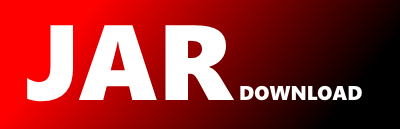
com.logmein.gotowebinar_2_0.api.model.RegistrantQAResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Describes a completed registration question for a webinar registrant. If you use 'Multiple choice' questions the response contains the numeric answerKey, otherwise the answer text. Example:
{
\"firstName\": \"First\",
\"lastName\": \"Last\",
\"email\": \"[email protected]\",
\"responses\": [{
\"questionKey\": 17023549,
\"responseText\": \"This is a short answer\"},{
\"questionKey\": 17023550,
\"answerKey\": 17023553} ]
}
*/
public class RegistrantQAResponse {
/* The unique key of the question */
private Long questionKey = null;
/* Answer of the question. */
private String responseText = null;
/* The numeric key of the answer to a multiple-choice question. */
private Long answerKey = null;
/**
* @return The unique key of the question
*/
public Long getQuestionKey() {
return questionKey;
}
/**
* @param questionKey The unique key of the question
*/
public void setQuestionKey(Long questionKey) {
this.questionKey = questionKey;
}
/**
* @return Answer of the question.
*/
public String getResponseText() {
return responseText;
}
/**
* @param responseText Answer of the question.
*/
public void setResponseText(String responseText) {
this.responseText = responseText;
}
/**
* @return The numeric key of the answer to a multiple-choice question.
*/
public Long getAnswerKey() {
return answerKey;
}
/**
* @param answerKey The numeric key of the answer to a multiple-choice question.
*/
public void setAnswerKey(Long answerKey) {
this.answerKey = answerKey;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RegistrantQAResponse {\n");
String questionKeyString = JsonUtil.Stringify(questionKey);
if (questionKeyString != null && !questionKeyString.isEmpty())
sb.append(String.format(" questionKey: %s\n", questionKeyString));
String responseTextString = JsonUtil.Stringify(responseText);
if (responseTextString != null && !responseTextString.isEmpty())
sb.append(String.format(" responseText: %s\n", responseTextString));
String answerKeyString = JsonUtil.Stringify(answerKey);
if (answerKeyString != null && !answerKeyString.isEmpty())
sb.append(String.format(" answerKey: %s\n", answerKeyString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy