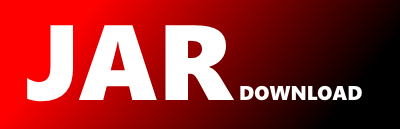
com.logmein.gotomeeting.api.model.Group Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotomeeting-api Show documentation
Show all versions of gotomeeting-api Show documentation
Java SDK for the GoToMeeting REST API
The newest version!
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotomeeting.api.model;
import com.logmein.gotomeeting.api.model.Status;
import com.logmein.gotomeeting.api.common.JsonUtil;
/**
* Describes a collection of organizers categorized by department or job function, for example. Can be a subgroup of a larger group.
*/
public class Group {
/* The key of this group */
private Long groupkey = null;
/* The name of this group */
private String groupName = null;
/* The key of the parent group this group belongs to. Identical with the groupkey if this group is not a subgroup */
private Long parentKey = null;
/* The status of this group, i.e. whether the organizers assigned to it are able to host meetings */
private Status status = null;
/* The number of organizers assigned to this group */
private Integer numOrganizers = null;
/**
* @return The key of this group
*/
public Long getGroupkey() {
return groupkey;
}
/**
* @param groupkey The key of this group
*/
public void setGroupkey(Long groupkey) {
this.groupkey = groupkey;
}
/**
* @return The name of this group
*/
public String getGroupName() {
return groupName;
}
/**
* @param groupName The name of this group
*/
public void setGroupName(String groupName) {
this.groupName = groupName;
}
/**
* @return The key of the parent group this group belongs to. Identical with the groupkey if this group is not a subgroup
*/
public Long getParentKey() {
return parentKey;
}
/**
* @param parentKey The key of the parent group this group belongs to. Identical with the groupkey if this group is not a subgroup
*/
public void setParentKey(Long parentKey) {
this.parentKey = parentKey;
}
/**
* @return The status of this group, i.e. whether the organizers assigned to it are able to host meetings
*/
public Status getStatus() {
return status;
}
/**
* @param status The status of this group, i.e. whether the organizers assigned to it are able to host meetings
*/
public void setStatus(Status status) {
this.status = status;
}
/**
* @return The number of organizers assigned to this group
*/
public Integer getNumOrganizers() {
return numOrganizers;
}
/**
* @param numOrganizers The number of organizers assigned to this group
*/
public void setNumOrganizers(Integer numOrganizers) {
this.numOrganizers = numOrganizers;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Group {\n");
String groupkeyString = JsonUtil.Stringify(groupkey);
if (groupkeyString != null && !groupkeyString.isEmpty())
sb.append(String.format(" groupkey: %s\n", groupkeyString));
String groupNameString = JsonUtil.Stringify(groupName);
if (groupNameString != null && !groupNameString.isEmpty())
sb.append(String.format(" groupName: %s\n", groupNameString));
String parentKeyString = JsonUtil.Stringify(parentKey);
if (parentKeyString != null && !parentKeyString.isEmpty())
sb.append(String.format(" parentKey: %s\n", parentKeyString));
String statusString = JsonUtil.Stringify(status);
if (statusString != null && !statusString.isEmpty())
sb.append(String.format(" status: %s\n", statusString));
String numOrganizersString = JsonUtil.Stringify(numOrganizers);
if (numOrganizersString != null && !numOrganizersString.isEmpty())
sb.append(String.format(" numOrganizers: %s\n", numOrganizersString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy