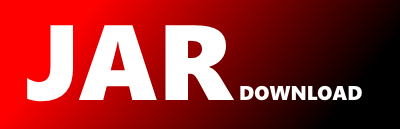
com.loocme.sys.util.ByteUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
/**
* 字节使用帮助类
*
* @author loocme
*
*/
public class ByteUtil
{
private static final int LENGTH_BYTE = 8;
/**
* 字节转换为int
*
* @param b
* 源字节数组
* @param start
* 起始位
* @param len
* 位数长度
* @return 转换后的int值
*/
public static int bytes2Int(byte[] b, int start, int len)
{
int sum = 0;
int end = start + len;
for (int i = start; i < end; i++)
{
int n = ((int) b[i]) & 0xff;
n <<= (--len) * LENGTH_BYTE;
sum = n + sum;
}
return sum;
}
public static int bytes2IntLow(byte[] b, int start, int len)
{
int sum = 0;
int end = start + len;
for (int i = start; i < end; i++)
{
int n = ((int) b[i]) & 0xff;
n <<= (i - start) * LENGTH_BYTE;
sum = n + sum;
}
return sum;
}
/**
* 将int值转换为字节数组
*
* @param value
* int数据
* @param len
* 该数据占多少长度
* @return 转换后的字节数组
*/
public static byte[] int2Byte(int value, int len)
{
byte[] b = new byte[len];
for (int i = 0; i < len; i++)
{
b[len - i - 1] = (byte) ((value >> LENGTH_BYTE * i) & 0xff);
}
return b;
}
public static byte[] int2ByteLow(int value, int len)
{
byte[] b = new byte[len];
for (int i = 0; i < len; i++)
{
b[i] = (byte) ((value >> LENGTH_BYTE * i) & 0xff);
}
return b;
}
/**
* 将字节数组转换成字符串
*
* @param b
* 源字节数组
* @param start
* 起始位置
* @param len
* 该数据所占长度
* @return 转换后的字符串
*/
public static String bytes2String(byte[] b, int start, int len)
{
return new String(b, start, len);
}
/**
* 将字符串转换为字节数组,使用默认字符集
*
* @param str
* 字符串
* @return 字节数组
*/
public static byte[] string2Bytes(String str)
{
return str.getBytes();
}
public static byte[] longToBytes(long values)
{
byte[] buffer = new byte[LENGTH_BYTE];
for (int i = 0; i < LENGTH_BYTE; i++)
{
int offset = 64 - (i + 1) * LENGTH_BYTE;
buffer[i] = (byte) ((values >> offset) & 0xff);
}
return buffer;
}
public static long bytesToLong(byte[] buffer)
{
long values = 0;
for (int i = 0; i < LENGTH_BYTE; i++)
{
values <<= LENGTH_BYTE;
values |= (buffer[i] & 0xff);
}
return values;
}
public static long bytesToLong(byte[] buffer, int start)
{
long values = 0;
for (int i = 0; i < LENGTH_BYTE; i++)
{
values <<= LENGTH_BYTE;
values |= (buffer[start + i] & 0xff);
}
return values;
}
public static byte[] double2Bytes(double d)
{
long value = Double.doubleToRawLongBits(d);
byte[] byteRet = new byte[LENGTH_BYTE];
for (int i = 0; i < LENGTH_BYTE; i++)
{
byteRet[i] = (byte) ((value >> LENGTH_BYTE * i) & 0xff);
}
return byteRet;
}
public static double bytes2Double(byte[] arr)
{
long value = 0;
for (int i = 0; i < LENGTH_BYTE; i++)
{
value |= ((long) (arr[i] & 0xff)) << (LENGTH_BYTE * i);
}
return Double.longBitsToDouble(value);
}
public static double bytes2Double(byte[] arr, int start)
{
long value = 0;
int end = start + LENGTH_BYTE;
for (int i = start; i < end; ++i)
{
value |= ((long) (arr[i] & 0xff)) << (LENGTH_BYTE * (i - start));
}
return Double.longBitsToDouble(value);
}
final private static char[] HEX_ARRAY = "0123456789ABCDEF".toCharArray();
public static String bytesToHex(byte[] bytes)
{
char[] hexChars = new char[bytes.length * 2];
for (int j = 0; j < bytes.length; j++)
{
int v = bytes[j] & 0xFF;
hexChars[j * 2] = HEX_ARRAY[v >>> 4];
hexChars[j * 2 + 1] = HEX_ARRAY[v & 0x0F];
}
return new String(hexChars);
}
public static String bytesToHexPrint(byte[] bytes)
{
char[] hexChars = new char[bytes.length * 3];
for (int j = 0; j < bytes.length; j++)
{
int v = bytes[j] & 0xFF;
hexChars[j * 3] = HEX_ARRAY[v >>> 4];
hexChars[j * 3 + 1] = HEX_ARRAY[v & 0x0F];
hexChars[j * 3 + 2] = ' ';
}
return new String(hexChars);
}
/**
* 字节数组中字节替换
*
* @param originalBytes
* 源字节数组
* @param offset
* 替换的起始位置
* @param len
* 替换的长度
* @param replaceBytes
* 替换成的字节数组内容
* @return 经过替换后的字节数组
*/
public static byte[] bytesReplace(byte[] originalBytes, int offset, int len,
byte[] replaceBytes)
{
byte[] newBytes = new byte[originalBytes.length
+ (replaceBytes.length - len)];
System.arraycopy(originalBytes, 0, newBytes, 0, offset);
System.arraycopy(replaceBytes, 0, newBytes, offset,
replaceBytes.length);
System.arraycopy(originalBytes, offset + len, newBytes,
offset + replaceBytes.length,
originalBytes.length - offset - len);
return newBytes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy