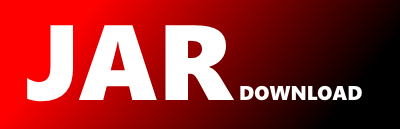
com.logmein.gotomeeting.api.model.MeetingCreated Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotomeeting-api Show documentation
Show all versions of gotomeeting-api Show documentation
Java SDK for the GoToMeeting REST API
The newest version!
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotomeeting.api.model;
import com.logmein.gotomeeting.api.common.JsonUtil;
/**
* Describes a newly created meeting.
*/
public class MeetingCreated {
/* The URL the meeting participants will use to join the meeting */
private String joinURL = null;
/* The meeting ID */
private Long meetingid = null;
/* The maximum number of participants allowed in the meeting */
private Integer maxParticipants = null;
/* The meeting ID. Field retained for backwards compatibility reasons */
private Long uniqueMeetingId = null;
/* Audio options for the meeting */
private String conferenceCallInfo = null;
/**
* @return The URL the meeting participants will use to join the meeting
*/
public String getJoinURL() {
return joinURL;
}
/**
* @param joinURL The URL the meeting participants will use to join the meeting
*/
public void setJoinURL(String joinURL) {
this.joinURL = joinURL;
}
/**
* @return The meeting ID
*/
public Long getMeetingid() {
return meetingid;
}
/**
* @param meetingid The meeting ID
*/
public void setMeetingid(Long meetingid) {
this.meetingid = meetingid;
}
/**
* @return The maximum number of participants allowed in the meeting
*/
public Integer getMaxParticipants() {
return maxParticipants;
}
/**
* @param maxParticipants The maximum number of participants allowed in the meeting
*/
public void setMaxParticipants(Integer maxParticipants) {
this.maxParticipants = maxParticipants;
}
/**
* @return The meeting ID. Field retained for backwards compatibility reasons
*/
public Long getUniqueMeetingId() {
return uniqueMeetingId;
}
/**
* @param uniqueMeetingId The meeting ID. Field retained for backwards compatibility reasons
*/
public void setUniqueMeetingId(Long uniqueMeetingId) {
this.uniqueMeetingId = uniqueMeetingId;
}
/**
* @return Audio options for the meeting
*/
public String getConferenceCallInfo() {
return conferenceCallInfo;
}
/**
* @param conferenceCallInfo Audio options for the meeting
*/
public void setConferenceCallInfo(String conferenceCallInfo) {
this.conferenceCallInfo = conferenceCallInfo;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MeetingCreated {\n");
String joinURLString = JsonUtil.Stringify(joinURL);
if (joinURLString != null && !joinURLString.isEmpty())
sb.append(String.format(" joinURL: %s\n", joinURLString));
String meetingidString = JsonUtil.Stringify(meetingid);
if (meetingidString != null && !meetingidString.isEmpty())
sb.append(String.format(" meetingid: %s\n", meetingidString));
String maxParticipantsString = JsonUtil.Stringify(maxParticipants);
if (maxParticipantsString != null && !maxParticipantsString.isEmpty())
sb.append(String.format(" maxParticipants: %s\n", maxParticipantsString));
String uniqueMeetingIdString = JsonUtil.Stringify(uniqueMeetingId);
if (uniqueMeetingIdString != null && !uniqueMeetingIdString.isEmpty())
sb.append(String.format(" uniqueMeetingId: %s\n", uniqueMeetingIdString));
String conferenceCallInfoString = JsonUtil.Stringify(conferenceCallInfo);
if (conferenceCallInfoString != null && !conferenceCallInfoString.isEmpty())
sb.append(String.format(" conferenceCallInfo: %s\n", conferenceCallInfoString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy