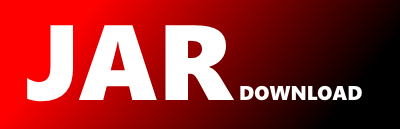
com.loocme.sys.util.MapUtil Maven / Gradle / Ivy
package com.loocme.sys.util;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
/**
* Map集合帮助类
*
* @author loocme
*
*/
public class MapUtil
{
/**
* 判断map集合是否为空
*
* @param map
* 集合
* @return 是否为空
*/
public static boolean isNull(Map, ?> map)
{
return null == map || map.isEmpty();
}
/**
* 判断map集合是否非空
*
* @param map
* 集合
* @return 是否非空
*/
public static boolean isNotNull(Map, ?> map)
{
return !isNull(map);
}
public static Map contactMap(Map map1, Map map2)
{
Map retMap = new HashMap(16);
if (null != map1)
{
retMap.putAll(map1);
}
if (null != map2)
{
retMap.putAll(map2);
}
return retMap;
}
public static Map fromJsonObject(JSONObject obj)
{
Map retMap = new HashMap(16);
if (null == obj)
{
return retMap;
}
Iterator it = obj.keySet().iterator();
while (it.hasNext())
{
String key = it.next();
Object tempVal = obj.get(key);
if (tempVal instanceof JSONObject)
{
retMap.put(key, fromJsonObject((JSONObject) tempVal));
}
else if (tempVal instanceof JSONArray)
{
retMap.put(key, ListUtil.fromJsonArray((JSONArray) tempVal));
}
else
{
retMap.put(key, tempVal);
}
}
return retMap;
}
public static String getString(Map map, String key)
{
if (MapUtil.isNull(map))
{
return "";
}
Object value = map.get(key);
if (null == value)
{
return "";
}
return value.toString();
}
public static Integer getInteger(Map map, String key)
{
return StringUtil.getInteger(getString(map, key));
}
public static Float getFloat(Map map, String key)
{
return StringUtil.getFloat(getString(map, key));
}
public static Double getDouble(Map map, String key)
{
return StringUtil.getDouble(getString(map, key));
}
public static Long getLong(Map map, String key)
{
return StringUtil.getLong(getString(map, key));
}
public static Boolean getBoolean(Map map, String key)
{
return StringUtil.getBoolean(getString(map, key));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy