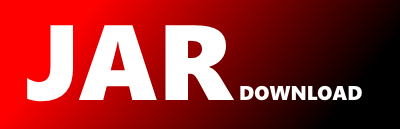
com.loocme.sys.util.HttpGetUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import java.util.List;
import java.util.Map;
import java.util.regex.Pattern;
import com.loocme.sys.constance.RegConst;
import com.loocme.sys.datastruct.Var;
import com.loocme.sys.exception.HttpConnectionException;
/**
* get请求使用帮助类
*
* 会根据请求的url判断使用http还是https
*
* @author loocme
*
*/
public class HttpGetUtil
{
private static final String REG_IS_HTTPS = "^https:.+$";
private static final String REG_IS_HTTP = "^http:.+$";
/**
* post请求数据
*
* @param url
* 请求地址
* @param content
* 请求参数
* @return 响应信息
* @throws HttpConnectionException
*/
public static String request(String url, String content)
throws HttpConnectionException
{
return request(url, null, content);
}
/**
* post请求数据
*
* @param url
* 请求地址
* @param headerMap
* 请求中需指定的头信息
* @param content
* 请求参数
* @return 响应信息
* @throws HttpConnectionException
*/
public static String request(String url, Map headerMap,
String content) throws HttpConnectionException
{
if (PatternUtil.isMatch(url, REG_IS_HTTPS, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.get(url, headerMap, content);
}
else if (PatternUtil
.isMatch(url, REG_IS_HTTP, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.get(url, headerMap, content);
}
else
{
throw new HttpConnectionException(1001, "不是http请求");
}
}
/**
* post请求数据
*
* @param url
* 请求地址
* @param encoding
* 字符编码
* @param headerMap
* 请求中需指定的头信息
* @return 响应信息
* @throws HttpConnectionException
*/
public static String request(String url, String encoding, int timeout,
Map headerMap, Map requestParams)
throws HttpConnectionException
{
if (PatternUtil.isMatch(url, REG_IS_HTTPS, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.get(url, encoding, timeout, headerMap, RequestUtil.getReqContent(requestParams));
}
else if (PatternUtil
.isMatch(url, REG_IS_HTTP, Pattern.CASE_INSENSITIVE))
{
return HttpUtil.get(url, encoding, timeout, headerMap, RequestUtil.getReqContent(requestParams));
}
else
{
throw new HttpConnectionException(1001, "不是http请求");
}
}
public static Var getQueryStringParams(String queryString)
{
Var ret = Var.newObject();
if (StringUtil.isNull(queryString))
{
return ret;
}
List matches = PatternUtil.getMatches(queryString, RegConst.URL_QUERY_STRING);
if (ListUtil.isNull(matches))
{
return ret;
}
for (int i = 0; i < matches.size(); i++)
{
String[] match = matches.get(i);
ret.set(match[2], match[3]);
}
return ret;
}
}