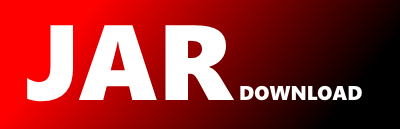
com.logmein.gotowebinar.api.model.ReportingSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gotowebinar-api Show documentation
Show all versions of gotowebinar-api Show documentation
Java SDK for the GoToWebinar REST API
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar.api.model;
import com.logmein.gotowebinar.api.common.JsonUtil;
import java.util.Date;
/**
* Describes a completed webinar session.
*/
public class ReportingSession {
/* The unique key of the webinar session */
private String sessionKey = null;
/* The unique key of the webinar */
private String webinarKey = null;
/* The webinar name */
private String webinarName = null;
/* The starting time of the webinar session */
private Date startTime = null;
/* The ending time of the webinar session */
private Date endTime = null;
/* The number of registrants who attended the webinar session */
private Integer registrantsAttended = null;
/* The total number of registrants for the webinar */
private Integer registrantCount = null;
/* The key of the account */
private String accountKey = null;
/* The key of the webinar organizer who scheduled the webinar */
private String creatingOrganizerKey = null;
/* The name of the webinar organizer who scheduled the webinar */
private String creatingOrganizerName = null;
/* The key of the webinar organizer who started the webinar session */
private String startingOrganizerKey = null;
/* The name of the webinar organizer who started the webinar session */
private String startingOrganizerName = null;
/* The total number of polls for the webinar session */
private Integer totalPollCount = null;
/* The timezone where the webinar is taking place */
private String timeZone = null;
/* Specifies the experience type. The possible values are \"classic\", \"broadcast\" and \"simulive\". */
private String experienceType = null;
/* The 9-digit webinar ID */
private String webinarID = null;
/**
* @return The unique key of the webinar session
*/
public String getSessionKey() {
return sessionKey;
}
/**
* @param sessionKey The unique key of the webinar session
*/
public void setSessionKey(String sessionKey) {
this.sessionKey = sessionKey;
}
/**
* @return The unique key of the webinar
*/
public String getWebinarKey() {
return webinarKey;
}
/**
* @param webinarKey The unique key of the webinar
*/
public void setWebinarKey(String webinarKey) {
this.webinarKey = webinarKey;
}
/**
* @return The webinar name
*/
public String getWebinarName() {
return webinarName;
}
/**
* @param webinarName The webinar name
*/
public void setWebinarName(String webinarName) {
this.webinarName = webinarName;
}
/**
* @return The starting time of the webinar session
*/
public Date getStartTime() {
return startTime;
}
/**
* @param startTime The starting time of the webinar session
*/
public void setStartTime(Date startTime) {
this.startTime = startTime;
}
/**
* @return The ending time of the webinar session
*/
public Date getEndTime() {
return endTime;
}
/**
* @param endTime The ending time of the webinar session
*/
public void setEndTime(Date endTime) {
this.endTime = endTime;
}
/**
* @return The number of registrants who attended the webinar session
*/
public Integer getRegistrantsAttended() {
return registrantsAttended;
}
/**
* @param registrantsAttended The number of registrants who attended the webinar session
*/
public void setRegistrantsAttended(Integer registrantsAttended) {
this.registrantsAttended = registrantsAttended;
}
/**
* @return The total number of registrants for the webinar
*/
public Integer getRegistrantCount() {
return registrantCount;
}
/**
* @param registrantCount The total number of registrants for the webinar
*/
public void setRegistrantCount(Integer registrantCount) {
this.registrantCount = registrantCount;
}
/**
* @return The key of the account
*/
public String getAccountKey() {
return accountKey;
}
/**
* @param accountKey The key of the account
*/
public void setAccountKey(String accountKey) {
this.accountKey = accountKey;
}
/**
* @return The key of the webinar organizer who scheduled the webinar
*/
public String getCreatingOrganizerKey() {
return creatingOrganizerKey;
}
/**
* @param creatingOrganizerKey The key of the webinar organizer who scheduled the webinar
*/
public void setCreatingOrganizerKey(String creatingOrganizerKey) {
this.creatingOrganizerKey = creatingOrganizerKey;
}
/**
* @return The name of the webinar organizer who scheduled the webinar
*/
public String getCreatingOrganizerName() {
return creatingOrganizerName;
}
/**
* @param creatingOrganizerName The name of the webinar organizer who scheduled the webinar
*/
public void setCreatingOrganizerName(String creatingOrganizerName) {
this.creatingOrganizerName = creatingOrganizerName;
}
/**
* @return The key of the webinar organizer who started the webinar session
*/
public String getStartingOrganizerKey() {
return startingOrganizerKey;
}
/**
* @param startingOrganizerKey The key of the webinar organizer who started the webinar session
*/
public void setStartingOrganizerKey(String startingOrganizerKey) {
this.startingOrganizerKey = startingOrganizerKey;
}
/**
* @return The name of the webinar organizer who started the webinar session
*/
public String getStartingOrganizerName() {
return startingOrganizerName;
}
/**
* @param startingOrganizerName The name of the webinar organizer who started the webinar session
*/
public void setStartingOrganizerName(String startingOrganizerName) {
this.startingOrganizerName = startingOrganizerName;
}
/**
* @return The total number of polls for the webinar session
*/
public Integer getTotalPollCount() {
return totalPollCount;
}
/**
* @param totalPollCount The total number of polls for the webinar session
*/
public void setTotalPollCount(Integer totalPollCount) {
this.totalPollCount = totalPollCount;
}
/**
* @return The timezone where the webinar is taking place
*/
public String getTimeZone() {
return timeZone;
}
/**
* @param timeZone The timezone where the webinar is taking place
*/
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
/**
* @return Specifies the experience type. The possible values are \"classic\", \"broadcast\" and \"simulive\".
*/
public String getExperienceType() {
return experienceType;
}
/**
* @param experienceType Specifies the experience type. The possible values are \"classic\", \"broadcast\" and \"simulive\".
*/
public void setExperienceType(String experienceType) {
this.experienceType = experienceType;
}
/**
* @return The 9-digit webinar ID
*/
public String getWebinarID() {
return webinarID;
}
/**
* @param webinarID The 9-digit webinar ID
*/
public void setWebinarID(String webinarID) {
this.webinarID = webinarID;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ReportingSession {\n");
String sessionKeyString = JsonUtil.Stringify(sessionKey);
if (sessionKeyString != null && !sessionKeyString.isEmpty())
sb.append(String.format(" sessionKey: %s\n", sessionKeyString));
String webinarKeyString = JsonUtil.Stringify(webinarKey);
if (webinarKeyString != null && !webinarKeyString.isEmpty())
sb.append(String.format(" webinarKey: %s\n", webinarKeyString));
String webinarNameString = JsonUtil.Stringify(webinarName);
if (webinarNameString != null && !webinarNameString.isEmpty())
sb.append(String.format(" webinarName: %s\n", webinarNameString));
String startTimeString = JsonUtil.Stringify(startTime);
if (startTimeString != null && !startTimeString.isEmpty())
sb.append(String.format(" startTime: %s\n", startTimeString));
String endTimeString = JsonUtil.Stringify(endTime);
if (endTimeString != null && !endTimeString.isEmpty())
sb.append(String.format(" endTime: %s\n", endTimeString));
String registrantsAttendedString = JsonUtil.Stringify(registrantsAttended);
if (registrantsAttendedString != null && !registrantsAttendedString.isEmpty())
sb.append(String.format(" registrantsAttended: %s\n", registrantsAttendedString));
String registrantCountString = JsonUtil.Stringify(registrantCount);
if (registrantCountString != null && !registrantCountString.isEmpty())
sb.append(String.format(" registrantCount: %s\n", registrantCountString));
String accountKeyString = JsonUtil.Stringify(accountKey);
if (accountKeyString != null && !accountKeyString.isEmpty())
sb.append(String.format(" accountKey: %s\n", accountKeyString));
String creatingOrganizerKeyString = JsonUtil.Stringify(creatingOrganizerKey);
if (creatingOrganizerKeyString != null && !creatingOrganizerKeyString.isEmpty())
sb.append(String.format(" creatingOrganizerKey: %s\n", creatingOrganizerKeyString));
String creatingOrganizerNameString = JsonUtil.Stringify(creatingOrganizerName);
if (creatingOrganizerNameString != null && !creatingOrganizerNameString.isEmpty())
sb.append(String.format(" creatingOrganizerName: %s\n", creatingOrganizerNameString));
String startingOrganizerKeyString = JsonUtil.Stringify(startingOrganizerKey);
if (startingOrganizerKeyString != null && !startingOrganizerKeyString.isEmpty())
sb.append(String.format(" startingOrganizerKey: %s\n", startingOrganizerKeyString));
String startingOrganizerNameString = JsonUtil.Stringify(startingOrganizerName);
if (startingOrganizerNameString != null && !startingOrganizerNameString.isEmpty())
sb.append(String.format(" startingOrganizerName: %s\n", startingOrganizerNameString));
String totalPollCountString = JsonUtil.Stringify(totalPollCount);
if (totalPollCountString != null && !totalPollCountString.isEmpty())
sb.append(String.format(" totalPollCount: %s\n", totalPollCountString));
String timeZoneString = JsonUtil.Stringify(timeZone);
if (timeZoneString != null && !timeZoneString.isEmpty())
sb.append(String.format(" timeZone: %s\n", timeZoneString));
String experienceTypeString = JsonUtil.Stringify(experienceType);
if (experienceTypeString != null && !experienceTypeString.isEmpty())
sb.append(String.format(" experienceType: %s\n", experienceTypeString));
String webinarIDString = JsonUtil.Stringify(webinarID);
if (webinarIDString != null && !webinarIDString.isEmpty())
sb.append(String.format(" webinarID: %s\n", webinarIDString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy