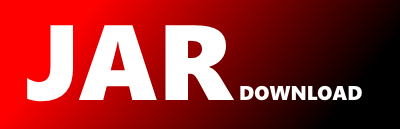
com.loocme.sys.udp.SocketClient Maven / Gradle / Ivy
package com.loocme.sys.udp;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
import java.net.*;
@Slf4j
public class SocketClient
{
private static DatagramSocket priClient;
private static byte[] priClientLock = new byte[1];
public static void send(String ip, int port, byte[] data)
{
if (null == data || 0 == data.length)
{
return;
}
DatagramSocket client = getPriClient();
if (null == client)
{
return;
}
try
{
DatagramPacket packet = new DatagramPacket(data, data.length, new InetSocketAddress(ip, port));
client.send(packet);
}
catch (IOException e)
{
log.error("upd send data failed, the reason is : ", e);
}
}
public static void broadcast(int port, byte[] data)
{
if (null == data || 0 == data.length)
{
return;
}
DatagramSocket client = getPriClient();
if (null == client)
{
return;
}
try
{
DatagramPacket packet = new DatagramPacket(data, data.length, InetAddress.getByName("255.255.255.255"), port);
client.send(packet);
}
catch (IOException e)
{
log.error("upd send data failed, the reason is : ", e);
}
}
private static DatagramSocket getPriClient()
{
if (null != priClient)
{
return priClient;
}
synchronized (priClientLock)
{
if (null != priClient)
{
return priClient;
}
try
{
priClient = new DatagramSocket();
log.info("start upd client .");
}
catch (SocketException e)
{
log.error("start upd client failed. the reason is : ", e);
priClient = null;
}
}
return priClient;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy