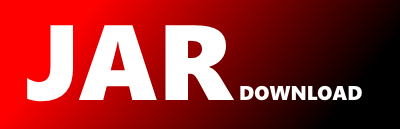
com.logmein.gotowebinar.api.RecordingAssetsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar.api;
import com.logmein.gotowebinar.api.common.ApiException;
import com.logmein.gotowebinar.api.common.ApiInvoker;
import com.logmein.gotowebinar.api.common.JsonUtil;
import com.logmein.gotowebinar.api.model.AssetsResponse;
import com.logmein.gotowebinar.api.model.AssetsSearchReq;
import com.sun.jersey.multipart.FormDataMultiPart;
import org.apache.commons.lang3.StringUtils;
import java.util.HashMap;
import java.util.Map;
public class RecordingAssetsApi {
private String basePath = "https://api.getgo.com/G2W/rest/v2";
private ApiInvoker apiInvoker = ApiInvoker.getInstance();
/**
* Initializes a new instance of the RecordingAssetsApi class using the
* default endpoint base url for the services being accessed.
*/
public RecordingAssetsApi() {
}
/**
* Initializes a new instance of the RecordingAssetsApi class using an endpoint base
* url other than the default. Use this constructor only if you should need
* to override the default endpoint base url.
* @param basePath The endpoint base url for the services being accessed.
*/
public RecordingAssetsApi(String basePath) {
this.basePath = basePath;
}
public ApiInvoker getInvoker() {
return apiInvoker;
}
public String getBasePath() {
return basePath;
}
/**
* Search for completed recordingassets
* Allows search for a completed recordingasset.
* @param authorization Access token
* @param body The asset search parameters
* @param page The page number to be displayed. The first page is 0.
* @param size The size of the page.
* @param includes Add response variables in AssetsResponse
* @return AssetsResponse
* @throws ApiException If the response status code is not Successful 2xx, or an error has occurred during parameter serialization or response deserialization
*/
public AssetsResponse searchAssets(String authorization, AssetsSearchReq body, Long page, Long size, String includes) throws ApiException {
Object postBody = body;
if(authorization == null) {
throw new ApiException("Required parameter authorization is null.");
}
if(body == null) {
throw new ApiException("Required parameter body is null.");
}
// create path and map variables
String path = "/recordingassets/search"
.replaceAll("\\{format\\}", "json");
// query params
Map queryParams = new HashMap();
Map headerParams = new HashMap();
Map formParams = new HashMap();
String pageString = JsonUtil.Stringify(page);
if(!"null".equals(pageString)){
queryParams.put("page", pageString);
}
String sizeString = JsonUtil.Stringify(size);
if(!"null".equals(sizeString)){
queryParams.put("size", sizeString);
}
String includesString = JsonUtil.Stringify(includes);
if(StringUtils.isNotBlank(includesString)){
queryParams.put("includes", includesString);
}
headerParams.put("Authorization", authorization);
String[] contentTypes = {
"application/json"
};
String contentType = contentTypes.length > 0 ? contentTypes[0] : "application/json";
if(contentType.startsWith("multipart/form-data")) {
boolean hasFields = false;
FormDataMultiPart mp = new FormDataMultiPart();
if(hasFields)
postBody = mp;
}
else {
}
String response = apiInvoker.invokeAPI(basePath, path, "POST", queryParams, postBody, headerParams, formParams, contentType);
if(response != null){
return (AssetsResponse) ApiInvoker.deserialize(response, "", AssetsResponse.class);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy