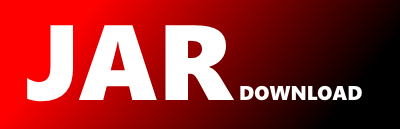
com.logmein.gotomeeting.api.model.MeetingReqUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotomeeting-api Show documentation
Show all versions of gotomeeting-api Show documentation
Java SDK for the GoToMeeting REST API
The newest version!
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotomeeting.api.model;
import com.logmein.gotomeeting.api.model.MeetingType;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import com.logmein.gotomeeting.api.common.JsonUtil;
/**
* Describes the information required to update a meeting.
*/
public class MeetingReqUpdate {
/* A description of the meeting. 100 characters maximum. The characters '>' and '<' have to be replaced with the corresponding html character code (> for '>' and < for '<') */
private String subject = null;
/* The starting time of the meeting. A required ISO8601 UTC string, e.g. 2015-07-01T22:00:00Z */
private Date starttime = null;
/* The ending time of the meeting. A required ISO8601 UTC string, e.g. 2015-07-01T22:00:00Z */
private Date endtime = null;
/* Indicates whether a password is required to join the meeting. Required parameter */
private Boolean passwordrequired = null;
/* A required string. Can be one of the following options:
PSTN (PSTN only),
Free (PSTN and VoIP),
Hybrid, (PSTN and VoIP),
Private (you provide numbers and access code), or
VoIP (VoIP only).
You may also enter plain text for numbers and access codes with a limit of 255 characters */
private String conferencecallinfo = null;
/* DEPRECATED. Must be provided and set to empty string '' */
@Deprecated
private String timezonekey = null;
/* The meeting type */
private MeetingType meetingtype = null;
/* Co-organizer keys. Co-organizers can start the meeting on the organizers behalf. Retrieve a list of valid organizers from the \"Get all organizers\" call. */
private List coorganizerKeys = new ArrayList();
/**
* @return A description of the meeting. 100 characters maximum. The characters '>' and '<' have to be replaced with the corresponding html character code (> for '>' and < for '<')
*/
public String getSubject() {
return subject;
}
/**
* @param subject A description of the meeting. 100 characters maximum. The characters '>' and '<' have to be replaced with the corresponding html character code (> for '>' and < for '<')
*/
public void setSubject(String subject) {
this.subject = subject;
}
/**
* @return The starting time of the meeting. A required ISO8601 UTC string, e.g. 2015-07-01T22:00:00Z
*/
public Date getStarttime() {
return starttime;
}
/**
* @param starttime The starting time of the meeting. A required ISO8601 UTC string, e.g. 2015-07-01T22:00:00Z
*/
public void setStarttime(Date starttime) {
this.starttime = starttime;
}
/**
* @return The ending time of the meeting. A required ISO8601 UTC string, e.g. 2015-07-01T22:00:00Z
*/
public Date getEndtime() {
return endtime;
}
/**
* @param endtime The ending time of the meeting. A required ISO8601 UTC string, e.g. 2015-07-01T22:00:00Z
*/
public void setEndtime(Date endtime) {
this.endtime = endtime;
}
/**
* @return Indicates whether a password is required to join the meeting. Required parameter
*/
public Boolean getPasswordrequired() {
return passwordrequired;
}
/**
* @param passwordrequired Indicates whether a password is required to join the meeting. Required parameter
*/
public void setPasswordrequired(Boolean passwordrequired) {
this.passwordrequired = passwordrequired;
}
/**
* @return A required string. Can be one of the following options:
PSTN (PSTN only),
Free (PSTN and VoIP),
Hybrid, (PSTN and VoIP),
Private (you provide numbers and access code), or
VoIP (VoIP only).
You may also enter plain text for numbers and access codes with a limit of 255 characters
*/
public String getConferencecallinfo() {
return conferencecallinfo;
}
/**
* @param conferencecallinfo A required string. Can be one of the following options:
PSTN (PSTN only),
Free (PSTN and VoIP),
Hybrid, (PSTN and VoIP),
Private (you provide numbers and access code), or
VoIP (VoIP only).
You may also enter plain text for numbers and access codes with a limit of 255 characters
*/
public void setConferencecallinfo(String conferencecallinfo) {
this.conferencecallinfo = conferencecallinfo;
}
/**
* @return DEPRECATED. Must be provided and set to empty string ''
*/
@Deprecated
public String getTimezonekey() {
return timezonekey;
}
/**
* @param timezonekey DEPRECATED. Must be provided and set to empty string ''
*/
@Deprecated
public void setTimezonekey(String timezonekey) {
this.timezonekey = timezonekey;
}
/**
* @return The meeting type
*/
public MeetingType getMeetingtype() {
return meetingtype;
}
/**
* @param meetingtype The meeting type
*/
public void setMeetingtype(MeetingType meetingtype) {
this.meetingtype = meetingtype;
}
/**
* @return Co-organizer keys. Co-organizers can start the meeting on the organizers behalf. Retrieve a list of valid organizers from the \"Get all organizers\" call.
*/
public List getCoorganizerKeys() {
return coorganizerKeys;
}
/**
* @param coorganizerKeys Co-organizer keys. Co-organizers can start the meeting on the organizers behalf. Retrieve a list of valid organizers from the \"Get all organizers\" call.
*/
public void setCoorganizerKeys(List coorganizerKeys) {
this.coorganizerKeys = coorganizerKeys;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MeetingReqUpdate {\n");
String subjectString = JsonUtil.Stringify(subject);
if (subjectString != null && !subjectString.isEmpty())
sb.append(String.format(" subject: %s\n", subjectString));
String starttimeString = JsonUtil.Stringify(starttime);
if (starttimeString != null && !starttimeString.isEmpty())
sb.append(String.format(" starttime: %s\n", starttimeString));
String endtimeString = JsonUtil.Stringify(endtime);
if (endtimeString != null && !endtimeString.isEmpty())
sb.append(String.format(" endtime: %s\n", endtimeString));
String passwordrequiredString = JsonUtil.Stringify(passwordrequired);
if (passwordrequiredString != null && !passwordrequiredString.isEmpty())
sb.append(String.format(" passwordrequired: %s\n", passwordrequiredString));
String conferencecallinfoString = JsonUtil.Stringify(conferencecallinfo);
if (conferencecallinfoString != null && !conferencecallinfoString.isEmpty())
sb.append(String.format(" conferencecallinfo: %s\n", conferencecallinfoString));
String timezonekeyString = JsonUtil.Stringify(timezonekey);
if (timezonekeyString != null && !timezonekeyString.isEmpty())
sb.append(String.format(" timezonekey: %s\n", timezonekeyString));
String meetingtypeString = JsonUtil.Stringify(meetingtype);
if (meetingtypeString != null && !meetingtypeString.isEmpty())
sb.append(String.format(" meetingtype: %s\n", meetingtypeString));
String coorganizerKeysString = JsonUtil.Stringify(coorganizerKeys);
if (coorganizerKeysString != null && !coorganizerKeysString.isEmpty())
sb.append(String.format(" coorganizerKeys: %s\n", coorganizerKeysString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy