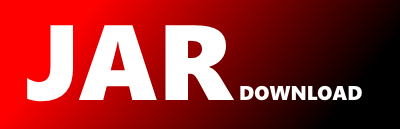
com.loocme.sys.util.AnnotationUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import lombok.extern.slf4j.Slf4j;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
/**
* 注解读取帮助类
*
* @author loocme
*
*/
@Slf4j
public class AnnotationUtil
{
/**
* 获取class类中的特定注解
*
* @param
* 泛型
* @param clz
* 需要获取的class类
* @param annotationClass
* 注解类型
* @return 注解
*/
public static T getAnnotation(Class> clz,
Class annotationClass)
{
T anno = clz.getAnnotation(annotationClass);
if (null == anno)
{
try
{
throw new Exception(String.format("类(%s)上没有定义注解(%s)",
clz.getName(), annotationClass.getName()));
}
catch (Exception e)
{
log.error("", e);
}
}
return anno;
}
/**
* 获取field字段中的特定注解
*
* @param
* 泛型
* @param field
* 需要获取的field字段
* @param annotationClass
* 注解类型
* @return 注解
*/
public static T getAnnotation(Field field,
Class annotationClass)
{
T anno = field.getAnnotation(annotationClass);
if (null == anno)
{
try
{
throw new Exception(String.format("类(%s)中属性(%s)没有定义注解(%s)",
field.getDeclaringClass().getName(), field.getName(),
annotationClass.getName()));
}
catch (Exception e)
{
log.error("", e);
}
}
return anno;
}
public static T getAnnotation(Method method,
Class annotationClass)
{
T anno = method.getAnnotation(annotationClass);
if (null == anno)
{
try
{
throw new Exception(String.format("类(%s)中方法(%s)没有定义注解(%s)",
method.getDeclaringClass().getName(), method.getName(),
annotationClass.getName()));
}
catch (Exception e)
{
log.error("", e);
}
}
return anno;
}
/**
* 判断class中是否包含某种注解
*
* @param clz
* class类
* @param annotationClass
* 注解类型
* @return 是否包含
*/
public static boolean hasAnnotation(Class> clz,
Class extends Annotation> annotationClass)
{
return clz.getAnnotation(annotationClass) == null ? false : true;
}
/**
* 判断field字段中是否包含某种注解
*
* @param field
* field字段
* @param annotationClass
* 注解类型
* @return 是否包含
*/
public static boolean hasAnnotation(Field field,
Class extends Annotation> annotationClass)
{
return field.getAnnotation(annotationClass) == null ? false : true;
}
public static boolean hasAnnotation(Method method,
Class extends Annotation> annotationClass)
{
return method.getAnnotation(annotationClass) == null ? false : true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy