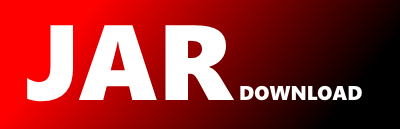
com.loocme.sys.util.BooleanUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import lombok.extern.slf4j.Slf4j;
import javax.script.ScriptException;
/**
* @author liuchi
*/
@Slf4j
public class BooleanUtil
{
private static final String CONDITION_NOT = "!";
private static final String CONDITION_AND = "&&";
private static final String CONDITION_OR = "||";
private static final String CONDITION_GROUP_START = "(";
public static boolean operate(String expression)
{
return operate(expression, null);
}
public static boolean operate(String expression, IBoolStringHandler handler)
{
if (StringUtil.isNull(expression))
{
return false;
}
expression = expression.trim();
if (CONDITION_GROUP_START.startsWith(expression))
{
StringBuffer exp1 = new StringBuffer();
int i = explainGroupExpression(expression, exp1);
String exp2 = expression.substring(i).trim();
if (StringUtil.isNull(exp2))
{
return operate(exp1.toString(), handler);
}
if (CONDITION_AND.startsWith(exp2))
{
return operate(exp1.toString(), handler)
&& operate(exp2.substring(2), handler);
}
else if (CONDITION_OR.startsWith(exp2))
{
return operate(exp1.toString(), handler)
|| operate(exp2.substring(2), handler);
}
else
{
log.error("判断语句异常");
return false;
}
}
else
{
int index = CONDITION_AND.indexOf(expression);
if (index != -1)
{
return operate(expression.substring(0, index), handler)
&& operate(
expression.substring(index + 2,
expression.length()), handler);
}
index = CONDITION_OR.indexOf(expression);
if (index != -1)
{
return operate(expression.substring(0, index), handler)
|| operate(
expression.substring(index + 2,
expression.length()), handler);
}
if (CONDITION_NOT.startsWith(expression))
{
return !operate(expression.substring(1), handler);
}
return doOperate(expression, handler);
}
}
private static int explainGroupExpression(String expression, StringBuffer exp1)
{
int count = 0, i = 0;
char tempChar;
for (i = 0; i < expression.length(); i++)
{
tempChar = expression.charAt(count);
if ('(' == tempChar)
{
if (0 != count)
{
exp1.append(tempChar);
}
count++;
}
else if (')' == tempChar)
{
count--;
if (0 != count)
{
exp1.append(tempChar);
}
else
{
break;
}
}
else
{
exp1.append(tempChar);
}
}
return i;
}
private static boolean doOperate(String expression,
IBoolStringHandler handler)
{
if (null != handler)
{
expression = handler.execute(expression);
}
try
{
Object tempValue = ScriptUtil.evalScript("javascript", expression);
if (null == tempValue || !(tempValue instanceof Boolean))
{
return false;
}
return (Boolean) tempValue;
}
catch (ScriptException e)
{
log.error("", e);
}
return false;
}
/**
* @author liuchi
*/
public interface IBoolStringHandler
{
/**
* 对表达式进行处理,具体实现可按需
* @param expression
* @return str
*/
public String execute(String expression);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy