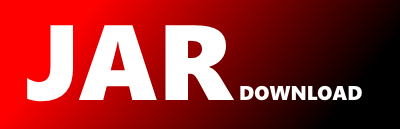
com.logmein.gotomeeting.api.GroupsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotomeeting-api Show documentation
Show all versions of gotomeeting-api Show documentation
Java SDK for the GoToMeeting REST API
The newest version!
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotomeeting.api;
import com.logmein.gotomeeting.api.common.ApiException;
import com.logmein.gotomeeting.api.common.ApiInvoker;
import com.logmein.gotomeeting.api.common.JsonUtil;
import com.logmein.gotomeeting.api.model.OrganizerReq;
import com.logmein.gotomeeting.api.model.OrganizerShort;
import java.util.Date;
import com.logmein.gotomeeting.api.model.AttendeeByGroup;
import com.logmein.gotomeeting.api.model.Group;
import com.logmein.gotomeeting.api.model.HistoricalMeetingByGroup;
import com.logmein.gotomeeting.api.model.HistoryMeetingByGroup;
import com.logmein.gotomeeting.api.model.OrganizerByGroup;
import com.logmein.gotomeeting.api.model.UpcomingMeetingByGroup;
import com.sun.jersey.multipart.FormDataMultiPart;
import javax.ws.rs.core.MediaType;
import java.util.*;
public class GroupsApi {
private String basePath = "https://api.getgo.com/G2M/rest";
private ApiInvoker apiInvoker = ApiInvoker.getInstance();
/**
* Initializes a new instance of the GroupsApi class using the
* default endpoint base url for the services being accessed.
*/
public GroupsApi() {
}
/**
* Initializes a new instance of the GroupsApi class using an endpoint base
* url other than the default. Use this constructor only if you should need
* to override the default endpoint base url.
* @param basePath The endpoint base url for the services being accessed.
*/
public GroupsApi(String basePath) {
this.basePath = basePath;
}
public ApiInvoker getInvoker() {
return apiInvoker;
}
public String getBasePath() {
return basePath;
}
/**
* Create organizer in group
* Creates a new organizer and sends an email to the email address defined in request. This API call is only available to users with the admin role. You may also pass 'G2W' or 'G2T' or 'OPENVOICE' as productType variables, creating organizers for those products. A G2W or G2T organizer will also have access to G2M.
* @param authorization Access token
* @param groupKey The key of the group
* @param body The details of the organizer to be created
* @return List<OrganizerShort>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List createOrganizer(String authorization, Long groupKey, OrganizerReq body) throws ApiException {
Object postBody = body;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(groupKey == null) {
throw new ApiException("Required parameter groupKey is null.");
}
if(body == null) {
throw new ApiException("Required parameter body is null.");
}
// create path and map variables
String path = "/groups/{groupKey}/organizers"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "groupKey" + "\\}", apiInvoker.escapeString(groupKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "POST", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", OrganizerShort.class);
}
return null;
}
/**
* Get attendees by group
* Returns all attendees for all meetings within specified date range held by organizers within the specified group. This API call is only available to users with the admin role. This API call can be used only for groups with maximum 50 organizers.
* @param authorization Access token
* @param groupKey The key of the group
* @param startDate Start of date range, in ISO8601 UTC format, e.g. 2015-07-01T22:00:00Z
* @param endDate End of date range, in ISO8601 UTC format, e.g. 2015-07-01T23:00:00Z
* @return List<AttendeeByGroup>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getAttendeesByGroup(String authorization, Long groupKey, Date startDate, Date endDate) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(groupKey == null) {
throw new ApiException("Required parameter groupKey is null.");
}
// create path and map variables
String path = "/groups/{groupKey}/attendees"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "groupKey" + "\\}", apiInvoker.escapeString(groupKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String startDateString = JsonUtil.Stringify(startDate);
if(!"null".equals(startDateString)){
queryParams.put("startDate", startDateString);
}
String endDateString = JsonUtil.Stringify(endDate);
if(!"null".equals(endDateString)){
queryParams.put("endDate", endDateString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", AttendeeByGroup.class);
}
return null;
}
/**
* Get groups
* List all groups for an account. This API call is only available to users with the admin role.
* @param authorization Access token
* @return List<Group>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getGroups(String authorization) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
// create path and map variables
String path = "/groups"
.replaceAll("\\{format\\}", "json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", Group.class);
}
return null;
}
/**
* Get historical meetings by group
* Get historical meetings for the specified group that started within the specified date/time range. This API call is only available to users with the admin role. This API call is restricted to groups with a maximum of 50 organizers. Remark: Meetings which are still ongoing at the time of the request are NOT contained in the result array.
* @param authorization Access token
* @param groupKey The key of the group
* @param startDate Required start of date range, in ISO8601 UTC format, e.g. 2015-07-01T22:00:00Z
* @param endDate Required end of date range, in ISO8601 UTC format, e.g. 2015-07-01T23:00:00Z
* @return List<HistoricalMeetingByGroup>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getHistoricalMeetingsByGroup(String authorization, Long groupKey, Date startDate, Date endDate) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(groupKey == null) {
throw new ApiException("Required parameter groupKey is null.");
}
if(startDate == null) {
throw new ApiException("Required parameter startDate is null.");
}
if(endDate == null) {
throw new ApiException("Required parameter endDate is null.");
}
// create path and map variables
String path = "/groups/{groupKey}/historicalMeetings"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "groupKey" + "\\}", apiInvoker.escapeString(groupKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String startDateString = JsonUtil.Stringify(startDate);
if(!"null".equals(startDateString)){
queryParams.put("startDate", startDateString);
}
String endDateString = JsonUtil.Stringify(endDate);
if(!"null".equals(endDateString)){
queryParams.put("endDate", endDateString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", HistoricalMeetingByGroup.class);
}
return null;
}
/**
* DEPRECATED: Get historical meetings by group
* DEPRECATED: Please use the new API calls 'Get historical meetings by group' and 'Get upcoming meetings by group'. Get meetings for a specified group. Additional filters can be used to view only meetings within a specified date range. This API call is only available to users with the admin role.
* @param authorization Access token
* @param groupKey The key of the group
* @param history When 'true', returns all past meetings within date range
* @param startDate If history=true, required start of date range, in ISO8601 UTC format, e.g. 2015-07-01T22:00:00Z
* @param endDate If history=true, required end of date range, in ISO8601 UTC format, e.g. 2015-07-01T23:00:00Z
* @return List<HistoryMeetingByGroup>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
@Deprecated
public List getHistoryMeetingsByGroup(String authorization, Long groupKey, Boolean history, Date startDate, Date endDate) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(groupKey == null) {
throw new ApiException("Required parameter groupKey is null.");
}
if(history == null) {
throw new ApiException("Required parameter history is null.");
}
if(startDate == null) {
throw new ApiException("Required parameter startDate is null.");
}
if(endDate == null) {
throw new ApiException("Required parameter endDate is null.");
}
// create path and map variables
String path = "/groups/{groupKey}/meetings"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "groupKey" + "\\}", apiInvoker.escapeString(groupKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String historyString = JsonUtil.Stringify(history);
if(!"null".equals(historyString)){
queryParams.put("history", historyString);
}
String startDateString = JsonUtil.Stringify(startDate);
if(!"null".equals(startDateString)){
queryParams.put("startDate", startDateString);
}
String endDateString = JsonUtil.Stringify(endDate);
if(!"null".equals(endDateString)){
queryParams.put("endDate", endDateString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", HistoryMeetingByGroup.class);
}
return null;
}
/**
* Get organizers by group
* Returns all the organizers within a specific group. This API call is only available to users with the admin role.
* @param authorization Access token
* @param groupKey The key of the group
* @return List<OrganizerByGroup>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getOrganizersByGroup(String authorization, Long groupKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(groupKey == null) {
throw new ApiException("Required parameter groupKey is null.");
}
// create path and map variables
String path = "/groups/{groupKey}/organizers"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "groupKey" + "\\}", apiInvoker.escapeString(groupKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", OrganizerByGroup.class);
}
return null;
}
/**
* Get upcoming meetings by group
* Get upcoming meetings for a specified group. This API call is only available to users with the admin role. This API call can be used only for groups with maximum 50 organizers.
* @param authorization Access token
* @param groupKey The key of the group
* @return List<UpcomingMeetingByGroup>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getUpcomingMeetingsByGroup(String authorization, Long groupKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(groupKey == null) {
throw new ApiException("Required parameter groupKey is null.");
}
// create path and map variables
String path = "/groups/{groupKey}/upcomingMeetings"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "groupKey" + "\\}", apiInvoker.escapeString(groupKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", UpcomingMeetingByGroup.class);
}
return null;
}
/**
* Get scheduled meetings by group
* Get scheduled meetings for a group. This API is only available to corporate users with the admin role.
* @deprecated Please use getUpcomingMeetingsByGroup() instead
* @param authorization Access token
* @param groupKey The key of the group
* @param scheduled When 'true' returns all future meetings
* @param startDate If history=true, required start of date range, in ISO8601 UTC format
* @param endDate If history=true, required end of date range, in ISO8601 UTC format
* @return List<ScheduledMeetingByGroup>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
//FIME what is that? hardcoding!!!
@Deprecated
public List getScheduledMeetingsByGroup (String authorization, Long groupKey, Boolean scheduled, Date startDate, Date endDate) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(groupKey == null) {
throw new ApiException("Required parameter groupKey is null.");
}
if(scheduled == null) {
throw new ApiException("Required parameter scheduled is null.");
}
if(startDate == null) {
throw new ApiException("Required parameter startDate is null.");
}
if(endDate == null) {
throw new ApiException("Required parameter endDate is null.");
}
// create path and map variables
String path = "/groups/{groupKey}/meetings"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "groupKey" + "\\}", apiInvoker.escapeString(groupKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String scheduledString = JsonUtil.Stringify(scheduled);
if(!"null".equals(scheduledString)){
queryParams.put("scheduled", scheduledString);
}
String startDateString = JsonUtil.Stringify(startDate);
if(!"null".equals(startDateString)){
queryParams.put("startDate", startDateString);
}
String endDateString = JsonUtil.Stringify(endDate);
if(!"null".equals(endDateString)){
queryParams.put("endDate", endDateString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null) {
return (List)ApiInvoker.deserialize(response, "List", com.logmein.gotomeeting.api.model.ScheduledMeetingByGroup.class);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy