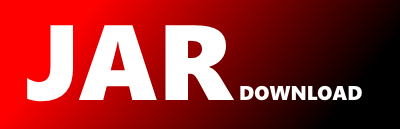
com.logmein.gotowebinar.api.model.PollsAndSurveysStatistics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar.api.model;
import com.logmein.gotowebinar.api.common.JsonUtil;
/**
* Details on the polls and surveys for a webinar session.
*/
public class PollsAndSurveysStatistics {
/* The number of polls launched at a webinar session */
private Integer pollCount = null;
/* The percentage of surveys launched at a webinar session */
private Float surveyCount = null;
/* The number of questions asked at a webinar session */
private Integer questionsAsked = null;
/* The percentage of polls completed by the attendees */
private Float percentagePollsCompleted = null;
/* The percentage of surveys completed by the attendees */
private Float percentageSurveysCompleted = null;
/**
* @return The number of polls launched at a webinar session
*/
public Integer getPollCount() {
return pollCount;
}
/**
* @param pollCount The number of polls launched at a webinar session
*/
public void setPollCount(Integer pollCount) {
this.pollCount = pollCount;
}
/**
* @return The percentage of surveys launched at a webinar session
*/
public Float getSurveyCount() {
return surveyCount;
}
/**
* @param surveyCount The percentage of surveys launched at a webinar session
*/
public void setSurveyCount(Float surveyCount) {
this.surveyCount = surveyCount;
}
/**
* @return The number of questions asked at a webinar session
*/
public Integer getQuestionsAsked() {
return questionsAsked;
}
/**
* @param questionsAsked The number of questions asked at a webinar session
*/
public void setQuestionsAsked(Integer questionsAsked) {
this.questionsAsked = questionsAsked;
}
/**
* @return The percentage of polls completed by the attendees
*/
public Float getPercentagePollsCompleted() {
return percentagePollsCompleted;
}
/**
* @param percentagePollsCompleted The percentage of polls completed by the attendees
*/
public void setPercentagePollsCompleted(Float percentagePollsCompleted) {
this.percentagePollsCompleted = percentagePollsCompleted;
}
/**
* @return The percentage of surveys completed by the attendees
*/
public Float getPercentageSurveysCompleted() {
return percentageSurveysCompleted;
}
/**
* @param percentageSurveysCompleted The percentage of surveys completed by the attendees
*/
public void setPercentageSurveysCompleted(Float percentageSurveysCompleted) {
this.percentageSurveysCompleted = percentageSurveysCompleted;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PollsAndSurveysStatistics {\n");
String pollCountString = JsonUtil.Stringify(pollCount);
if (pollCountString != null && !pollCountString.isEmpty())
sb.append(String.format(" pollCount: %s\n", pollCountString));
String surveyCountString = JsonUtil.Stringify(surveyCount);
if (surveyCountString != null && !surveyCountString.isEmpty())
sb.append(String.format(" surveyCount: %s\n", surveyCountString));
String questionsAskedString = JsonUtil.Stringify(questionsAsked);
if (questionsAskedString != null && !questionsAskedString.isEmpty())
sb.append(String.format(" questionsAsked: %s\n", questionsAskedString));
String percentagePollsCompletedString = JsonUtil.Stringify(percentagePollsCompleted);
if (percentagePollsCompletedString != null && !percentagePollsCompletedString.isEmpty())
sb.append(String.format(" percentagePollsCompleted: %s\n", percentagePollsCompletedString));
String percentageSurveysCompletedString = JsonUtil.Stringify(percentageSurveysCompleted);
if (percentageSurveysCompletedString != null && !percentageSurveysCompletedString.isEmpty())
sb.append(String.format(" percentageSurveysCompleted: %s\n", percentageSurveysCompletedString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy