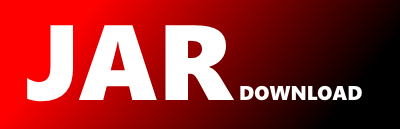
com.loocme.sys.util.FileUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import lombok.extern.slf4j.Slf4j;
import java.io.*;
import java.util.regex.Pattern;
/**
* 文件读写帮助类
*
* @author loocme
*/
@Slf4j
public class FileUtil
{
private static final int FILE_READ_BUFFER_SIZE = 100;
private static final String REG_FILEPATH_ISFILE = "[/\\\\][^/\\\\]+\\.[a-zA-Z0-9]+$";
/**
* 判断文件或文件夹是否存在
*
* @param path 文件路径
* @return 是否存在
*/
public static boolean isExist(String path)
{
return isExist(new File(path));
}
/**
* 判断文件或文件夹是否存在
*
* @param file 文件
* @return 是否存在
*/
public static boolean isExist(File file)
{
return file.exists();
}
public static boolean createFile(File file)
{
return createFile(file, false);
}
public static boolean createFile(File file, boolean force)
{
mkdirs(file);
try
{
if (file.exists())
{
if (file.isDirectory())
{
return false;
}
if (force)
{
file.delete();
file.createNewFile();
}
return true;
}
else
{
file.createNewFile();
return true;
}
}
catch (IOException e)
{
log.error("", e);
return false;
}
}
/**
* 创建文件所需的路径
*
* @param path 文件或文件夹路径
*/
public static void mkdirs(String path)
{
mkdirs(new File(path));
}
/**
* 创建文件所需的路径
*
* @param file 文件或文件夹
*/
public static void mkdirs(File file)
{
if (isExist(file))
{
return;
}
if (PatternUtil.isMatch(file.getAbsolutePath(), REG_FILEPATH_ISFILE, Pattern.CASE_INSENSITIVE))
{
File folder = file.getParentFile();
if (isExist(folder))
{
return;
}
folder.mkdirs();
}
else
{
file.mkdirs();
}
}
/**
* 判断路径是否为文件夹
*
* @param path 文件路径
* @return 是否为文件夹
*/
public static boolean isDirectory(String path)
{
return isDirectory(new File(path));
}
/**
* 判断文件是否为文件夹
*
* @param file 文件
* @return 是否为文件夹
*/
public static boolean isDirectory(File file)
{
return file.isDirectory();
}
/**
* 向文件中追加写入内容
*
* @param file 文件
* @param content 要追加的内容
*/
public static void write(File file, String content)
{
write(file, content, "UTF-8", false);
}
public static void write(File file, String content, boolean append)
{
write(file, content, "UTF-8", append);
}
public static void write(File file, String content, String charset)
{
write(file, content, charset, false);
}
/**
* 向文件中追加写入内容
*
* @param file 文件
* @param content 要追加的内容
*/
public static void write(File file, String content, String charset, boolean append)
{
mkdirs(file);
OutputStreamWriter osw = null;
try
{
osw = new OutputStreamWriter(new FileOutputStream(file, append), charset);
osw.write(content);
}
catch (IOException e)
{
log.error("", e);
}
finally
{
try
{
if (osw != null)
{
osw.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
}
public static void write(File file, byte[] by)
{
mkdirs(file);
FileOutputStream osw = null;
try
{
osw = new FileOutputStream(file);
osw.write(by);
}
catch (IOException e)
{
log.error("", e);
}
finally
{
try
{
if (osw != null)
{
osw.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
}
/**
* 向文件中追加写入内容
*
* @param file 文件
* @param content 要追加的内容
*/
public static void writeNewLine(File file, String content)
{
writeNewLine(file, content, "UTF-8", false);
}
public static void writeNewLine(File file, String content, boolean append)
{
writeNewLine(file, content, "UTF-8", append);
}
public static void writeNewLine(File file, String content, String charset)
{
writeNewLine(file, content, charset, false);
}
/**
* 向文件中追加写入内容
*
* @param file 文件
* @param content 要追加的内容
*/
public static void writeNewLine(File file, String content, String charset, boolean append)
{
mkdirs(file);
OutputStreamWriter osw = null;
try
{
osw = new OutputStreamWriter(new FileOutputStream(file, append), charset);
osw.write(System.getProperty("line.separator"));
osw.write(content);
}
catch (IOException e)
{
log.error("", e);
}
finally
{
try
{
if (osw != null)
{
osw.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
}
/**
* 向文件中追加写入内容
*
* @param file 文件
* @param is 需要写入的内容流
*/
public static void write(File file, InputStream is)
{
mkdirs(file);
FileOutputStream fos = null;
try
{
fos = new FileOutputStream(file);
byte[] bytes = new byte[1024];
int length = is.read(bytes);
while (length != -1)
{
fos.write(bytes, 0, length);
length = is.read(bytes);
}
}
catch (IOException e)
{
log.error("", e);
}
finally
{
try
{
if (fos != null)
{
fos.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
}
public static void writeObject(File file, Object object)
{
writeObject(file, object, false);
}
/**
* 向文件中追加写入内容
*
* @param file 文件
* @param object 要追加的内容
*/
public static void writeObject(File file, Object object, boolean append)
{
mkdirs(file);
ObjectOutputStream oos = null;
try
{
oos = new ObjectOutputStream(new FileOutputStream(file, append));
oos.writeObject(object);
oos.flush();
}
catch (IOException e)
{
log.error("", e);
}
finally
{
try
{
if (oos != null)
{
oos.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
}
@SuppressWarnings("unchecked")
public static T readObject(File file, Class clz)
{
Object obj = readObject(file);
if (null == obj)
{
return null;
}
if (clz.isAssignableFrom(obj.getClass()))
{
return (T) obj;
}
return null;
}
public static Object readObject(File file)
{
if (null == file || !file.isFile())
{
return null;
}
ObjectInputStream ois = null;
Object obj = null;
try
{
ois = new ObjectInputStream(new FileInputStream(file));
ois.readObject();
}
catch (IOException | ClassNotFoundException e)
{
log.error("", e);
} finally
{
try
{
if (ois != null)
{
ois.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
return obj;
}
/**
* 从文件中读取数据
*
* @param file 需要读取的文件
* @return 文件中的内容
*/
public static String read(File file)
{
if (!isExist(file) || isDirectory(file))
{
return null;
}
StringBuffer content = new StringBuffer();
String line;
BufferedReader br = null;
try
{
br = new BufferedReader(new InputStreamReader(new FileInputStream(file), "UTF-8"));
while ((line = br.readLine()) != null)
{
content.append(line);
}
}
catch (IOException e)
{
log.error("", e);
}
finally
{
try
{
if (br != null)
{
br.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
return content.toString();
}
/**
* 从文件中读取数据
*
* @param is 需要读取的文件
* @return 文件中的内容
*/
public static String read(InputStream is)
{
if (null == is)
{
return null;
}
StringBuffer content = new StringBuffer();
String line;
BufferedReader br = null;
try
{
br = new BufferedReader(new InputStreamReader(is, "UTF-8"));
while ((line = br.readLine()) != null)
{
content.append(line);
}
}
catch (IOException e)
{
log.error("", e);
}
finally
{
try
{
if (br != null)
{
br.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
return content.toString();
}
/**
* 从文件中读取数据
*
* @param file 需要读取的文件
* @return 文件中的内容
*/
public static byte[] readBytes(File file)
{
if (!isExist(file) || isDirectory(file))
{
return null;
}
InputStream inStream = null;
ByteArrayOutputStream swapStream = null;
try
{
inStream = new FileInputStream(file);
swapStream = new ByteArrayOutputStream();
byte[] buff = new byte[FILE_READ_BUFFER_SIZE];
int rc = 0;
while ((rc = inStream.read(buff, 0, FILE_READ_BUFFER_SIZE)) > 0)
{
swapStream.write(buff, 0, rc);
}
byte[] in2b = swapStream.toByteArray();
return in2b;
}
catch (IOException e)
{
log.error("", e);
}
finally
{
if (null != inStream)
{
try
{
inStream.close();
}
catch (IOException e)
{
log.error("", e);
}
}
if (null != swapStream)
{
try
{
swapStream.close();
}
catch (IOException e)
{
log.error("", e);
}
}
}
return null;
}
public static byte[] readBytes(InputStream inputStream)
{
if (null == inputStream)
{
return null;
}
ByteArrayOutputStream swapStream = null;
try
{
swapStream = new ByteArrayOutputStream();
byte[] buff = new byte[FILE_READ_BUFFER_SIZE];
int rc = 0;
while ((rc = inputStream.read(buff, 0, FILE_READ_BUFFER_SIZE)) > 0)
{
swapStream.write(buff, 0, rc);
}
byte[] in2b = swapStream.toByteArray();
return in2b;
}
catch (IOException e)
{
log.error("", e);
}
finally
{
if (null != swapStream)
{
try
{
swapStream.close();
}
catch (IOException e)
{
log.error("", e);
}
}
}
return null;
}
/**
* @author liuchi
*/
public interface IFileDealCall
{
/**
* 定义按行处理文件的具体实现
* @param line
* @param index
*/
public void dealLine(String line, long index);
}
public static void dealFile(File file, IFileDealCall call) throws Exception
{
dealFile(file, "UTF-8", call);
}
public static void dealFile(File file, String charset, IFileDealCall call) throws Exception
{
if (!isExist(file) || isDirectory(file))
{
return;
}
String line;
BufferedReader br = null;
try
{
br = new BufferedReader(new InputStreamReader(new FileInputStream(file), charset));
long count = 0;
while ((line = br.readLine()) != null)
{
count++;
call.dealLine(line, count);
}
}
catch (IOException e)
{
log.error("", e);
}
finally
{
try
{
if (br != null)
{
br.close();
}
}
catch (IOException e)
{
log.error("", e);
}
}
}
public static boolean appendNewLine(File destFile, File srcFile)
{
return append(destFile, srcFile, true);
}
public static boolean append(File destFile, File srcFile)
{
return append(destFile, srcFile, false);
}
private static boolean append(File destFile, File srcFile, boolean newLine)
{
FileInputStream fis = null;
FileOutputStream fos = null;
try
{
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile, true);
if (newLine)
{
fos.write(System.getProperty("line.separator").getBytes());
}
byte[] bytes = new byte[1024];
int length = fis.read(bytes);
while (length != -1)
{
fos.write(bytes, 0, length);
length = fis.read(bytes);
}
}
catch (IOException e)
{
log.error("", e);
}
finally
{
if (null != fis)
{
try
{
fis.close();
}
catch (IOException e)
{
log.error("", e);
}
}
if (null != fos)
{
try
{
fos.close();
}
catch (IOException e)
{
log.error("", e);
}
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy