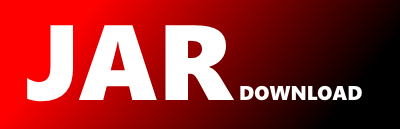
com.lonepulse.zombielink.executor.AsyncExecutionHandler Maven / Gradle / Ivy
The newest version!
package com.lonepulse.zombielink.executor;
/*
* #%L
* ZombieLink
* %%
* Copyright (C) 2013 - 2014 Lonepulse
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.http.HttpResponse;
import com.lonepulse.zombielink.annotation.Async;
import com.lonepulse.zombielink.processor.Processors;
import com.lonepulse.zombielink.proxy.InvocationContext;
import com.lonepulse.zombielink.proxy.InvocationException;
import com.lonepulse.zombielink.response.AsyncHandler;
/**
* This is an implementation of {@link ExecutionHandler} which manages {@link AsyncHandler}s that
* may be used in asynchronous requests. It should be used with {@link RequestExecutor}s that
* support asynchronous request execution (i.e. those requests annotated with @{@link Async}).
*
* @version 1.1.0
*
* @since 1.3.0
*
* @author Lahiru Sahan Jayasinghe
*/
final class AsyncExecutionHandler implements ExecutionHandler {
private static final Log LOGGER = LogFactory.getLog(AsyncRequestExecutor.class);
@SuppressWarnings("unchecked") //safe cast from Object to AsyncHandler
private static AsyncHandler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy