey The response key.
* @return Information containing the access token and other details about the user's product account.
* @throws ApiException If an error occurred during exchanging the response key for an access token.
*/
public LoginResponse getAccessTokenResponse(String clientId, String responseKey) throws ApiException {
HashMap queryParams = new HashMap();
HashMap headerParams = new HashMap();
headerParams.put("Accepts", "application/json");
HashMap formParams = new HashMap();
formParams.put("grant_type", "authorization_code");
formParams.put("client_id", clientId);
formParams.put("code", responseKey);
String response = apiInvoker.invokeAPI(basePath, "/access_token", "POST",
queryParams, null, headerParams, formParams, "application/x-www-form-urlencoded");
if (response != null)
return (LoginResponse) ApiInvoker.deserialize(response, "", LoginResponse.class);
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy