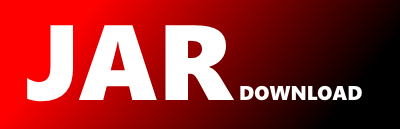
com.loocme.sys.util.ListUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import java.util.ArrayList;
import java.util.List;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
/**
* list集合帮助类
*
* @author loocme
*
*/
public class ListUtil
{
/**
* 判断list集合是否为空
*
* @param list
* 结合
* @return 是否为空
*/
public static boolean isNull(List> list)
{
if (null == list || list.size() == 0) {
return true;
}
return false;
}
/**
* 判断list集合是否非空
*
* @param list
* 集合
* @return 是否非空
*/
public static boolean isNotNull(List> list)
{
return !isNull(list);
}
/**
* list集合转换为array数组
*
* @param list
* list集合
* @return 转换得到的array数组
*/
public static Object[] toArray(List> list)
{
if (isNull(list)) {
return null;
}
return list.toArray();
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy