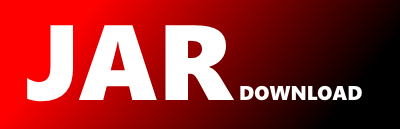
com.loocme.sys.datastruct.WeekSimple Maven / Gradle / Ivy
package com.loocme.sys.datastruct;
import java.util.Date;
import java.util.List;
import java.util.Map;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.loocme.sys.constance.DateFormatConst;
import com.loocme.sys.util.DateUtil;
/**
* @author liuchi
*/
class WeekSimple extends Var implements java.io.Serializable
{
private static final long serialVersionUID = 1L;
private transient Object priValue = null;
public WeekSimple()
{
super();
}
public WeekSimple(Object value)
{
super();
this.setValue(value);
}
@SuppressWarnings("unchecked")
void setValue(Object value)
{
if (null == value)
{
return;
}
if (value instanceof List)
{
JSONArray arr = new JSONArray((List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy