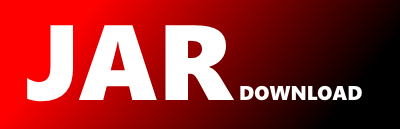
com.logmein.gotomeeting.api.model.HistoricalMeetingByGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotomeeting-api Show documentation
Show all versions of gotomeeting-api Show documentation
Java SDK for the GoToMeeting REST API
The newest version!
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotomeeting.api.model;
import com.logmein.gotomeeting.api.model.MeetingType;
import java.util.Date;
import com.logmein.gotomeeting.api.common.JsonUtil;
/**
* Describes a historical meeting within specified dates for a specified group.
*/
public class HistoricalMeetingByGroup {
/* The time the meeting instance started */
private Date startTime = null;
/* The surname of the meeting organizer */
private String lastName = null;
/* The duration of the meeting session in minutes */
private String duration = null;
/* The number of attendees at the meeting instance */
private String numAttendees = null;
/* The key of the company account */
private String accountKey = null;
/* The meeting organizer's email address */
private String email = null;
/* The subject of the meeting */
private String subject = null;
/* The current language setting of the organizer in the web portal */
private String locale = null;
/* The key of the meeting organizer */
private String organizerKey = null;
/* The meeting ID */
private String meetingId = null;
/* The meeting type */
private MeetingType meetingType = null;
/* The meeting organizer's first name */
private String firstName = null;
/* The time the meeting instance ended */
private Date endTime = null;
/* The name of the group */
private String groupName = null;
/**
* @return The time the meeting instance started
*/
public Date getStartTime() {
return startTime;
}
/**
* @param startTime The time the meeting instance started
*/
public void setStartTime(Date startTime) {
this.startTime = startTime;
}
/**
* @return The surname of the meeting organizer
*/
public String getLastName() {
return lastName;
}
/**
* @param lastName The surname of the meeting organizer
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
* @return The duration of the meeting session in minutes
*/
public String getDuration() {
return duration;
}
/**
* @param duration The duration of the meeting session in minutes
*/
public void setDuration(String duration) {
this.duration = duration;
}
/**
* @return The number of attendees at the meeting instance
*/
public String getNumAttendees() {
return numAttendees;
}
/**
* @param numAttendees The number of attendees at the meeting instance
*/
public void setNumAttendees(String numAttendees) {
this.numAttendees = numAttendees;
}
/**
* @return The key of the company account
*/
public String getAccountKey() {
return accountKey;
}
/**
* @param accountKey The key of the company account
*/
public void setAccountKey(String accountKey) {
this.accountKey = accountKey;
}
/**
* @return The meeting organizer's email address
*/
public String getEmail() {
return email;
}
/**
* @param email The meeting organizer's email address
*/
public void setEmail(String email) {
this.email = email;
}
/**
* @return The subject of the meeting
*/
public String getSubject() {
return subject;
}
/**
* @param subject The subject of the meeting
*/
public void setSubject(String subject) {
this.subject = subject;
}
/**
* @return The current language setting of the organizer in the web portal
*/
public String getLocale() {
return locale;
}
/**
* @param locale The current language setting of the organizer in the web portal
*/
public void setLocale(String locale) {
this.locale = locale;
}
/**
* @return The key of the meeting organizer
*/
public String getOrganizerKey() {
return organizerKey;
}
/**
* @param organizerKey The key of the meeting organizer
*/
public void setOrganizerKey(String organizerKey) {
this.organizerKey = organizerKey;
}
/**
* @return The meeting ID
*/
public String getMeetingId() {
return meetingId;
}
/**
* @param meetingId The meeting ID
*/
public void setMeetingId(String meetingId) {
this.meetingId = meetingId;
}
/**
* @return The meeting type
*/
public MeetingType getMeetingType() {
return meetingType;
}
/**
* @param meetingType The meeting type
*/
public void setMeetingType(MeetingType meetingType) {
this.meetingType = meetingType;
}
/**
* @return The meeting organizer's first name
*/
public String getFirstName() {
return firstName;
}
/**
* @param firstName The meeting organizer's first name
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
* @return The time the meeting instance ended
*/
public Date getEndTime() {
return endTime;
}
/**
* @param endTime The time the meeting instance ended
*/
public void setEndTime(Date endTime) {
this.endTime = endTime;
}
/**
* @return The name of the group
*/
public String getGroupName() {
return groupName;
}
/**
* @param groupName The name of the group
*/
public void setGroupName(String groupName) {
this.groupName = groupName;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HistoricalMeetingByGroup {\n");
String startTimeString = JsonUtil.Stringify(startTime);
if (startTimeString != null && !startTimeString.isEmpty())
sb.append(String.format(" startTime: %s\n", startTimeString));
String lastNameString = JsonUtil.Stringify(lastName);
if (lastNameString != null && !lastNameString.isEmpty())
sb.append(String.format(" lastName: %s\n", lastNameString));
String durationString = JsonUtil.Stringify(duration);
if (durationString != null && !durationString.isEmpty())
sb.append(String.format(" duration: %s\n", durationString));
String numAttendeesString = JsonUtil.Stringify(numAttendees);
if (numAttendeesString != null && !numAttendeesString.isEmpty())
sb.append(String.format(" numAttendees: %s\n", numAttendeesString));
String accountKeyString = JsonUtil.Stringify(accountKey);
if (accountKeyString != null && !accountKeyString.isEmpty())
sb.append(String.format(" accountKey: %s\n", accountKeyString));
String emailString = JsonUtil.Stringify(email);
if (emailString != null && !emailString.isEmpty())
sb.append(String.format(" email: %s\n", emailString));
String subjectString = JsonUtil.Stringify(subject);
if (subjectString != null && !subjectString.isEmpty())
sb.append(String.format(" subject: %s\n", subjectString));
String localeString = JsonUtil.Stringify(locale);
if (localeString != null && !localeString.isEmpty())
sb.append(String.format(" locale: %s\n", localeString));
String organizerKeyString = JsonUtil.Stringify(organizerKey);
if (organizerKeyString != null && !organizerKeyString.isEmpty())
sb.append(String.format(" organizerKey: %s\n", organizerKeyString));
String meetingIdString = JsonUtil.Stringify(meetingId);
if (meetingIdString != null && !meetingIdString.isEmpty())
sb.append(String.format(" meetingId: %s\n", meetingIdString));
String meetingTypeString = JsonUtil.Stringify(meetingType);
if (meetingTypeString != null && !meetingTypeString.isEmpty())
sb.append(String.format(" meetingType: %s\n", meetingTypeString));
String firstNameString = JsonUtil.Stringify(firstName);
if (firstNameString != null && !firstNameString.isEmpty())
sb.append(String.format(" firstName: %s\n", firstNameString));
String endTimeString = JsonUtil.Stringify(endTime);
if (endTimeString != null && !endTimeString.isEmpty())
sb.append(String.format(" endTime: %s\n", endTimeString));
String groupNameString = JsonUtil.Stringify(groupName);
if (groupNameString != null && !groupNameString.isEmpty())
sb.append(String.format(" groupName: %s\n", groupNameString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy