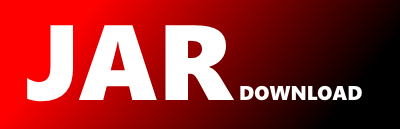
com.logmein.gotowebinar_2_0.api.model.WebinarByKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import com.logmein.gotowebinar_2_0.api.model.DateTimeRange;
import java.util.ArrayList;
import java.util.List;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Describes a webinar
*/
public class WebinarByKey {
/* The unique key of the webinar */
private Long webinarKey = null;
/* The 9-digit webinar ID */
private String webinarID = null;
/* The webinar subject. */
private String subject = null;
/* A short description of the webinar */
private String description = null;
/* The key of the webinar organizer */
private Long organizerKey = null;
/* The email of the webinar organizer */
private String organizerEmail = null;
/* The name of the webinar organizer */
private String organizerName = null;
/* Array with startTime and endTime for the webinar sessions */
private List times = new ArrayList();
/* The URL the webinar invitees can use to register */
private String registrationUrl = null;
/* Indicates whether there is a webinar session currently in progress */
private Boolean inSession = null;
/* A boolean flag indicating if the webinar type is impromptu */
private Boolean impromptu = null;
/* Specifies the recurrence type. The possible values are \"single_session\", \"series\" and \"sequence\". */
private String type = null;
/* The timezone where the webinar is taking place */
private String timeZone = null;
/* The number of registrants at the webinar */
private Integer numberOfRegistrants = null;
/* The maximum number of registrants a webinar can have */
private Integer registrationLimit = null;
/* The webinar language */
private LocaleEnum locale = null;
/* The key of the account */
private String accountKey = null;
/* Specifies the recurrence type. The possible values are \"single_session\", \"series\" and \"sequence\". */
private String recurrencePeriod = null;
/* Specifies the experience type. The possible values are \"classic\", \"broadcast\" and \"simulive\". */
private String experienceType = null;
/* A boolean flag indicating if the webinar is password protected */
private Boolean isPasswordProtected = null;
/**
* The webinar language
*/
public enum LocaleEnum { en_US, de_DE, es_ES, fr_FR, it_IT, zh_CN }
/**
* @return The unique key of the webinar
*/
public Long getWebinarKey() {
return webinarKey;
}
/**
* @param webinarKey The unique key of the webinar
*/
public void setWebinarKey(Long webinarKey) {
this.webinarKey = webinarKey;
}
/**
* @return The 9-digit webinar ID
*/
public String getWebinarID() {
return webinarID;
}
/**
* @param webinarID The 9-digit webinar ID
*/
public void setWebinarID(String webinarID) {
this.webinarID = webinarID;
}
/**
* @return The webinar subject.
*/
public String getSubject() {
return subject;
}
/**
* @param subject The webinar subject.
*/
public void setSubject(String subject) {
this.subject = subject;
}
/**
* @return A short description of the webinar
*/
public String getDescription() {
return description;
}
/**
* @param description A short description of the webinar
*/
public void setDescription(String description) {
this.description = description;
}
/**
* @return The key of the webinar organizer
*/
public Long getOrganizerKey() {
return organizerKey;
}
/**
* @param organizerKey The key of the webinar organizer
*/
public void setOrganizerKey(Long organizerKey) {
this.organizerKey = organizerKey;
}
/**
* @return The email of the webinar organizer
*/
public String getOrganizerEmail() {
return organizerEmail;
}
/**
* @param organizerEmail The email of the webinar organizer
*/
public void setOrganizerEmail(String organizerEmail) {
this.organizerEmail = organizerEmail;
}
/**
* @return The name of the webinar organizer
*/
public String getOrganizerName() {
return organizerName;
}
/**
* @param organizerName The name of the webinar organizer
*/
public void setOrganizerName(String organizerName) {
this.organizerName = organizerName;
}
/**
* @return Array with startTime and endTime for the webinar sessions
*/
public List getTimes() {
return times;
}
/**
* @param times Array with startTime and endTime for the webinar sessions
*/
public void setTimes(List times) {
this.times = times;
}
/**
* @return The URL the webinar invitees can use to register
*/
public String getRegistrationUrl() {
return registrationUrl;
}
/**
* @param registrationUrl The URL the webinar invitees can use to register
*/
public void setRegistrationUrl(String registrationUrl) {
this.registrationUrl = registrationUrl;
}
/**
* @return Indicates whether there is a webinar session currently in progress
*/
public Boolean getInSession() {
return inSession;
}
/**
* @param inSession Indicates whether there is a webinar session currently in progress
*/
public void setInSession(Boolean inSession) {
this.inSession = inSession;
}
/**
* @return A boolean flag indicating if the webinar type is impromptu
*/
public Boolean getImpromptu() {
return impromptu;
}
/**
* @param impromptu A boolean flag indicating if the webinar type is impromptu
*/
public void setImpromptu(Boolean impromptu) {
this.impromptu = impromptu;
}
/**
* @return Specifies the recurrence type. The possible values are \"single_session\", \"series\" and \"sequence\".
*/
public String getType() {
return type;
}
/**
* @param type Specifies the recurrence type. The possible values are \"single_session\", \"series\" and \"sequence\".
*/
public void setType(String type) {
this.type = type;
}
/**
* @return The timezone where the webinar is taking place
*/
public String getTimeZone() {
return timeZone;
}
/**
* @param timeZone The timezone where the webinar is taking place
*/
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
/**
* @return The number of registrants at the webinar
*/
public Integer getNumberOfRegistrants() {
return numberOfRegistrants;
}
/**
* @param numberOfRegistrants The number of registrants at the webinar
*/
public void setNumberOfRegistrants(Integer numberOfRegistrants) {
this.numberOfRegistrants = numberOfRegistrants;
}
/**
* @return The maximum number of registrants a webinar can have
*/
public Integer getRegistrationLimit() {
return registrationLimit;
}
/**
* @param registrationLimit The maximum number of registrants a webinar can have
*/
public void setRegistrationLimit(Integer registrationLimit) {
this.registrationLimit = registrationLimit;
}
/**
* @return The webinar language
*/
public LocaleEnum getLocale() {
return locale;
}
/**
* @param locale The webinar language
*/
public void setLocale(LocaleEnum locale) {
this.locale = locale;
}
/**
* @return The key of the account
*/
public String getAccountKey() {
return accountKey;
}
/**
* @param accountKey The key of the account
*/
public void setAccountKey(String accountKey) {
this.accountKey = accountKey;
}
/**
* @return Specifies the recurrence type. The possible values are \"single_session\", \"series\" and \"sequence\".
*/
public String getRecurrencePeriod() {
return recurrencePeriod;
}
/**
* @param recurrencePeriod Specifies the recurrence type. The possible values are \"single_session\", \"series\" and \"sequence\".
*/
public void setRecurrencePeriod(String recurrencePeriod) {
this.recurrencePeriod = recurrencePeriod;
}
/**
* @return Specifies the experience type. The possible values are \"classic\", \"broadcast\" and \"simulive\".
*/
public String getExperienceType() {
return experienceType;
}
/**
* @param experienceType Specifies the experience type. The possible values are \"classic\", \"broadcast\" and \"simulive\".
*/
public void setExperienceType(String experienceType) {
this.experienceType = experienceType;
}
/**
* @return A boolean flag indicating if the webinar is password protected
*/
public Boolean getIsPasswordProtected() {
return isPasswordProtected;
}
/**
* @param isPasswordProtected A boolean flag indicating if the webinar is password protected
*/
public void setIsPasswordProtected(Boolean isPasswordProtected) {
this.isPasswordProtected = isPasswordProtected;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WebinarByKey {\n");
String webinarKeyString = JsonUtil.Stringify(webinarKey);
if (webinarKeyString != null && !webinarKeyString.isEmpty())
sb.append(String.format(" webinarKey: %s\n", webinarKeyString));
String webinarIDString = JsonUtil.Stringify(webinarID);
if (webinarIDString != null && !webinarIDString.isEmpty())
sb.append(String.format(" webinarID: %s\n", webinarIDString));
String subjectString = JsonUtil.Stringify(subject);
if (subjectString != null && !subjectString.isEmpty())
sb.append(String.format(" subject: %s\n", subjectString));
String descriptionString = JsonUtil.Stringify(description);
if (descriptionString != null && !descriptionString.isEmpty())
sb.append(String.format(" description: %s\n", descriptionString));
String organizerKeyString = JsonUtil.Stringify(organizerKey);
if (organizerKeyString != null && !organizerKeyString.isEmpty())
sb.append(String.format(" organizerKey: %s\n", organizerKeyString));
String organizerEmailString = JsonUtil.Stringify(organizerEmail);
if (organizerEmailString != null && !organizerEmailString.isEmpty())
sb.append(String.format(" organizerEmail: %s\n", organizerEmailString));
String organizerNameString = JsonUtil.Stringify(organizerName);
if (organizerNameString != null && !organizerNameString.isEmpty())
sb.append(String.format(" organizerName: %s\n", organizerNameString));
String timesString = JsonUtil.Stringify(times);
if (timesString != null && !timesString.isEmpty())
sb.append(String.format(" times: %s\n", timesString));
String registrationUrlString = JsonUtil.Stringify(registrationUrl);
if (registrationUrlString != null && !registrationUrlString.isEmpty())
sb.append(String.format(" registrationUrl: %s\n", registrationUrlString));
String inSessionString = JsonUtil.Stringify(inSession);
if (inSessionString != null && !inSessionString.isEmpty())
sb.append(String.format(" inSession: %s\n", inSessionString));
String impromptuString = JsonUtil.Stringify(impromptu);
if (impromptuString != null && !impromptuString.isEmpty())
sb.append(String.format(" impromptu: %s\n", impromptuString));
String typeString = JsonUtil.Stringify(type);
if (typeString != null && !typeString.isEmpty())
sb.append(String.format(" type: %s\n", typeString));
String timeZoneString = JsonUtil.Stringify(timeZone);
if (timeZoneString != null && !timeZoneString.isEmpty())
sb.append(String.format(" timeZone: %s\n", timeZoneString));
String numberOfRegistrantsString = JsonUtil.Stringify(numberOfRegistrants);
if (numberOfRegistrantsString != null && !numberOfRegistrantsString.isEmpty())
sb.append(String.format(" numberOfRegistrants: %s\n", numberOfRegistrantsString));
String registrationLimitString = JsonUtil.Stringify(registrationLimit);
if (registrationLimitString != null && !registrationLimitString.isEmpty())
sb.append(String.format(" registrationLimit: %s\n", registrationLimitString));
String localeString = JsonUtil.Stringify(locale);
if (localeString != null && !localeString.isEmpty())
sb.append(String.format(" locale: %s\n", localeString));
String accountKeyString = JsonUtil.Stringify(accountKey);
if (accountKeyString != null && !accountKeyString.isEmpty())
sb.append(String.format(" accountKey: %s\n", accountKeyString));
String recurrencePeriodString = JsonUtil.Stringify(recurrencePeriod);
if (recurrencePeriodString != null && !recurrencePeriodString.isEmpty())
sb.append(String.format(" recurrencePeriod: %s\n", recurrencePeriodString));
String experienceTypeString = JsonUtil.Stringify(experienceType);
if (experienceTypeString != null && !experienceTypeString.isEmpty())
sb.append(String.format(" experienceType: %s\n", experienceTypeString));
String isPasswordProtectedString = JsonUtil.Stringify(isPasswordProtected);
if (isPasswordProtectedString != null && !isPasswordProtectedString.isEmpty())
sb.append(String.format(" isPasswordProtected: %s\n", isPasswordProtectedString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy