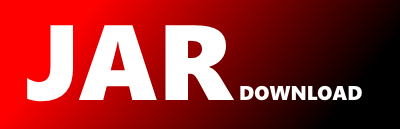
com.loocme.sys.util.FilePropUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import com.loocme.sys.util.ThreadUtil.ExecutorService;
import lombok.extern.slf4j.Slf4j;
import java.io.File;
import java.util.*;
/**
* @author liuchi
*/
@Slf4j
public class FilePropUtil
{
private static long LAST_UPDATE_TIME = 0;
private static Map PROP_MAP = new HashMap(16);
private static ExecutorService ESERVICE = null;
private static String FILE_PATH = "";
private static IWatchValue WATCH_VALUE_HANDLER = null;
public static String getFilePath()
{
return FILE_PATH;
}
public static void start(final String filePath)
{
ESERVICE = ThreadUtil.newSingleThreadExecutor();
FILE_PATH = filePath;
reload(filePath);
ESERVICE.execute(new Runnable()
{
@Override
public void run()
{
while (true)
{
try
{
Thread.sleep(10000);
}
catch (InterruptedException e)
{
log.error("", e);
}
reload(filePath);
}
}
});
}
public static void start(final String filePath, IWatchValue handler)
{
WATCH_VALUE_HANDLER = handler;
start(filePath);
}
public static Map loadAllProp(Properties props)
{
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy