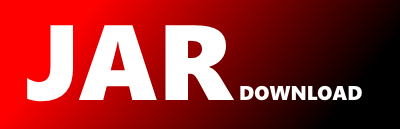
com.logmein.gotowebinar_2_0.api.model.RegistrationQuestion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import com.logmein.gotowebinar_2_0.api.model.RegistrationAnswer;
import java.util.ArrayList;
import java.util.List;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Describes a custom field for a webinar registration.
*/
public class RegistrationQuestion {
/* The unique key of the custom registration field */
private Long questionKey = null;
/* The value (text) of the custom registration field */
private String question = null;
/* Indicates whether the custom registration field is compulsory */
private Boolean required = null;
/* Indicates whether the custom registration field requires a single short answer or whether it is a multiple choice question */
private TypeEnum type = null;
/* The character size of the custom registration field (max 1000) */
private Integer maxSize = null;
/* The answers to a multiple choice custom registration field */
private List answers = new ArrayList();
/**
* Indicates whether the custom registration field requires a single short answer or whether it is a multiple choice question
*/
public enum TypeEnum { multipleChoice, shortAnswer }
/**
* @return The unique key of the custom registration field
*/
public Long getQuestionKey() {
return questionKey;
}
/**
* @param questionKey The unique key of the custom registration field
*/
public void setQuestionKey(Long questionKey) {
this.questionKey = questionKey;
}
/**
* @return The value (text) of the custom registration field
*/
public String getQuestion() {
return question;
}
/**
* @param question The value (text) of the custom registration field
*/
public void setQuestion(String question) {
this.question = question;
}
/**
* @return Indicates whether the custom registration field is compulsory
*/
public Boolean getRequired() {
return required;
}
/**
* @param required Indicates whether the custom registration field is compulsory
*/
public void setRequired(Boolean required) {
this.required = required;
}
/**
* @return Indicates whether the custom registration field requires a single short answer or whether it is a multiple choice question
*/
public TypeEnum getType() {
return type;
}
/**
* @param type Indicates whether the custom registration field requires a single short answer or whether it is a multiple choice question
*/
public void setType(TypeEnum type) {
this.type = type;
}
/**
* @return The character size of the custom registration field (max 1000)
*/
public Integer getMaxSize() {
return maxSize;
}
/**
* @param maxSize The character size of the custom registration field (max 1000)
*/
public void setMaxSize(Integer maxSize) {
this.maxSize = maxSize;
}
/**
* @return The answers to a multiple choice custom registration field
*/
public List getAnswers() {
return answers;
}
/**
* @param answers The answers to a multiple choice custom registration field
*/
public void setAnswers(List answers) {
this.answers = answers;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RegistrationQuestion {\n");
String questionKeyString = JsonUtil.Stringify(questionKey);
if (questionKeyString != null && !questionKeyString.isEmpty())
sb.append(String.format(" questionKey: %s\n", questionKeyString));
String questionString = JsonUtil.Stringify(question);
if (questionString != null && !questionString.isEmpty())
sb.append(String.format(" question: %s\n", questionString));
String requiredString = JsonUtil.Stringify(required);
if (requiredString != null && !requiredString.isEmpty())
sb.append(String.format(" required: %s\n", requiredString));
String typeString = JsonUtil.Stringify(type);
if (typeString != null && !typeString.isEmpty())
sb.append(String.format(" type: %s\n", typeString));
String maxSizeString = JsonUtil.Stringify(maxSize);
if (maxSizeString != null && !maxSizeString.isEmpty())
sb.append(String.format(" maxSize: %s\n", maxSizeString));
String answersString = JsonUtil.Stringify(answers);
if (answersString != null && !answersString.isEmpty())
sb.append(String.format(" answers: %s\n", answersString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy