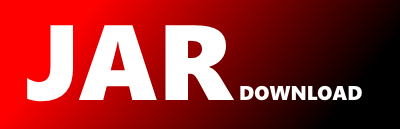
com.logmein.gotomeeting.api.MeetingsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotomeeting-api Show documentation
Show all versions of gotomeeting-api Show documentation
Java SDK for the GoToMeeting REST API
The newest version!
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotomeeting.api;
import com.logmein.gotomeeting.api.common.ApiException;
import com.logmein.gotomeeting.api.common.ApiInvoker;
import com.logmein.gotomeeting.api.common.JsonUtil;
import com.logmein.gotomeeting.api.model.MeetingCreated;
import com.logmein.gotomeeting.api.model.MeetingReqCreate;
import com.logmein.gotomeeting.api.model.AttendeeByMeeting;
import java.util.Date;
import com.logmein.gotomeeting.api.model.HistoricalMeeting;
import com.logmein.gotomeeting.api.model.MeetingHistory;
import com.logmein.gotomeeting.api.model.MeetingById;
import com.logmein.gotomeeting.api.model.UpcomingMeeting;
import com.logmein.gotomeeting.api.model.StartUrl;
import com.logmein.gotomeeting.api.model.MeetingReqUpdate;
import com.sun.jersey.multipart.FormDataMultiPart;
import javax.ws.rs.core.MediaType;
import java.util.*;
public class MeetingsApi {
private String basePath = "https://api.getgo.com/G2M/rest";
private ApiInvoker apiInvoker = ApiInvoker.getInstance();
/**
* Initializes a new instance of the MeetingsApi class using the
* default endpoint base url for the services being accessed.
*/
public MeetingsApi() {
}
/**
* Initializes a new instance of the MeetingsApi class using an endpoint base
* url other than the default. Use this constructor only if you should need
* to override the default endpoint base url.
* @param basePath The endpoint base url for the services being accessed.
*/
public MeetingsApi(String basePath) {
this.basePath = basePath;
}
public ApiInvoker getInvoker() {
return apiInvoker;
}
public String getBasePath() {
return basePath;
}
/**
* Create meeting
* Create a new meeting based on the parameters specified.
* @param authorization Access token
* @param body The meeting details
* @return List<MeetingCreated>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List createMeeting(String authorization, MeetingReqCreate body) throws ApiException {
Object postBody = body;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(body == null) {
throw new ApiException("Required parameter body is null.");
}
// create path and map variables
String path = "/meetings"
.replaceAll("\\{format\\}", "json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "POST", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", MeetingCreated.class);
}
return null;
}
/**
* Delete meeting
* Deletes the meeting identified by the meetingId.
* @param authorization Access token
* @param meetingId The meeting ID
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public void deleteMeeting(String authorization, Long meetingId) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(meetingId == null) {
throw new ApiException("Required parameter meetingId is null.");
}
// create path and map variables
String path = "/meetings/{meetingId}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "meetingId" + "\\}", apiInvoker.escapeString(meetingId.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
apiInvoker.invokeAPI(basePath, path, "DELETE", queryParams, postBody, headerParams, formParams, contentType);
}
/**
* Get attendees by meeting
* List all attendees for specified meetingId of historical meeting. The historical meetings can be fetched using 'Get historical meetings', 'Get historical meetings by organizer', and 'Get historical meetings by group'. For users with the admin role this call returns attendees for any meeting. For any other user the call will return attendees for meetings on which the user is a valid organizer.
* @param authorization Access token
* @param meetingId The meeting ID
* @return List<AttendeeByMeeting>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getAttendeesByMeetings(String authorization, Long meetingId) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(meetingId == null) {
throw new ApiException("Required parameter meetingId is null.");
}
// create path and map variables
String path = "/meetings/{meetingId}/attendees"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "meetingId" + "\\}", apiInvoker.escapeString(meetingId.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", AttendeeByMeeting.class);
}
return null;
}
/**
* Get historical meetings
* Get historical meetings for the currently authenticated organizer that started within the specified date/time range. Remark: Meetings which are still ongoing at the time of the request are NOT contained in the result array.
* @param authorization Access token
* @param startDate Required start of date range, in ISO8601 UTC format, e.g. 2015-07-01T22:00:00Z
* @param endDate Required end of date range, in ISO8601 UTC format, e.g. 2015-07-01T23:00:00Z
* @return List<HistoricalMeeting>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getHistoricalMeetings(String authorization, Date startDate, Date endDate) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(startDate == null) {
throw new ApiException("Required parameter startDate is null.");
}
if(endDate == null) {
throw new ApiException("Required parameter endDate is null.");
}
// create path and map variables
String path = "/historicalMeetings"
.replaceAll("\\{format\\}", "json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String startDateString = JsonUtil.Stringify(startDate);
if(!"null".equals(startDateString)){
queryParams.put("startDate", startDateString);
}
String endDateString = JsonUtil.Stringify(endDate);
if(!"null".equals(endDateString)){
queryParams.put("endDate", endDateString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", HistoricalMeeting.class);
}
return null;
}
/**
* DEPRECATED: Get historical meetings
* DEPRECATED: Please use the new API calls 'Get historical meetings' and 'Get upcoming meetings'. Gets historical meetings for the current authenticated organizer. Requires date range for filtering results to only meetings within specified dates. History searches will contain the parameter 'meetingInstanceKey' which is used in conjunction with the call 'Get Attendees by Meeting' to get attendee information for a past meeting.
* @param authorization Access token
* @param history When 'true', returns all past meetings within date range
* @param startDate If history=true, required start of date range, in ISO8601 UTC format, e.g. 2015-07-01T22:00:00Z
* @param endDate If history=true, required end of date range, in ISO8601 UTC format, e.g. 2015-07-01T23:00:00Z
* @return List<MeetingHistory>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
@Deprecated
public List getHistoryMeetings(String authorization, Boolean history, Date startDate, Date endDate) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
// create path and map variables
String path = "/meetings"
.replaceAll("\\{format\\}", "json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String historyString = JsonUtil.Stringify(history);
if(!"null".equals(historyString)){
queryParams.put("history", historyString);
}
String startDateString = JsonUtil.Stringify(startDate);
if(!"null".equals(startDateString)){
queryParams.put("startDate", startDateString);
}
String endDateString = JsonUtil.Stringify(endDate);
if(!"null".equals(endDateString)){
queryParams.put("endDate", endDateString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", MeetingHistory.class);
}
return null;
}
/**
* Get meeting
* Returns the meeting details for the specified meeting.
* @param authorization Access token
* @param meetingId The meeting ID
* @return List<MeetingById>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getMeeting(String authorization, Long meetingId) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(meetingId == null) {
throw new ApiException("Required parameter meetingId is null.");
}
// create path and map variables
String path = "/meetings/{meetingId}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "meetingId" + "\\}", apiInvoker.escapeString(meetingId.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", MeetingById.class);
}
return null;
}
/**
* Get upcoming meetings
* Gets upcoming meetings for the current authenticated organizer.
* @param authorization Access token
* @return List<UpcomingMeeting>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getUpcomingMeetings(String authorization) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
// create path and map variables
String path = "/upcomingMeetings"
.replaceAll("\\{format\\}", "json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", UpcomingMeeting.class);
}
return null;
}
/**
* Start meeting
* Returns a host URL that can be used to start a meeting. When this URL is opened in a web browser, the GoToMeeting client will be downloaded and launched and the meeting will start. The end user is not required to login to a client.
* @param authorization Access token
* @param meetingId The meeting ID
* @return StartUrl
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public StartUrl startMeeting(String authorization, Long meetingId) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(meetingId == null) {
throw new ApiException("Required parameter meetingId is null.");
}
// create path and map variables
String path = "/meetings/{meetingId}/start"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "meetingId" + "\\}", apiInvoker.escapeString(meetingId.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (StartUrl) ApiInvoker.deserialize(response, "", StartUrl.class);
}
return null;
}
/**
* Update meeting
* Updates an existing meeting specified by meetingId.
* @param authorization Access token
* @param meetingId The meeting ID
* @param body The meeting details
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public void updateMeeting(String authorization, Long meetingId, MeetingReqUpdate body) throws ApiException {
Object postBody = body;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(meetingId == null) {
throw new ApiException("Required parameter meetingId is null.");
}
if(body == null) {
throw new ApiException("Required parameter body is null.");
}
// create path and map variables
String path = "/meetings/{meetingId}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "meetingId" + "\\}", apiInvoker.escapeString(meetingId.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
apiInvoker.invokeAPI(basePath, path, "PUT", queryParams, postBody, headerParams, formParams, contentType);
}
/**
* Get scheduled meetings
* Gets scheduled meetings (meetings that have not occurred) for a specified organizer.
* @deprecated Use getUpcomingMeetings() instead.
* @param authorization Access token
* @param scheduled When "true" returns all future meetings
* @return List<MeetingScheduled>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
@Deprecated
public List getScheduledMeetings (String authorization, Boolean scheduled) throws ApiException {
Object postBody = null;
if(authorization == null ) {
throw new ApiException("Required parameter authorization is null.");
}
// create path and map variables
String path = "/meetings".replaceAll("\\{format\\}","json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String scheduledString = JsonUtil.Stringify(scheduled);
if(!"null".equals(scheduledString)){
queryParams.put("scheduled", scheduledString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List)ApiInvoker.deserialize(response, "List", com.logmein.gotomeeting.api.model.MeetingScheduled.class);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy