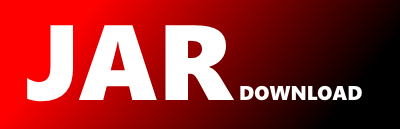
com.logmein.gotowebinar_2_0.api.model.Coorganizer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Describes a webinar co-organizer. A co-organizer without a GoToWebinar account is marked as external and is returned without a surname
*/
public class Coorganizer {
/* The co-organizer's join link */
private String joinLink = null;
/* The co-organizer's email address */
private String email = null;
/* The co-organizer's organizer key. A new member key is created for external co-organizers */
private String memberKey = null;
/* If the co-organizer has no GoToWebinar account, this value is set to 'true' */
private Boolean external = null;
/* The co-organizer's surname. For external co-organizers this parameter is not returned */
private String surname = null;
/* The co-organizer's given name */
private String givenName = null;
/**
* @return The co-organizer's join link
*/
public String getJoinLink() {
return joinLink;
}
/**
* @param joinLink The co-organizer's join link
*/
public void setJoinLink(String joinLink) {
this.joinLink = joinLink;
}
/**
* @return The co-organizer's email address
*/
public String getEmail() {
return email;
}
/**
* @param email The co-organizer's email address
*/
public void setEmail(String email) {
this.email = email;
}
/**
* @return The co-organizer's organizer key. A new member key is created for external co-organizers
*/
public String getMemberKey() {
return memberKey;
}
/**
* @param memberKey The co-organizer's organizer key. A new member key is created for external co-organizers
*/
public void setMemberKey(String memberKey) {
this.memberKey = memberKey;
}
/**
* @return If the co-organizer has no GoToWebinar account, this value is set to 'true'
*/
public Boolean getExternal() {
return external;
}
/**
* @param external If the co-organizer has no GoToWebinar account, this value is set to 'true'
*/
public void setExternal(Boolean external) {
this.external = external;
}
/**
* @return The co-organizer's surname. For external co-organizers this parameter is not returned
*/
public String getSurname() {
return surname;
}
/**
* @param surname The co-organizer's surname. For external co-organizers this parameter is not returned
*/
public void setSurname(String surname) {
this.surname = surname;
}
/**
* @return The co-organizer's given name
*/
public String getGivenName() {
return givenName;
}
/**
* @param givenName The co-organizer's given name
*/
public void setGivenName(String givenName) {
this.givenName = givenName;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Coorganizer {\n");
String joinLinkString = JsonUtil.Stringify(joinLink);
if (joinLinkString != null && !joinLinkString.isEmpty())
sb.append(String.format(" joinLink: %s\n", joinLinkString));
String emailString = JsonUtil.Stringify(email);
if (emailString != null && !emailString.isEmpty())
sb.append(String.format(" email: %s\n", emailString));
String memberKeyString = JsonUtil.Stringify(memberKey);
if (memberKeyString != null && !memberKeyString.isEmpty())
sb.append(String.format(" memberKey: %s\n", memberKeyString));
String externalString = JsonUtil.Stringify(external);
if (externalString != null && !externalString.isEmpty())
sb.append(String.format(" external: %s\n", externalString));
String surnameString = JsonUtil.Stringify(surname);
if (surnameString != null && !surnameString.isEmpty())
sb.append(String.format(" surname: %s\n", surnameString));
String givenNameString = JsonUtil.Stringify(givenName);
if (givenNameString != null && !givenNameString.isEmpty())
sb.append(String.format(" givenName: %s\n", givenNameString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy