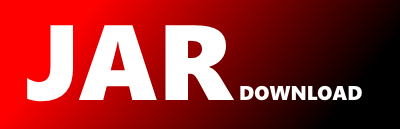
com.logmein.gotowebinar_2_0.api.model.AttendanceStatistics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Describes attendance metrics for a webinar session.
*/
public class AttendanceStatistics {
/* The number of registrations for the webinar */
private Integer registrantCount = null;
/* The percentage of registrants that actually attended the webinar session */
private Float percentageAttendance = null;
/* Numerical value 1-100 (100 being most interested) indicating the average interest rating of the webinar attendees */
private Float averageInterestRating = null;
/* Average based on the focus of the attendees Viewer during the webinar session */
private Float averageAttentiveness = null;
/* Average attendance time in seconds */
private Float averageAttendanceTimeSeconds = null;
/**
* @return The number of registrations for the webinar
*/
public Integer getRegistrantCount() {
return registrantCount;
}
/**
* @param registrantCount The number of registrations for the webinar
*/
public void setRegistrantCount(Integer registrantCount) {
this.registrantCount = registrantCount;
}
/**
* @return The percentage of registrants that actually attended the webinar session
*/
public Float getPercentageAttendance() {
return percentageAttendance;
}
/**
* @param percentageAttendance The percentage of registrants that actually attended the webinar session
*/
public void setPercentageAttendance(Float percentageAttendance) {
this.percentageAttendance = percentageAttendance;
}
/**
* @return Numerical value 1-100 (100 being most interested) indicating the average interest rating of the webinar attendees
*/
public Float getAverageInterestRating() {
return averageInterestRating;
}
/**
* @param averageInterestRating Numerical value 1-100 (100 being most interested) indicating the average interest rating of the webinar attendees
*/
public void setAverageInterestRating(Float averageInterestRating) {
this.averageInterestRating = averageInterestRating;
}
/**
* @return Average based on the focus of the attendees Viewer during the webinar session
*/
public Float getAverageAttentiveness() {
return averageAttentiveness;
}
/**
* @param averageAttentiveness Average based on the focus of the attendees Viewer during the webinar session
*/
public void setAverageAttentiveness(Float averageAttentiveness) {
this.averageAttentiveness = averageAttentiveness;
}
/**
* @return Average attendance time in seconds
*/
public Float getAverageAttendanceTimeSeconds() {
return averageAttendanceTimeSeconds;
}
/**
* @param averageAttendanceTimeSeconds Average attendance time in seconds
*/
public void setAverageAttendanceTimeSeconds(Float averageAttendanceTimeSeconds) {
this.averageAttendanceTimeSeconds = averageAttendanceTimeSeconds;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AttendanceStatistics {\n");
String registrantCountString = JsonUtil.Stringify(registrantCount);
if (registrantCountString != null && !registrantCountString.isEmpty())
sb.append(String.format(" registrantCount: %s\n", registrantCountString));
String percentageAttendanceString = JsonUtil.Stringify(percentageAttendance);
if (percentageAttendanceString != null && !percentageAttendanceString.isEmpty())
sb.append(String.format(" percentageAttendance: %s\n", percentageAttendanceString));
String averageInterestRatingString = JsonUtil.Stringify(averageInterestRating);
if (averageInterestRatingString != null && !averageInterestRatingString.isEmpty())
sb.append(String.format(" averageInterestRating: %s\n", averageInterestRatingString));
String averageAttentivenessString = JsonUtil.Stringify(averageAttentiveness);
if (averageAttentivenessString != null && !averageAttentivenessString.isEmpty())
sb.append(String.format(" averageAttentiveness: %s\n", averageAttentivenessString));
String averageAttendanceTimeSecondsString = JsonUtil.Stringify(averageAttendanceTimeSeconds);
if (averageAttendanceTimeSecondsString != null && !averageAttendanceTimeSecondsString.isEmpty())
sb.append(String.format(" averageAttendanceTimeSeconds: %s\n", averageAttendanceTimeSecondsString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy