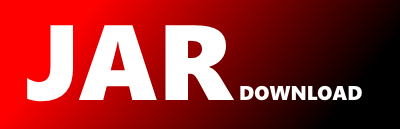
com.logmein.gotowebinar_2_0.api.model.WebinarReqCreate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import com.logmein.gotowebinar_2_0.api.model.DateTimeRange;
import java.util.ArrayList;
import java.util.List;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Describes the details used to create a new single session webinar.
*/
public class WebinarReqCreate {
/* The name/subject of the webinar (128 characters maximum) */
private String subject = null;
/* A short description of the webinar (2048 characters maximum) */
private String description = null;
/* Array with startTime and endTime for webinar. Since this call creates single session webinars, the array can only contain a single pair of startTime and endTime */
private List times = new ArrayList();
/* The time zone where the webinar is taking place (must be a valid time zone ID, see https://goto-developer.logmein.com/time-zones). If this parameter is not passed, the timezone of the organizer's profile will be used */
private String timeZone = null;
/* Specifies the webinar type. The default type value is \"single_session\", which is used to create a single webinar session. The possible values are \"single_session\", \"series\", \"sequence\". If type is set to \"single_session\", a single webinar session is created. If type is set to \"series\", a webinar series is created. In this case 2 or more timeframes must be specified for each webinar. Example: \"times\": [{\"startTime\": \"...\", \"endTime\": \"...\"},{\"startTime\": \"...\", \"endTime\": \"...\"},{\"startTime\": \"...\", \"endTime\": \"...\"}. If type is set to \"sequence\" a sequence of webinars is created. The times object in the body must be replaced by the \"recurrenceStart\" object. Example: \"recurrenceStart\": {\"startTime\":\"2012-06-12T16:00:00Z\", \"endTime\": \"2012-06-12T17:00:00Z\" }. The \"recurrenceEnd\" and \"recurrencePattern\" body parameter must be specified. Example: , \"recurrenceEnd\": \"2012-07-10\", \"recurrencePattern\": \"daily\". */
private String type = "single_session";
/* A boolean flag indicating if the webinar is password protected or not. */
private Boolean isPasswordProtected = false;
/* The recording asset with which the simulive webinar should be created from. In case the recordingasset was created as an online recording the simulive webinar settings, poll and surveys would be copied from the webinar whose session was recorded. */
private String recordingAssetKey = null;
/* A boolean flag indicating if the webinar should be ondemand */
private Boolean isOndemand = false;
/* Specifies the experience type. The possible values are \"CLASSIC\", \"BROADCAST\" and \"SIMULIVE\". */
private String experienceType = "CLASSIC";
/**
* @return The name/subject of the webinar (128 characters maximum)
*/
public String getSubject() {
return subject;
}
/**
* @param subject The name/subject of the webinar (128 characters maximum)
*/
public void setSubject(String subject) {
this.subject = subject;
}
/**
* @return A short description of the webinar (2048 characters maximum)
*/
public String getDescription() {
return description;
}
/**
* @param description A short description of the webinar (2048 characters maximum)
*/
public void setDescription(String description) {
this.description = description;
}
/**
* @return Array with startTime and endTime for webinar. Since this call creates single session webinars, the array can only contain a single pair of startTime and endTime
*/
public List getTimes() {
return times;
}
/**
* @param times Array with startTime and endTime for webinar. Since this call creates single session webinars, the array can only contain a single pair of startTime and endTime
*/
public void setTimes(List times) {
this.times = times;
}
/**
* @return The time zone where the webinar is taking place (must be a valid time zone ID, see https://goto-developer.logmein.com/time-zones). If this parameter is not passed, the timezone of the organizer's profile will be used
*/
public String getTimeZone() {
return timeZone;
}
/**
* @param timeZone The time zone where the webinar is taking place (must be a valid time zone ID, see https://goto-developer.logmein.com/time-zones). If this parameter is not passed, the timezone of the organizer's profile will be used
*/
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
/**
* @return Specifies the webinar type. The default type value is \"single_session\", which is used to create a single webinar session. The possible values are \"single_session\", \"series\", \"sequence\". If type is set to \"single_session\", a single webinar session is created. If type is set to \"series\", a webinar series is created. In this case 2 or more timeframes must be specified for each webinar. Example: \"times\": [{\"startTime\": \"...\", \"endTime\": \"...\"},{\"startTime\": \"...\", \"endTime\": \"...\"},{\"startTime\": \"...\", \"endTime\": \"...\"}. If type is set to \"sequence\" a sequence of webinars is created. The times object in the body must be replaced by the \"recurrenceStart\" object. Example: \"recurrenceStart\": {\"startTime\":\"2012-06-12T16:00:00Z\", \"endTime\": \"2012-06-12T17:00:00Z\" }. The \"recurrenceEnd\" and \"recurrencePattern\" body parameter must be specified. Example: , \"recurrenceEnd\": \"2012-07-10\", \"recurrencePattern\": \"daily\".
*/
public String getType() {
return type;
}
/**
* @param type Specifies the webinar type. The default type value is \"single_session\", which is used to create a single webinar session. The possible values are \"single_session\", \"series\", \"sequence\". If type is set to \"single_session\", a single webinar session is created. If type is set to \"series\", a webinar series is created. In this case 2 or more timeframes must be specified for each webinar. Example: \"times\": [{\"startTime\": \"...\", \"endTime\": \"...\"},{\"startTime\": \"...\", \"endTime\": \"...\"},{\"startTime\": \"...\", \"endTime\": \"...\"}. If type is set to \"sequence\" a sequence of webinars is created. The times object in the body must be replaced by the \"recurrenceStart\" object. Example: \"recurrenceStart\": {\"startTime\":\"2012-06-12T16:00:00Z\", \"endTime\": \"2012-06-12T17:00:00Z\" }. The \"recurrenceEnd\" and \"recurrencePattern\" body parameter must be specified. Example: , \"recurrenceEnd\": \"2012-07-10\", \"recurrencePattern\": \"daily\".
*/
public void setType(String type) {
this.type = type;
}
/**
* @return A boolean flag indicating if the webinar is password protected or not.
*/
public Boolean getIsPasswordProtected() {
return isPasswordProtected;
}
/**
* @param isPasswordProtected A boolean flag indicating if the webinar is password protected or not.
*/
public void setIsPasswordProtected(Boolean isPasswordProtected) {
this.isPasswordProtected = isPasswordProtected;
}
/**
* @return The recording asset with which the simulive webinar should be created from. In case the recordingasset was created as an online recording the simulive webinar settings, poll and surveys would be copied from the webinar whose session was recorded.
*/
public String getRecordingAssetKey() {
return recordingAssetKey;
}
/**
* @param recordingAssetKey The recording asset with which the simulive webinar should be created from. In case the recordingasset was created as an online recording the simulive webinar settings, poll and surveys would be copied from the webinar whose session was recorded.
*/
public void setRecordingAssetKey(String recordingAssetKey) {
this.recordingAssetKey = recordingAssetKey;
}
/**
* @return A boolean flag indicating if the webinar should be ondemand
*/
public Boolean getIsOndemand() {
return isOndemand;
}
/**
* @param isOndemand A boolean flag indicating if the webinar should be ondemand
*/
public void setIsOndemand(Boolean isOndemand) {
this.isOndemand = isOndemand;
}
/**
* @return Specifies the experience type. The possible values are \"CLASSIC\", \"BROADCAST\" and \"SIMULIVE\".
*/
public String getExperienceType() {
return experienceType;
}
/**
* @param experienceType Specifies the experience type. The possible values are \"CLASSIC\", \"BROADCAST\" and \"SIMULIVE\".
*/
public void setExperienceType(String experienceType) {
this.experienceType = experienceType;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WebinarReqCreate {\n");
String subjectString = JsonUtil.Stringify(subject);
if (subjectString != null && !subjectString.isEmpty())
sb.append(String.format(" subject: %s\n", subjectString));
String descriptionString = JsonUtil.Stringify(description);
if (descriptionString != null && !descriptionString.isEmpty())
sb.append(String.format(" description: %s\n", descriptionString));
String timesString = JsonUtil.Stringify(times);
if (timesString != null && !timesString.isEmpty())
sb.append(String.format(" times: %s\n", timesString));
String timeZoneString = JsonUtil.Stringify(timeZone);
if (timeZoneString != null && !timeZoneString.isEmpty())
sb.append(String.format(" timeZone: %s\n", timeZoneString));
String typeString = JsonUtil.Stringify(type);
if (typeString != null && !typeString.isEmpty())
sb.append(String.format(" type: %s\n", typeString));
String isPasswordProtectedString = JsonUtil.Stringify(isPasswordProtected);
if (isPasswordProtectedString != null && !isPasswordProtectedString.isEmpty())
sb.append(String.format(" isPasswordProtected: %s\n", isPasswordProtectedString));
String recordingAssetKeyString = JsonUtil.Stringify(recordingAssetKey);
if (recordingAssetKeyString != null && !recordingAssetKeyString.isEmpty())
sb.append(String.format(" recordingAssetKey: %s\n", recordingAssetKeyString));
String isOndemandString = JsonUtil.Stringify(isOndemand);
if (isOndemandString != null && !isOndemandString.isEmpty())
sb.append(String.format(" isOndemand: %s\n", isOndemandString));
String experienceTypeString = JsonUtil.Stringify(experienceType);
if (experienceTypeString != null && !experienceTypeString.isEmpty())
sb.append(String.format(" experienceType: %s\n", experienceTypeString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy