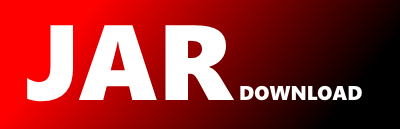
com.logmein.gotowebinar.api.model.CoorganizerReqCreate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar.api.model;
import com.logmein.gotowebinar.api.common.JsonUtil;
/**
* Details used for creating a co-organizer for a webinar.
*/
public class CoorganizerReqCreate {
/* If the co-organizer has no GoToWebinar account, this value has to be set to 'true' */
private Boolean external = null;
/* The co-organizer's organizer key. This parameter has to be passed only, if 'external' is set to 'false' */
private String organizerKey = null;
/* The co-organizer's given name. This parameter has to be passed only, if 'external' is set to 'true' */
private String givenName = null;
/* The co-organizer's email address. This parameter has to be passed only, if 'external' is set to 'true' */
private String email = null;
/**
* @return If the co-organizer has no GoToWebinar account, this value has to be set to 'true'
*/
public Boolean getExternal() {
return external;
}
/**
* @param external If the co-organizer has no GoToWebinar account, this value has to be set to 'true'
*/
public void setExternal(Boolean external) {
this.external = external;
}
/**
* @return The co-organizer's organizer key. This parameter has to be passed only, if 'external' is set to 'false'
*/
public String getOrganizerKey() {
return organizerKey;
}
/**
* @param organizerKey The co-organizer's organizer key. This parameter has to be passed only, if 'external' is set to 'false'
*/
public void setOrganizerKey(String organizerKey) {
this.organizerKey = organizerKey;
}
/**
* @return The co-organizer's given name. This parameter has to be passed only, if 'external' is set to 'true'
*/
public String getGivenName() {
return givenName;
}
/**
* @param givenName The co-organizer's given name. This parameter has to be passed only, if 'external' is set to 'true'
*/
public void setGivenName(String givenName) {
this.givenName = givenName;
}
/**
* @return The co-organizer's email address. This parameter has to be passed only, if 'external' is set to 'true'
*/
public String getEmail() {
return email;
}
/**
* @param email The co-organizer's email address. This parameter has to be passed only, if 'external' is set to 'true'
*/
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CoorganizerReqCreate {\n");
String externalString = JsonUtil.Stringify(external);
if (externalString != null && !externalString.isEmpty())
sb.append(String.format(" external: %s\n", externalString));
String organizerKeyString = JsonUtil.Stringify(organizerKey);
if (organizerKeyString != null && !organizerKeyString.isEmpty())
sb.append(String.format(" organizerKey: %s\n", organizerKeyString));
String givenNameString = JsonUtil.Stringify(givenName);
if (givenNameString != null && !givenNameString.isEmpty())
sb.append(String.format(" givenName: %s\n", givenNameString));
String emailString = JsonUtil.Stringify(email);
if (emailString != null && !emailString.isEmpty())
sb.append(String.format(" email: %s\n", emailString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy