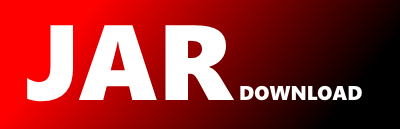
com.logmein.gotowebinar.api.model.WebinarEmailSettings Maven / Gradle / Ivy
/*
* © 2017 LogMeIn, Inc. All Rights Reserved.
* All rights reserved.
*
* This software is distributed under the terms and conditions of the
* LogMeIn SDK License Agreement. Please see file LICENSE for details.
*
* Auto-generated file.
*/
package com.logmein.gotowebinar.api.model;
import com.logmein.gotowebinar.api.common.JsonUtil;
/**
* Describes email settings of a webinar.
*/
public class WebinarEmailSettings {
/* Describes confirmation email settings. */
private EmailSettings confirmationEmail = null;
/* Describes reminder email settings. */
private EmailSettings reminderEmail = null;
/* Describes absentee follow-up email settings. */
private EmailSettings absenteeFollowUpEmail = null;
/* Describes attendee follow-up email settings. */
private AttendeeFollowUpEmailSetting attendeeFollowUpEmail = null;
/**
* @return Describes confirmation email settings.
*/
public EmailSettings getConfirmationEmail() {
return confirmationEmail;
}
/**
* @param confirmationEmail Describes confirmation email settings.
*/
public void setConfirmationEmail(EmailSettings confirmationEmail) {
this.confirmationEmail = confirmationEmail;
}
/**
* @return Describes reminder email settings.
*/
public EmailSettings getReminderEmail() {
return reminderEmail;
}
/**
* @param reminderEmail Describes reminder email settings.
*/
public void setReminderEmail(EmailSettings reminderEmail) {
this.reminderEmail = reminderEmail;
}
/**
* @return Describes absentee follow-up email settings.
*/
public EmailSettings getAbsenteeFollowUpEmail() {
return absenteeFollowUpEmail;
}
/**
* @param absenteeFollowUpEmail Describes absentee follow-up email settings.
*/
public void setAbsenteeFollowUpEmail(EmailSettings absenteeFollowUpEmail) {
this.absenteeFollowUpEmail = absenteeFollowUpEmail;
}
/**
* @return Describes attendee follow-up email settings.
*/
public AttendeeFollowUpEmailSetting getAttendeeFollowUpEmail() {
return attendeeFollowUpEmail;
}
/**
* @param attendeeFollowUpEmail Describes attendee follow-up email settings.
*/
public void setAttendeeFollowUpEmail(AttendeeFollowUpEmailSetting attendeeFollowUpEmail) {
this.attendeeFollowUpEmail = attendeeFollowUpEmail;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class WebinarEmailSettings {\n");
String confirmationEmailString = JsonUtil.Stringify(confirmationEmail);
if (confirmationEmailString != null && !confirmationEmailString.isEmpty())
sb.append(String.format(" confirmationEmail: %s\n", confirmationEmailString));
String reminderEmailString = JsonUtil.Stringify(reminderEmail);
if (reminderEmailString != null && !reminderEmailString.isEmpty())
sb.append(String.format(" reminderEmail: %s\n", reminderEmailString));
String absenteeFollowUpEmailString = JsonUtil.Stringify(absenteeFollowUpEmail);
if (absenteeFollowUpEmailString != null && !absenteeFollowUpEmailString.isEmpty())
sb.append(String.format(" absenteeFollowUpEmail: %s\n", absenteeFollowUpEmailString));
String attendeeFollowUpEmailString = JsonUtil.Stringify(attendeeFollowUpEmail);
if (attendeeFollowUpEmailString != null && !attendeeFollowUpEmailString.isEmpty())
sb.append(String.format(" attendeeFollowUpEmail: %s\n", attendeeFollowUpEmailString));
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy