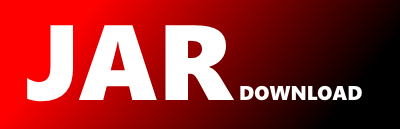
com.logmein.gotowebinar_2_0.api.model.RegistrantFields Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import com.logmein.gotowebinar_2_0.api.model.RegistrantQAResponse;
import java.util.ArrayList;
import java.util.List;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Detailed description of a all fields to register a registrant for a webinar.
*/
public class RegistrantFields {
/* The registrant's first name */
private String firstName = null;
/* The registrant's last name */
private String lastName = null;
/* The registrant's email address */
private String email = null;
/* The source that led to the registration. This can be any string like 'Newsletter 123' or 'Marketing campaign ABC' */
private String source = null;
/* The registrant's address */
private String address = null;
/* The registrant's city */
private String city = null;
/* The registrant's state (US only) */
private String state = null;
/* The registrant's zip (post) code */
private String zipCode = null;
/* The registrant's country */
private String country = null;
/* The registrant's phone */
private String phone = null;
/* The registrant's organization */
private String organization = null;
/* The registrant's job title */
private String jobTitle = null;
/* Any questions or comments the registrant made at the time of registration */
private String questionsAndComments = null;
/* The type of industry the registrant's organization belongs to */
private String industry = null;
/* The size in employees of the registrant's organization */
private String numberOfEmployees = null;
/* The time frame within which the product will be purchased */
private String purchasingTimeFrame = null;
/* The registrant's role in purchasing the product */
private String purchasingRole = null;
/* Set the answers of all questions */
private List responses = new ArrayList();
/**
* @return The registrant's first name
*/
public String getFirstName() {
return firstName;
}
/**
* @param firstName The registrant's first name
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
* @return The registrant's last name
*/
public String getLastName() {
return lastName;
}
/**
* @param lastName The registrant's last name
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
* @return The registrant's email address
*/
public String getEmail() {
return email;
}
/**
* @param email The registrant's email address
*/
public void setEmail(String email) {
this.email = email;
}
/**
* @return The source that led to the registration. This can be any string like 'Newsletter 123' or 'Marketing campaign ABC'
*/
public String getSource() {
return source;
}
/**
* @param source The source that led to the registration. This can be any string like 'Newsletter 123' or 'Marketing campaign ABC'
*/
public void setSource(String source) {
this.source = source;
}
/**
* @return The registrant's address
*/
public String getAddress() {
return address;
}
/**
* @param address The registrant's address
*/
public void setAddress(String address) {
this.address = address;
}
/**
* @return The registrant's city
*/
public String getCity() {
return city;
}
/**
* @param city The registrant's city
*/
public void setCity(String city) {
this.city = city;
}
/**
* @return The registrant's state (US only)
*/
public String getState() {
return state;
}
/**
* @param state The registrant's state (US only)
*/
public void setState(String state) {
this.state = state;
}
/**
* @return The registrant's zip (post) code
*/
public String getZipCode() {
return zipCode;
}
/**
* @param zipCode The registrant's zip (post) code
*/
public void setZipCode(String zipCode) {
this.zipCode = zipCode;
}
/**
* @return The registrant's country
*/
public String getCountry() {
return country;
}
/**
* @param country The registrant's country
*/
public void setCountry(String country) {
this.country = country;
}
/**
* @return The registrant's phone
*/
public String getPhone() {
return phone;
}
/**
* @param phone The registrant's phone
*/
public void setPhone(String phone) {
this.phone = phone;
}
/**
* @return The registrant's organization
*/
public String getOrganization() {
return organization;
}
/**
* @param organization The registrant's organization
*/
public void setOrganization(String organization) {
this.organization = organization;
}
/**
* @return The registrant's job title
*/
public String getJobTitle() {
return jobTitle;
}
/**
* @param jobTitle The registrant's job title
*/
public void setJobTitle(String jobTitle) {
this.jobTitle = jobTitle;
}
/**
* @return Any questions or comments the registrant made at the time of registration
*/
public String getQuestionsAndComments() {
return questionsAndComments;
}
/**
* @param questionsAndComments Any questions or comments the registrant made at the time of registration
*/
public void setQuestionsAndComments(String questionsAndComments) {
this.questionsAndComments = questionsAndComments;
}
/**
* @return The type of industry the registrant's organization belongs to
*/
public String getIndustry() {
return industry;
}
/**
* @param industry The type of industry the registrant's organization belongs to
*/
public void setIndustry(String industry) {
this.industry = industry;
}
/**
* @return The size in employees of the registrant's organization
*/
public String getNumberOfEmployees() {
return numberOfEmployees;
}
/**
* @param numberOfEmployees The size in employees of the registrant's organization
*/
public void setNumberOfEmployees(String numberOfEmployees) {
this.numberOfEmployees = numberOfEmployees;
}
/**
* @return The time frame within which the product will be purchased
*/
public String getPurchasingTimeFrame() {
return purchasingTimeFrame;
}
/**
* @param purchasingTimeFrame The time frame within which the product will be purchased
*/
public void setPurchasingTimeFrame(String purchasingTimeFrame) {
this.purchasingTimeFrame = purchasingTimeFrame;
}
/**
* @return The registrant's role in purchasing the product
*/
public String getPurchasingRole() {
return purchasingRole;
}
/**
* @param purchasingRole The registrant's role in purchasing the product
*/
public void setPurchasingRole(String purchasingRole) {
this.purchasingRole = purchasingRole;
}
/**
* @return Set the answers of all questions
*/
public List getResponses() {
return responses;
}
/**
* @param responses Set the answers of all questions
*/
public void setResponses(List responses) {
this.responses = responses;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RegistrantFields {\n");
String firstNameString = JsonUtil.Stringify(firstName);
if (firstNameString != null && !firstNameString.isEmpty())
sb.append(String.format(" firstName: %s\n", firstNameString));
String lastNameString = JsonUtil.Stringify(lastName);
if (lastNameString != null && !lastNameString.isEmpty())
sb.append(String.format(" lastName: %s\n", lastNameString));
String emailString = JsonUtil.Stringify(email);
if (emailString != null && !emailString.isEmpty())
sb.append(String.format(" email: %s\n", emailString));
String sourceString = JsonUtil.Stringify(source);
if (sourceString != null && !sourceString.isEmpty())
sb.append(String.format(" source: %s\n", sourceString));
String addressString = JsonUtil.Stringify(address);
if (addressString != null && !addressString.isEmpty())
sb.append(String.format(" address: %s\n", addressString));
String cityString = JsonUtil.Stringify(city);
if (cityString != null && !cityString.isEmpty())
sb.append(String.format(" city: %s\n", cityString));
String stateString = JsonUtil.Stringify(state);
if (stateString != null && !stateString.isEmpty())
sb.append(String.format(" state: %s\n", stateString));
String zipCodeString = JsonUtil.Stringify(zipCode);
if (zipCodeString != null && !zipCodeString.isEmpty())
sb.append(String.format(" zipCode: %s\n", zipCodeString));
String countryString = JsonUtil.Stringify(country);
if (countryString != null && !countryString.isEmpty())
sb.append(String.format(" country: %s\n", countryString));
String phoneString = JsonUtil.Stringify(phone);
if (phoneString != null && !phoneString.isEmpty())
sb.append(String.format(" phone: %s\n", phoneString));
String organizationString = JsonUtil.Stringify(organization);
if (organizationString != null && !organizationString.isEmpty())
sb.append(String.format(" organization: %s\n", organizationString));
String jobTitleString = JsonUtil.Stringify(jobTitle);
if (jobTitleString != null && !jobTitleString.isEmpty())
sb.append(String.format(" jobTitle: %s\n", jobTitleString));
String questionsAndCommentsString = JsonUtil.Stringify(questionsAndComments);
if (questionsAndCommentsString != null && !questionsAndCommentsString.isEmpty())
sb.append(String.format(" questionsAndComments: %s\n", questionsAndCommentsString));
String industryString = JsonUtil.Stringify(industry);
if (industryString != null && !industryString.isEmpty())
sb.append(String.format(" industry: %s\n", industryString));
String numberOfEmployeesString = JsonUtil.Stringify(numberOfEmployees);
if (numberOfEmployeesString != null && !numberOfEmployeesString.isEmpty())
sb.append(String.format(" numberOfEmployees: %s\n", numberOfEmployeesString));
String purchasingTimeFrameString = JsonUtil.Stringify(purchasingTimeFrame);
if (purchasingTimeFrameString != null && !purchasingTimeFrameString.isEmpty())
sb.append(String.format(" purchasingTimeFrame: %s\n", purchasingTimeFrameString));
String purchasingRoleString = JsonUtil.Stringify(purchasingRole);
if (purchasingRoleString != null && !purchasingRoleString.isEmpty())
sb.append(String.format(" purchasingRole: %s\n", purchasingRoleString));
String responsesString = JsonUtil.Stringify(responses);
if (responsesString != null && !responsesString.isEmpty())
sb.append(String.format(" responses: %s\n", responsesString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy