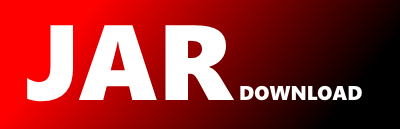
com.logmein.gotowebinar_2_0.api.model.WebinarReqUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar_2_0.api.model;
import com.logmein.gotowebinar_2_0.api.model.DateTimeRange;
import java.util.ArrayList;
import java.util.List;
import com.logmein.gotowebinar_2_0.api.common.JsonUtil;
/**
* Describes the details of the webinar
*/
public class WebinarReqUpdate {
/* The name/subject of the webinar (128 characters maximum) */
private String subject = null;
/* A description of the webinar (2048 characters maximum) */
private String description = null;
/* Array with start and end time(s) for webinar */
private List times = new ArrayList();
/* The time zone where the webinar is taking place (must be a valid time zone ID, see https://goto-developer.logmein.com/time-zones). If this parameter is not passed, the timezone of the organizer's profile will be used */
private String timeZone = null;
/* The webinar language */
private LocaleEnum locale = null;
/**
* The webinar language
*/
public enum LocaleEnum { en_US, de_DE, es_ES, fr_FR, it_IT, zh_CN }
/**
* @return The name/subject of the webinar (128 characters maximum)
*/
public String getSubject() {
return subject;
}
/**
* @param subject The name/subject of the webinar (128 characters maximum)
*/
public void setSubject(String subject) {
this.subject = subject;
}
/**
* @return A description of the webinar (2048 characters maximum)
*/
public String getDescription() {
return description;
}
/**
* @param description A description of the webinar (2048 characters maximum)
*/
public void setDescription(String description) {
this.description = description;
}
/**
* @return Array with start and end time(s) for webinar
*/
public List getTimes() {
return times;
}
/**
* @param times Array with start and end time(s) for webinar
*/
public void setTimes(List times) {
this.times = times;
}
/**
* @return The time zone where the webinar is taking place (must be a valid time zone ID, see https://goto-developer.logmein.com/time-zones). If this parameter is not passed, the timezone of the organizer's profile will be used
*/
public String getTimeZone() {
return timeZone;
}
/**
* @param timeZone The time zone where the webinar is taking place (must be a valid time zone ID, see https://goto-developer.logmein.com/time-zones). If this parameter is not passed, the timezone of the organizer's profile will be used
*/
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
/**
* @return The webinar language
*/
public LocaleEnum getLocale() {
return locale;
}
/**
* @param locale The webinar language
*/
public void setLocale(LocaleEnum locale) {
this.locale = locale;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WebinarReqUpdate {\n");
String subjectString = JsonUtil.Stringify(subject);
if (subjectString != null && !subjectString.isEmpty())
sb.append(String.format(" subject: %s\n", subjectString));
String descriptionString = JsonUtil.Stringify(description);
if (descriptionString != null && !descriptionString.isEmpty())
sb.append(String.format(" description: %s\n", descriptionString));
String timesString = JsonUtil.Stringify(times);
if (timesString != null && !timesString.isEmpty())
sb.append(String.format(" times: %s\n", timesString));
String timeZoneString = JsonUtil.Stringify(timeZone);
if (timeZoneString != null && !timeZoneString.isEmpty())
sb.append(String.format(" timeZone: %s\n", timeZoneString));
String localeString = JsonUtil.Stringify(locale);
if (localeString != null && !localeString.isEmpty())
sb.append(String.format(" locale: %s\n", localeString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy