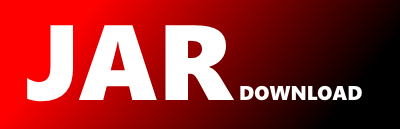
com.logmein.gotowebinar_2_0.api.WebinarsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api;
import com.logmein.gotowebinar_2_0.api.common.ApiException;
import com.logmein.gotowebinar_2_0.api.common.ApiInvoker;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
import com.logmein.gotowebinar_2_0.api.model.WebinarReqCreate;
import com.logmein.gotowebinar_2_0.api.model.CreatedWebinar;
import com.logmein.gotowebinar_2_0.api.model.ReportingWebinarsResponse;
import java.util.Date;
import com.logmein.gotowebinar_2_0.api.model.ReportingAttendeeResponse;
import com.logmein.gotowebinar_2_0.api.model.Audio;
import com.logmein.gotowebinar_2_0.api.model.BrokerWebinar;
import com.logmein.gotowebinar_2_0.api.model.SessionPerformance;
import com.logmein.gotowebinar_2_0.api.model.WebinarByKey;
import com.logmein.gotowebinar_2_0.api.model.DateTimeRange;
import com.logmein.gotowebinar_2_0.api.model.AudioUpdate;
import com.logmein.gotowebinar_2_0.api.model.WebinarReqUpdate;
import com.sun.jersey.multipart.FormDataMultiPart;
import javax.ws.rs.core.MediaType;
import java.util.*;
public class WebinarsApi {
private String basePath = "https://api.getgo.com/G2W/rest/v2";
private ApiInvoker apiInvoker = ApiInvoker.getInstance();
/**
* Initializes a new instance of the WebinarsApi class using the
* default endpoint base url for the services being accessed.
*/
public WebinarsApi() {
}
/**
* Initializes a new instance of the WebinarsApi class using an endpoint base
* url other than the default. Use this constructor only if you should need
* to override the default endpoint base url.
* @param basePath The endpoint base url for the services being accessed.
*/
public WebinarsApi(String basePath) {
this.basePath = basePath;
}
public ApiInvoker getInvoker() {
return apiInvoker;
}
public String getBasePath() {
return basePath;
}
/**
* Cancel webinar
* Cancels a specific webinar. If the webinar is a series or sequence, this call deletes all scheduled sessions. To send cancellation emails to registrants set sendCancellationEmails=true in the request. When the cancellation emails are sent, the default generated message is used in the cancellation email body.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param sendCancellationEmails Indicates whether cancellation notice emails should be sent. The default value is false
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public void cancelWebinar(String authorization, Long organizerKey, Long webinarKey, Boolean sendCancellationEmails) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String sendCancellationEmailsString = JsonUtil.Stringify(sendCancellationEmails);
if(!"null".equals(sendCancellationEmailsString)){
queryParams.put("sendCancellationEmails", sendCancellationEmailsString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
apiInvoker.invokeAPI(basePath, path, "DELETE", queryParams, postBody, headerParams, formParams, contentType);
}
/**
* Create webinar
* Creates a single session webinar, a sequence of webinars or a series of webinars depending on the type field in the body: \"single_session\" creates a single webinar session, \"sequence\" creates a webinar with multiple meeting times where attendees are expected to be the same for all sessions, and \"series\" creates a webinar with multiple meetings times where attendees choose only one to attend. The default, if no type is declared, is single_session. A sequence webinar requires a \"recurrenceStart\" object consisting of a \"startTime\" and \"endTime\" key for the first webinar of the sequence, a \"recurrencePattern\" of \"daily\", \"weekly\", \"monthly\", and a \"recurrenceEnd\" which is the last date of the sequence (for example, 2016-12-01). A series webinar requires a \"times\" array with a discrete \"startTime\" and \"endTime\" for each webinar in the series. The call requires a webinar subject and description. The \"isPasswordProtected\" sets whether the webinar requires a password for attendees to join. If set to True, the organizer must go to Registration Settings at My Webinars (https://global.gotowebinar.com/webinars.tmpl) and add the password to the webinar, and send the password to the registrants. The response provides a numeric webinarKey in string format for the new webinar. Once a webinar has been created with this method, you can accept registrations. To create a scheduled simulive webinar set the \"experienceType\" as \"SIMULIVE\" along with the \"recordingAssetKey\". The \"recordingAssetKey\" is the unique identifier for the recording asset with which the simulive webinar should be created from. In case the asset was created as an online recording the simulive webinar settings, poll and surveys would be copied from the webinar whose session was recorded. The \"recordingAssetKey\" can be obtained using the new search recordingassets call. To create an on demand webinar set \"isOndemand\" to true along with the \"experienceType\" and the \"recordingAssetKey\". Simulive does not support sequence webinars.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param body The webinar details
* @return CreatedWebinar
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public CreatedWebinar createWebinar(String authorization, Long organizerKey, WebinarReqCreate body) throws ApiException {
Object postBody = body;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(body == null) {
throw new ApiException("Required parameter body is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "POST", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (CreatedWebinar) ApiInvoker.deserialize(response, "", CreatedWebinar.class);
}
return null;
}
/**
* Get all webinars for an account
* Retrieves the list of webinars for an account within a given date range. __*Page*__ and __*size*__ parameters are optional. Default __*page*__ is 0 and default __*size*__ is 20. For technical reasons, this call cannot be executed from this documentation. Please use the curl command to execute it.
* @param authorization Access token
* @param accountKey The key of the account
* @param fromTime A required start of datetime range in ISO8601 UTC format, e.g. 2015-07-13T10:00:00Z
* @param toTime A required end of datetime range in ISO8601 UTC format, e.g. 2015-07-13T22:00:00Z
* @param page The page number to be displayed. The first page is 0.
* @param size The size of the page.
* @return ReportingWebinarsResponse
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public ReportingWebinarsResponse getAllAccountWebinars(String authorization, Long accountKey, Date fromTime, Date toTime, Long page, Long size) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(accountKey == null) {
throw new ApiException("Required parameter accountKey is null.");
}
if(fromTime == null) {
throw new ApiException("Required parameter fromTime is null.");
}
if(toTime == null) {
throw new ApiException("Required parameter toTime is null.");
}
// create path and map variables
String path = "/accounts/{accountKey}/webinars"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "accountKey" + "\\}", apiInvoker.escapeString(accountKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String fromTimeString = JsonUtil.Stringify(fromTime);
if(!"null".equals(fromTimeString)){
queryParams.put("fromTime", fromTimeString);
}
String toTimeString = JsonUtil.Stringify(toTime);
if(!"null".equals(toTimeString)){
queryParams.put("toTime", toTimeString);
}
String pageString = JsonUtil.Stringify(page);
if(!"null".equals(pageString)){
queryParams.put("page", pageString);
}
String sizeString = JsonUtil.Stringify(size);
if(!"null".equals(sizeString)){
queryParams.put("size", sizeString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (ReportingWebinarsResponse) ApiInvoker.deserialize(response, "", ReportingWebinarsResponse.class);
}
return null;
}
/**
* Get attendees for all webinar sessions
* Returns all attendees for all sessions of the specified webinar.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @return ReportingAttendeeResponse
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public ReportingAttendeeResponse getAttendeesForAllWebinarSessions(String authorization, Long organizerKey, Long webinarKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/attendees"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (ReportingAttendeeResponse) ApiInvoker.deserialize(response, "", ReportingAttendeeResponse.class);
}
return null;
}
/**
* Get audio information
* Retrieves the audio/conferencing information for a specific webinar.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @return Audio
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public Audio getAudioInformation(String authorization, Long organizerKey, Long webinarKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/audio"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (Audio) ApiInvoker.deserialize(response, "", Audio.class);
}
return null;
}
/**
* Get All Insession Webinars
* Returns all insession webinars for the currently authenticated organizer that are scheduled within the specified date/time range. All inession webinars are returned in case no date/time range is provided.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param fromTime Start of datetime range in ISO8601 UTC format, e.g. 2015-07-13T10:00:00Z
* @param toTime End of datetime range in ISO8601 UTC format, e.g. 2015-07-13T22:00:00Z
* @return List<BrokerWebinar>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getInSessionWebinars(String authorization, Long organizerKey, Date fromTime, Date toTime) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/insessionWebinars"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String fromTimeString = JsonUtil.Stringify(fromTime);
if(!"null".equals(fromTimeString)){
queryParams.put("fromTime", fromTimeString);
}
String toTimeString = JsonUtil.Stringify(toTime);
if(!"null".equals(toTimeString)){
queryParams.put("toTime", toTimeString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", BrokerWebinar.class);
}
return null;
}
/**
* Get performance for all webinar sessions
* Gets performance details for all sessions of a specific webinar.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @return Map<String, SessionPerformance>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public Map getPerformanceForAllWebinarSessions(String authorization, Long organizerKey, Long webinarKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/performance"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (Map) ApiInvoker.deserialize(response, "Map", SessionPerformance.class);
}
return null;
}
/**
* Get webinar
* Retrieve information on a specific webinar. If the type of the webinar is 'sequence', a sequence of future times will be provided. Webinars of type 'series' are treated the same as normal webinars - each session in the webinar series has a different webinarKey. If an organizer cancels a webinar, then a request to get that webinar would return a '404 Not Found' error.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @return WebinarByKey
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public WebinarByKey getWebinar(String authorization, Long organizerKey, Long webinarKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (WebinarByKey) ApiInvoker.deserialize(response, "", WebinarByKey.class);
}
return null;
}
/**
* Get webinar meeting times
* Retrieves the meeting times for a webinar.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @return List<DateTimeRange>
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public List getWebinarMeetingTimes(String authorization, Long organizerKey, Long webinarKey) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/meetingtimes"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (List) ApiInvoker.deserialize(response, "List", DateTimeRange.class);
}
return null;
}
/**
* Get Webinars
* Returns upcoming and past webinars for the currently authenticated organizer that are scheduled within the specified date/time range.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param fromTime A required start of datetime range in ISO8601 UTC format, e.g. 2015-07-13T10:00:00Z
* @param toTime A required end of datetime range in ISO8601 UTC format, e.g. 2015-07-13T22:00:00Z
* @return ReportingWebinarsResponse
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public ReportingWebinarsResponse getWebinars(String authorization, Long organizerKey, Date fromTime, Date toTime) throws ApiException {
Object postBody = null;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(fromTime == null) {
throw new ApiException("Required parameter fromTime is null.");
}
if(toTime == null) {
throw new ApiException("Required parameter toTime is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String fromTimeString = JsonUtil.Stringify(fromTime);
if(!"null".equals(fromTimeString)){
queryParams.put("fromTime", fromTimeString);
}
String toTimeString = JsonUtil.Stringify(toTime);
if(!"null".equals(toTimeString)){
queryParams.put("toTime", toTimeString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "GET", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (ReportingWebinarsResponse) ApiInvoker.deserialize(response, "", ReportingWebinarsResponse.class);
}
return null;
}
/**
* Update audio information
* Updates the audio/conferencing settings for a specific webinar
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param notifyParticipants Defines whether to send notifications to participants
* @param body The audio/conferencing settings
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public void updateAudioInformation(String authorization, Long organizerKey, Long webinarKey, Boolean notifyParticipants, AudioUpdate body) throws ApiException {
Object postBody = body;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(body == null) {
throw new ApiException("Required parameter body is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}/audio"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String notifyParticipantsString = JsonUtil.Stringify(notifyParticipants);
if(!"null".equals(notifyParticipantsString)){
queryParams.put("notifyParticipants", notifyParticipantsString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
apiInvoker.invokeAPI(basePath, path, "POST", queryParams, postBody, headerParams, formParams, contentType);
}
/**
* Update webinar
* Updates a webinar. The call requires at least one of the parameters in the request body. The request completely replaces the existing session, series, or sequence and so must include the full definition of each as for the Create call. Set notifyParticipants=true to send update emails to registrants.
* @param authorization Access token
* @param organizerKey The key of the organizer
* @param webinarKey The key of the webinar
* @param notifyParticipants Defines whether to send notifications to participants
* @param body The webinar details
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public void updateWebinar(String authorization, Long organizerKey, Long webinarKey, Boolean notifyParticipants, WebinarReqUpdate body) throws ApiException {
Object postBody = body;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(organizerKey == null) {
throw new ApiException("Required parameter organizerKey is null.");
}
if(webinarKey == null) {
throw new ApiException("Required parameter webinarKey is null.");
}
if(body == null) {
throw new ApiException("Required parameter body is null.");
}
// create path and map variables
String path = "/organizers/{organizerKey}/webinars/{webinarKey}"
.replaceAll("\\{format\\}", "json")
.replaceAll("\\{" + "organizerKey" + "\\}", apiInvoker.escapeString(organizerKey.toString()))
.replaceAll("\\{" + "webinarKey" + "\\}", apiInvoker.escapeString(webinarKey.toString()));
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String notifyParticipantsString = JsonUtil.Stringify(notifyParticipants);
if(!"null".equals(notifyParticipantsString)){
queryParams.put("notifyParticipants", notifyParticipantsString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
apiInvoker.invokeAPI(basePath, path, "PUT", queryParams, postBody, headerParams, formParams, contentType);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy