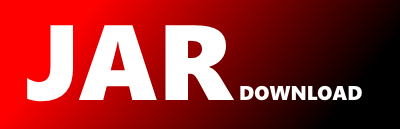
com.logmein.gotowebinar_2_0.api.model.Registrant Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import java.util.Date;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Describes a webinar registrant
*/
public class Registrant {
/* The registrant's last name */
private String lastName = null;
/* The registrant's email address */
private String email = null;
/* The registrant's first name */
private String firstName = null;
/* The registrant's key */
private Long registrantKey = null;
/* The registration date and time */
private Date registrationDate = null;
/* The registration status */
private StatusEnum status = null;
/* The URL the registrant will use to join the webinar */
private String joinUrl = null;
/* The time zone where the webinar will take place */
private String timeZone = null;
/**
* The registration status
*/
public enum StatusEnum { APPROVED, DENIED, WAITING }
/**
* @return The registrant's last name
*/
public String getLastName() {
return lastName;
}
/**
* @param lastName The registrant's last name
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
* @return The registrant's email address
*/
public String getEmail() {
return email;
}
/**
* @param email The registrant's email address
*/
public void setEmail(String email) {
this.email = email;
}
/**
* @return The registrant's first name
*/
public String getFirstName() {
return firstName;
}
/**
* @param firstName The registrant's first name
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
* @return The registrant's key
*/
public Long getRegistrantKey() {
return registrantKey;
}
/**
* @param registrantKey The registrant's key
*/
public void setRegistrantKey(Long registrantKey) {
this.registrantKey = registrantKey;
}
/**
* @return The registration date and time
*/
public Date getRegistrationDate() {
return registrationDate;
}
/**
* @param registrationDate The registration date and time
*/
public void setRegistrationDate(Date registrationDate) {
this.registrationDate = registrationDate;
}
/**
* @return The registration status
*/
public StatusEnum getStatus() {
return status;
}
/**
* @param status The registration status
*/
public void setStatus(StatusEnum status) {
this.status = status;
}
/**
* @return The URL the registrant will use to join the webinar
*/
public String getJoinUrl() {
return joinUrl;
}
/**
* @param joinUrl The URL the registrant will use to join the webinar
*/
public void setJoinUrl(String joinUrl) {
this.joinUrl = joinUrl;
}
/**
* @return The time zone where the webinar will take place
*/
public String getTimeZone() {
return timeZone;
}
/**
* @param timeZone The time zone where the webinar will take place
*/
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Registrant {\n");
String lastNameString = JsonUtil.Stringify(lastName);
if (lastNameString != null && !lastNameString.isEmpty())
sb.append(String.format(" lastName: %s\n", lastNameString));
String emailString = JsonUtil.Stringify(email);
if (emailString != null && !emailString.isEmpty())
sb.append(String.format(" email: %s\n", emailString));
String firstNameString = JsonUtil.Stringify(firstName);
if (firstNameString != null && !firstNameString.isEmpty())
sb.append(String.format(" firstName: %s\n", firstNameString));
String registrantKeyString = JsonUtil.Stringify(registrantKey);
if (registrantKeyString != null && !registrantKeyString.isEmpty())
sb.append(String.format(" registrantKey: %s\n", registrantKeyString));
String registrationDateString = JsonUtil.Stringify(registrationDate);
if (registrationDateString != null && !registrationDateString.isEmpty())
sb.append(String.format(" registrationDate: %s\n", registrationDateString));
String statusString = JsonUtil.Stringify(status);
if (statusString != null && !statusString.isEmpty())
sb.append(String.format(" status: %s\n", statusString));
String joinUrlString = JsonUtil.Stringify(joinUrl);
if (joinUrlString != null && !joinUrlString.isEmpty())
sb.append(String.format(" joinUrl: %s\n", joinUrlString));
String timeZoneString = JsonUtil.Stringify(timeZone);
if (timeZoneString != null && !timeZoneString.isEmpty())
sb.append(String.format(" timeZone: %s\n", timeZoneString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy