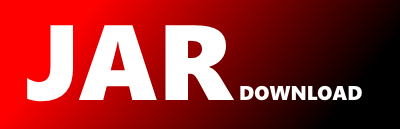
com.logmein.gotowebinar_2_0.api.AttendeesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api;
import com.logmein.gotowebinar_2_0.api.common.ApiException;
import com.logmein.gotowebinar_2_0.api.common.ApiInvoker;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
import com.logmein.gotowebinar_2_0.api.model.Registrant;
import com.logmein.gotowebinar_2_0.api.model.PollAnswer;
import com.logmein.gotowebinar_2_0.api.model.AttendeeQuestion;
import com.logmein.gotowebinar_2_0.api.model.Attendee;
import com.sun.jersey.multipart.FormDataMultiPart;
import javax.ws.rs.core.MediaType;
import java.util.*;
public class AttendeesApi {
private String basePath = "https://api.getgo.com/G2W/rest/v2";
private ApiInvoker apiInvoker = ApiInvoker.getInstance();
/**
* Initializes a new instance of the AttendeesApi class using the
* default endpoint base url for the services being accessed.
*/
public AttendeesApi() {
}
/**
* Initializes a new instance of the AttendeesApi class using an endpoint base
* url other than the default. Use this constructor only if you should need
* to override the default endpoint base url.
* @param basePath The endpoint base url for the services being accessed.
*/
public AttendeesApi(String basePath) {
this.basePath = basePath;
}
public ApiInvoker getInvoker() {
return apiInvoker;
}
public String getBasePath() {
return basePath;
}
/**
* Get attendee
* Retrieve registration details for a particular attendee of a specific webinar session. For technical reasons, this call cannot be executed from this documentation. Please use the curl command to execute it.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param sessionKey The key of the webinar session
* @param registrantKey The key of the registrant
* @return Registrant
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public Registrant getAttendee(String authorization, Long organizerKey, Long webinarKey, Long sessionKey, Long registrantKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(sessionKey == null) {
throw new ApiException("Required parameter sessionKey is null.");
}
if(registrantKey == null) {
throw new ApiException("Required parameter registrantKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/sessions/{sessionKey}/attendees/{registrantKey}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()))
.replaceAll("\\{" + "sessionKey" + "\\}", apiInvoker.escapeString(sessionKey.toString()))
.replaceAll("\\{" + "registrantKey" + "\\}", apiInvoker.escapeString(registrantKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (Registrant) ApiInvoker.deserialize(response, "", Registrant.class);
}
return null;
}
/**
* Get attendee poll answers
* Get poll answers from a particular attendee of a specific webinar session. For technical reasons, this call cannot be executed from this documentation. Please use the curl command to execute it.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param sessionKey The key of the webinar session
* @param registrantKey The key of the registrant
* @return List<PollAnswer>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getAttendeePollAnswers(String authorization, Long organizerKey, Long webinarKey, Long sessionKey, Long registrantKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(sessionKey == null) {
throw new ApiException("Required parameter sessionKey is null.");
}
if(registrantKey == null) {
throw new ApiException("Required parameter registrantKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/sessions/{sessionKey}/attendees/{registrantKey}/polls"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()))
.replaceAll("\\{" + "sessionKey" + "\\}", apiInvoker.escapeString(sessionKey.toString()))
.replaceAll("\\{" + "registrantKey" + "\\}", apiInvoker.escapeString(registrantKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", PollAnswer.class);
}
return null;
}
/**
* Get attendee questions
* Get questions asked by an attendee during a webinar session. For technical reasons, this call cannot be executed from this documentation. Please use the curl command to execute it.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param sessionKey The key of the webinar session
* @param registrantKey The key of the registrant
* @return List<AttendeeQuestion>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getAttendeeQuestions(String authorization, Long organizerKey, Long webinarKey, Long sessionKey, Long registrantKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(sessionKey == null) {
throw new ApiException("Required parameter sessionKey is null.");
}
if(registrantKey == null) {
throw new ApiException("Required parameter registrantKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/sessions/{sessionKey}/attendees/{registrantKey}/questions"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()))
.replaceAll("\\{" + "sessionKey" + "\\}", apiInvoker.escapeString(sessionKey.toString()))
.replaceAll("\\{" + "registrantKey" + "\\}", apiInvoker.escapeString(registrantKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", AttendeeQuestion.class);
}
return null;
}
/**
* Get attendee survey answers
* Retrieve survey answers from a particular attendee during a webinar session. For technical reasons, this call cannot be executed from this documentation. Please use the curl command to execute it.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param sessionKey The key of the webinar session
* @param registrantKey The key of the registrant
* @return List<PollAnswer>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getAttendeeSurveyAnswers(String authorization, Long organizerKey, Long webinarKey, Long sessionKey, Long registrantKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(sessionKey == null) {
throw new ApiException("Required parameter sessionKey is null.");
}
if(registrantKey == null) {
throw new ApiException("Required parameter registrantKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/sessions/{sessionKey}/attendees/{registrantKey}/surveys"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()))
.replaceAll("\\{" + "sessionKey" + "\\}", apiInvoker.escapeString(sessionKey.toString()))
.replaceAll("\\{" + "registrantKey" + "\\}", apiInvoker.escapeString(registrantKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", PollAnswer.class);
}
return null;
}
/**
* Get session attendees
* Retrieve details for all attendees of a specific webinar session. For technical reasons, this call cannot be executed from this documentation. Please use the curl command to execute it.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param sessionKey The key of the webinar session
* @return List<Attendee>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getAttendees(String authorization, Long organizerKey, Long webinarKey, Long sessionKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(sessionKey == null) {
throw new ApiException("Required parameter sessionKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/sessions/{sessionKey}/attendees"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()))
.replaceAll("\\{" + "sessionKey" + "\\}", apiInvoker.escapeString(sessionKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", Attendee.class);
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy