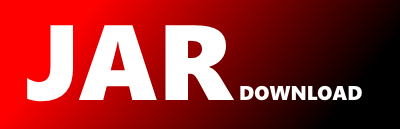
com.logmein.gotowebinar_2_0.api.RegistrantsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api;
import com.logmein.gotowebinar_2_0.api.common.ApiException;
import com.logmein.gotowebinar_2_0.api.common.ApiInvoker;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
import com.logmein.gotowebinar_2_0.api.model.RegistrantFields;
import com.logmein.gotowebinar_2_0.api.model.RegistrantCreated;
import com.logmein.gotowebinar_2_0.api.model.Registrant;
import com.logmein.gotowebinar_2_0.api.model.RegistrantDetailed;
import com.logmein.gotowebinar_2_0.api.model.RegistrationFields;
import com.sun.jersey.multipart.FormDataMultiPart;
import javax.ws.rs.core.MediaType;
import java.util.*;
public class RegistrantsApi {
private String basePath = "https://api.getgo.com/G2W/rest/v2";
private ApiInvoker apiInvoker = ApiInvoker.getInstance();
/**
* Initializes a new instance of the RegistrantsApi class using the
* default endpoint base url for the services being accessed.
*/
public RegistrantsApi() {
}
/**
* Initializes a new instance of the RegistrantsApi class using an endpoint base
* url other than the default. Use this constructor only if you should need
* to override the default endpoint base url.
* @param basePath The endpoint base url for the services being accessed.
*/
public RegistrantsApi(String basePath) {
this.basePath = basePath;
}
public ApiInvoker getInvoker() {
return apiInvoker;
}
public String getBasePath() {
return basePath;
}
/**
* Create registrant
* Register an attendee for a scheduled webinar. The response contains the registrantKey and join URL for the registrant. An email will be sent to the registrant unless the organizer turns off the confirmation email setting from the GoToWebinar website. Please note that you must provide all required fields including custom fields defined during the webinar creation. Use the API call 'Get registration fields' to get a list of all fields, if they are required, and their possible values. At this time there are two versions of the 'Create Registrant' call. The first version only accepts firstName, lastName, and email and ignores all other fields. If you have custom fields or want to capture additional information this version won't work for you. The second version allows you to pass all required and optional fields, including custom fields defined when creating the webinar. To use the second version you must pass the header value 'Accept: application/vnd.citrix.g2wapi-v1.1+json' instead of 'Accept: application/json'. Leaving this header out results in the first version of the API call.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param accept Set to 'application/vnd.citrix.g2wapi-v1.1+json' to make a registration using fields (custom or default) additional to the basic ones.
* @param resendConfirmation Indicates whether the confirmation email should be resent when a registrant is re-registered. The default value is false.
* @param body The registrant details. To get all possible values run the API call 'Get registration fields' which is also part of this documentation.
* @return RegistrantCreated
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public RegistrantCreated createRegistrant(String authorization, Long organizerKey, Long webinarKey, String accept, Boolean resendConfirmation, RegistrantFields body) throws ApiException {
Object postBody = body;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(body == null) {
throw new ApiException("Required parameter body is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/registrants"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String resendConfirmationString = JsonUtil.Stringify(resendConfirmation);
if(!"null".equals(resendConfirmationString)){
queryParams.put("resendConfirmation", resendConfirmationString);
}
headerParams.put("Authorization", authorization);
headerParams.put("Accept", accept);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "POST", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (RegistrantCreated) ApiInvoker.deserialize(response, "", RegistrantCreated.class);
}
return null;
}
/**
* Delete registrant
* Removes a webinar registrant from current registrations for the specified webinar. The webinar must be a scheduled, future webinar.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param registrantKey The key of the registrant
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public void deleteRegistrant(String authorization, Long organizerKey, Long webinarKey, Long registrantKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(registrantKey == null) {
throw new ApiException("Required parameter registrantKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/registrants/{registrantKey}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()))
.replaceAll("\\{" + "registrantKey" + "\\}", apiInvoker.escapeString(registrantKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
apiInvoker.invokeAPI(basePath, path, "DELETE", queryParams, postBody, headerParams, formParams, contentType);
}
/**
* Get registrants
* Retrieve registration details for all registrants of a specific webinar. Registrant details will not include all fields captured when creating the registrant. To see all data, use the API call 'Get Registrant'. Registrants can have one of the following states;
WAITING - registrant registered and is awaiting approval (where organizer has required approval),
APPROVED - registrant registered and is approved, and
DENIED - registrant registered and was denied.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @return List<Registrant>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getAllRegistrantsForWebinar(String authorization, Long organizerKey, Long webinarKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/registrants"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", Registrant.class);
}
return null;
}
/**
* Get registrant
* Retrieve registration details for a specific registrant.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param registrantKey The key of the registrant
* @return RegistrantDetailed
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public RegistrantDetailed getRegistrant(String authorization, Long organizerKey, Long webinarKey, Long registrantKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(registrantKey == null) {
throw new ApiException("Required parameter registrantKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/registrants/{registrantKey}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()))
.replaceAll("\\{" + "registrantKey" + "\\}", apiInvoker.escapeString(registrantKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (RegistrantDetailed) ApiInvoker.deserialize(response, "", RegistrantDetailed.class);
}
return null;
}
/**
* Get registration fields
* Retrieve required, optional registration, and custom questions for a specified webinar.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @return RegistrationFields
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public RegistrationFields getRegistrationFields(String authorization, Long organizerKey, Long webinarKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/registrants/fields"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (RegistrationFields) ApiInvoker.deserialize(response, "", RegistrationFields.class);
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy